Description
Class which defines a contact surface for FEA elements, using a mesh of triangles.
Differently from ChContactSurfaceNodeCloud, this also captures the node-vs-face and edge-vs-edge cases, but has a higher computational overhead.
#include <ChContactSurfaceMesh.h>
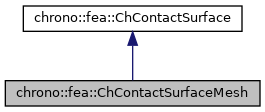
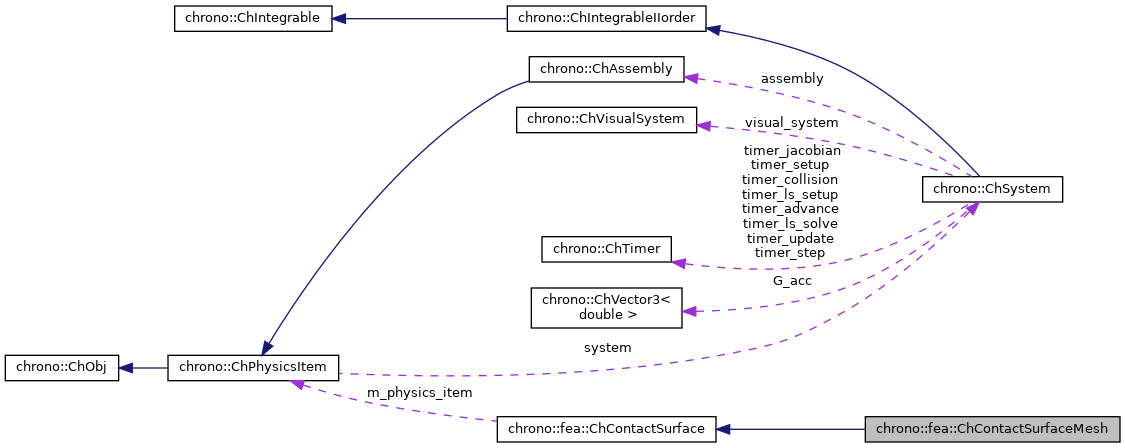
Public Member Functions | |
ChContactSurfaceMesh (std::shared_ptr< ChContactMaterial > material, ChMesh *mesh=nullptr) | |
void | AddFace (std::shared_ptr< ChNodeFEAxyz > node1, std::shared_ptr< ChNodeFEAxyz > node2, std::shared_ptr< ChNodeFEAxyz > node3, std::shared_ptr< ChNodeFEAxyz > edge_node1, std::shared_ptr< ChNodeFEAxyz > edge_node2, std::shared_ptr< ChNodeFEAxyz > edge_node3, bool owns_node1, bool owns_node2, bool owns_node3, bool owns_edge1, bool owns_edge2, bool owns_edge3, double sphere_swept=0.0) |
Add the face specified by the three specified XYZ nodes to this collision mesh. More... | |
void | AddFace (std::shared_ptr< ChNodeFEAxyzrot > node1, std::shared_ptr< ChNodeFEAxyzrot > node2, std::shared_ptr< ChNodeFEAxyzrot > node3, std::shared_ptr< ChNodeFEAxyzrot > edge_node1, std::shared_ptr< ChNodeFEAxyzrot > edge_node2, std::shared_ptr< ChNodeFEAxyzrot > edge_node3, bool owns_node1, bool owns_node2, bool owns_node3, bool owns_edge1, bool owns_edge2, bool owns_edge3, double sphere_swept=0.0) |
Add the face specified by the three given XYZROT nodes to this collision mesh. More... | |
void | AddFacesFromBoundary (const ChMesh &mesh, double sphere_swept=0.0, bool ccw=true, bool include_cable_elements=true, bool include_beam_elements=true) |
Utility function to add all boundary faces of the specified FEA mesh to this collision surface. More... | |
void | ConstructFromTrimesh (std::shared_ptr< ChTriangleMeshConnected > trimesh, double sphere_swept=0.0) |
Construct a contact surface from a triangular mesh. More... | |
virtual ChAABB | GetAABB () const override |
Get the current axis-aligned bounding box. | |
std::vector< std::shared_ptr< ChContactTriangleXYZ > > & | GetTrianglesXYZ () |
Get the list of triangles. | |
std::vector< std::shared_ptr< ChContactTriangleXYZRot > > & | GetTrianglesXYZRot () |
Get the list of triangles for nodes with rotational dofs. | |
unsigned int | GetNumTriangles () const |
Get the number of triangles. | |
unsigned int | GetNumVertices () const |
Get the number of vertices. | |
virtual void | SyncCollisionModels () const override |
virtual void | AddCollisionModelsToSystem (ChCollisionSystem *coll_sys) const override |
virtual void | RemoveCollisionModelsFromSystem (ChCollisionSystem *coll_sys) const override |
void | OutputSimpleMesh (std::vector< ChVector3d > &vert_pos, std::vector< ChVector3d > &vert_vel, std::vector< ChVector3i > &triangles, std::vector< ChVector3b > &owns_node, std::vector< ChVector3b > &owns_edge) const |
Utility function for exporting the contact mesh in a pointer-less manner. More... | |
![]() | |
ChContactSurface (std::shared_ptr< ChContactMaterial > material, ChPhysicsItem *mesh=nullptr) | |
ChPhysicsItem * | GetPhysicsItem () |
Get the owner physics item (e.g., an FEA mesh). | |
void | SetPhysicsItem (ChPhysicsItem *physics_item) |
Set the owner physics item (e.g., an FEA mesh). | |
void | DisableSelfCollisions (int family) |
Disable self-collisions (default: enabled). More... | |
std::shared_ptr< ChContactMaterial > & | GetMaterialSurface () |
Get the surface contact material. | |
Additional Inherited Members | |
![]() | |
std::shared_ptr< ChContactMaterial > | m_material |
contact material properties | |
ChPhysicsItem * | m_physics_item |
associated physics item (e.g., an FEA mesh) | |
bool | m_self_collide |
include self-collisions? | |
int | m_collision_family |
collision family (if no self-collisions) | |
Member Function Documentation
◆ AddFace() [1/2]
void chrono::fea::ChContactSurfaceMesh::AddFace | ( | std::shared_ptr< ChNodeFEAxyz > | node1, |
std::shared_ptr< ChNodeFEAxyz > | node2, | ||
std::shared_ptr< ChNodeFEAxyz > | node3, | ||
std::shared_ptr< ChNodeFEAxyz > | edge_node1, | ||
std