Description
Class for a generic 3D finite element node, with x,y,z displacement.
This is the typical node that can be used for tetrahedrons, etc.
#include <ChNodeFEAxyz.h>

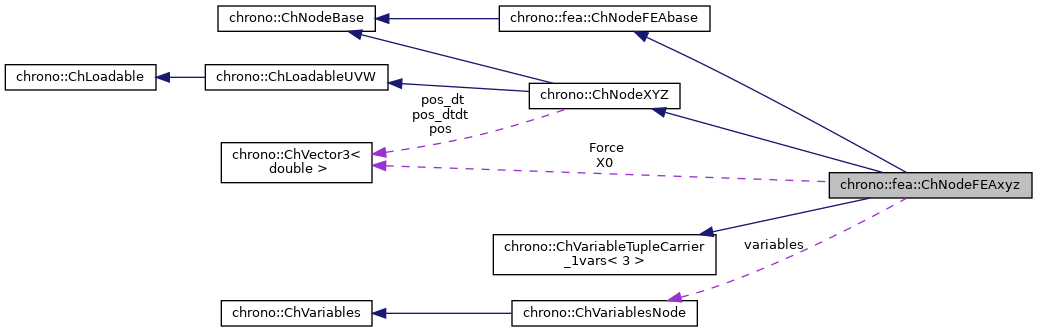
Public Member Functions | |
ChNodeFEAxyz (ChVector3d initial_pos=VNULL) | |
ChNodeFEAxyz (const ChNodeFEAxyz &other) | |
ChNodeFEAxyz & | operator= (const ChNodeFEAxyz &other) |
virtual ChVariablesNode & | Variables () override |
virtual void | Relax () override |
Set the rest position as the actual position. | |
virtual void | ForceToRest () override |
Reset to no speed and acceleration. | |
virtual void | SetFixed (bool fixed) override |
Fix/release this node. More... | |
virtual bool | IsFixed () const override |
Return true if the node is fixed (i.e., its state variables are not changed by the solver). | |
virtual double | GetMass () const override |
Get mass of the node. | |
virtual void | SetMass (double m) override |
Set mass of the node. | |
virtual void | SetX0 (const ChVector3d &x) |
Set the initial (reference) position. | |
virtual const ChVector3d & | GetX0 () const |
Get the initial (reference) position. | |
virtual void | SetForce (const ChVector3d &frc) |
Set the 3d applied force, in absolute reference. | |
virtual const ChVector3d & | GetForce () const |
Get the 3d applied force, in absolute reference. | |
virtual unsigned int | GetNumCoordsPosLevel () const override |
Get the number of degrees of freedom. | |
virtual void | ArchiveOut (ChArchiveOut &archive) override |
Method to allow serialization of transient data to archives. | |
virtual void | ArchiveIn (ChArchiveIn &archive) override |
Method to allow de-serialization of transient data from archives. | |
virtual ChVariables * | GetVariables1 () override |
virtual void | NodeIntStateGather (const unsigned int off_x, ChState &x, const unsigned int off_v, ChStateDelta &v, double &T) override |
virtual void | NodeIntStateScatter (const unsigned int off_x, const ChState &x, const unsigned int off_v, const ChStateDelta &v, const double T) override |
virtual void | NodeIntStateGatherAcceleration (const unsigned int off_a, ChStateDelta &a) override |
virtual void | NodeIntStateScatterAcceleration (const unsigned int off_a, const ChStateDelta &a) override |
virtual void | NodeIntStateIncrement (const unsigned int off_x, ChState &x_new, const ChState &x, const unsigned int off_v, const ChStateDelta &Dv) override |
virtual void | NodeIntStateGetIncrement (const unsigned int off_x, const ChState &x_new, const ChState &x, const unsigned int off_v, ChStateDelta &Dv) override |
virtual void | NodeIntLoadResidual_F (const unsigned int off, ChVectorDynamic<> &R, const double c) override |
virtual void | NodeIntLoadResidual_Mv (const unsigned int off, ChVectorDynamic<> &R, const ChVectorDynamic<> &w, const double c) override |
virtual void | NodeIntLoadLumpedMass_Md (const unsigned int off, ChVectorDynamic<> &Md, double &error, const double c) override |
virtual void | NodeIntToDescriptor (const unsigned int off_v, const ChStateDelta &v, const ChVectorDynamic<> &R) override |
virtual void | NodeIntFromDescriptor (const unsigned int off_v, ChStateDelta &v) override |
virtual void | InjectVariables (ChSystemDescriptor &descriptor) override |
Register with the given system descriptor any ChVariable objects associated with this item. | |
virtual void | VariablesFbReset () override |
Set the 'fb' part (the known term) of the encapsulated ChVariables to zero. | |
virtual void | VariablesFbLoadForces (double factor=1) override |
Add the current forces (applied to node) into the encapsulated ChVariables. More... | |
virtual void | VariablesQbLoadSpeed () override |
Initialize the 'qb' part of the ChVariables with the current value of speeds. | |
virtual void | VariablesQbSetSpeed (double step=0) override |
Fetch the item speed (ex. More... | |
virtual void | VariablesFbIncrementMq () override |
Add M*q (masses multiplied current 'qb') to Fb, ex. More... | |
virtual void | VariablesQbIncrementPosition (double step) override |
Increment node positions by the 'qb' part of the ChVariables, multiplied by a 'step' factor. More... | |
![]() | |
virtual void | SetIndex (unsigned int mindex) |
Sets the global index of the node. | |
virtual unsigned int | GetIndex () |
Gets the global index of the node. | |
virtual void | SetupInitial (ChSystem *system) |
Initial setup. | |
![]() | |
ChNodeBase (const ChNodeBase &other) | |
ChNodeBase & | operator= (const ChNodeBase &other) |
virtual unsigned int | GetNumCoordsVelLevel () const |
Get the number of degrees of freedom, derivative. More... | |
virtual unsigned int | GetNumCoordsPosLevelActive () const |
Get the actual number of active degrees of freedom. More... | |
virtual unsigned int | GetNumCoordsVelLevelActive () const |
Get the actual number of active degrees of freedom, derivative. More... | |
virtual bool | IsAllCoordsActive () const |
Return true if all node DOFs are active (no node variable is fixed). | |
unsigned int | NodeGetOffsetPosLevel () |
Get offset in the state vector (position part). | |
unsigned int | NodeGetOffsetVelLevel () |
Get offset in the state vector (speed part). | |
void | NodeSetOffsetPosLevel (const unsigned int moff) |
Set offset in the state vector (position part). | |
void | NodeSetOffsetVelLevel (const unsigned int moff) |
Set offset in the state vector (speed part). | |
![]() | |
ChNodeXYZ (const ChVector3d &initial_pos) | |
ChNodeXYZ (const ChNodeXYZ &other) | |
ChNodeXYZ & | operator= (const ChNodeXYZ &other) |
const ChVector3d & | GetPos () const |
void | SetPos (const ChVector3d &mpos) |
const ChVector3d & | GetPosDt () const |
void | SetPosDt (const ChVector3d &mposdt) |
const ChVector3d & | GetPosDt2 () const |
void | SetPosDt2 (const ChVector3d &mposdtdt) |
virtual unsigned int | GetLoadableNumCoordsPosLevel () override |
Gets the number of DOFs affected by this element (position part) | |
virtual unsigned int | GetLoadableNumCoordsVelLevel () override |
Gets the number of DOFs affected by this element (speed part) | |
virtual void | LoadableGetStateBlockPosLevel (int block_offset, ChState &mD) override |
Gets all the DOFs packed in a single vector (position part) | |
virtual void | LoadableGetStateBlockVelLevel (int block_offset, ChStateDelta &mD) override |
Gets all the DOFs packed in a single vector (speed part) | |
virtual void | LoadableStateIncrement (const unsigned int off_x, ChState &x_new, const ChState &x, const unsigned int off_v, const ChStateDelta &Dv) override |
Increment all DOFs using a delta. | |
virtual unsigned int | GetNumFieldCoords () override |
Number of coordinates in the interpolated field, ex=3 for a tetrahedron finite element or a cable, etc. More... | |
virtual unsigned int | GetNumSubBlocks () override |
Get the number of DOFs sub-blocks. | |
virtual unsigned int | GetSubBlockOffset (unsigned int nblock) override |
Get the offset of the specified sub-block of DOFs in global vector. | |
virtual unsigned int | GetSubBlockSize (unsigned int nblock) override |
Get the size of the specified sub-block of DOFs in global vector. | |
virtual bool | IsSubBlockActive (unsigned int nblock) const override |
Check if the specified sub-block of DOFs is active. | |
virtual void | LoadableGetVariables (std::vector< ChVariables * > &mvars) override |
Get the pointers to the contained ChVariables, appending to the mvars vector. | |
virtual void | ComputeNF (const double U, const double V, const double W, ChVectorDynamic<> &Qi, double &detJ, const ChVectorDynamic<> &F, ChVectorDynamic<> *state_x, ChVectorDynamic<> *state_w) override |
Evaluate Q=N'*F , for Q generalized lagrangian load, where N is some type of matrix evaluated at point P(U,V,W) assumed in absolute coordinates, and F is a load assumed in absolute coordinates. More... | |
virtual double | GetDensity () override |
This is not needed because not used in quadrature. | |
![]() | |
virtual bool | IsTetrahedronIntegrationNeeded () |
If true, use quadrature over u,v,w in [0..1] range as tetrahedron volumetric coords (with z=1-u-v-w) otherwise use default quadrature over u,v,w in [-1..+1] as box isoparametric coords. | |
virtual bool | IsTrianglePrismIntegrationNeeded () |
If true, use quadrature over u,v in [0..1] range as triangle natural coords (with z=1-u-v), and use linear quadrature over w in [-1..+1], otherwise use default quadrature over u,v,w in [-1..+1] as box isoparametric coords. | |
Protected Attributes | |
ChVariablesNode | variables |
3D node variables, with x,y,z | |
ChVector3d | X0 |
reference position | |
ChVector3d | Force |
applied force | |
![]() | |
unsigned int | g_index |
global node index | |
![]() | |
unsigned int | offset_x |
offset in vector of state (position part) | |
unsigned int | offset_w |
offset in vector of state (speed part) | |
Additional Inherited Members | |
![]() | |
typedef ChConstraintTuple_1vars< ChVariableTupleCarrier_1vars< N1 > > | type_constraint_tuple |
![]() | |
double | m_TotalMass |
Nodal mass obtained from element mass matrix. | |
![]() | |
ChVector3d | pos |
ChVector3d | pos_dt |
ChVector3d | pos_dtdt |
![]() | |
static const int | nvars1 |
Member Function Documentation
◆ SetFixed()
|
overridevirtual |
Fix/release this node.
If fixed, its state variables are not changed by the solver.
Implements chrono::fea::ChNodeFEAbase.
Reimplemented in chrono::fea::ChNodeFEAxyzDDD, chrono::fea::ChNodeFEAxyzDD, and chrono::fea::ChNodeFEAxyzD.
◆ VariablesFbIncrementMq()
|
overridevirtual |
Add M*q (masses multiplied current 'qb') to Fb, ex.
if qb is initialized with v_old using VariablesQbLoadSpeed, this method can be used in timestepping schemes that do: M*v_new = M*v_old + forces*dt
Reimplemented from chrono::ChNodeBase.
Reimplemented in chrono::fea::ChNodeFEAxyzDDD, chrono::fea::ChNodeFEAxyzDD, and chrono::fea::ChNodeFEAxyzD.
◆ VariablesFbLoadForces()
|
overridevirtual |
Add the current forces (applied to node) into the encapsulated ChVariables.
Include in the 'fb' part: qf+=forces*factor
Reimplemented from chrono::ChNodeBase.
Reimplemented in chrono::fea::ChNodeFEAxyzDDD, chrono::fea::ChNodeFEAxyzDD, and chrono::fea::ChNodeFEAxyzD.
◆ VariablesQbIncrementPosition()
|
overridevirtual |
Increment node positions by the 'qb' part of the ChVariables, multiplied by a 'step' factor.
pos+=qb*step If qb is a speed, this behaves like a single step of 1-st order numerical integration (Euler integration).
Reimplemented from chrono::ChNodeBase.
Reimplemented in chrono::fea::ChNodeFEAxyzDDD, chrono::fea::ChNodeFEAxyzDD, and chrono::fea::ChNodeFEAxyzD.
◆ VariablesQbSetSpeed()
|
overridevirtual |
Fetch the item speed (ex.
linear velocity, in xyz nodes) from the 'qb' part of the ChVariables and sets it as the current item speed. If 'step' is not 0, also should compute the approximate acceleration of the item using backward differences, that is accel=(new_speed-old_speed)/step. Mostly used after the solver provided the solution in ChVariables.
Reimplemented from chrono::ChNodeBase.
Reimplemented in chrono::fea::ChNodeFEAxyzDDD, chrono::fea::ChNodeFEAxyzDD, and chrono::fea::ChNodeFEAxyzD.
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono/fea/ChNodeFEAxyz.h
- /builds/uwsbel/chrono/src/chrono/fea/ChNodeFEAxyz.cpp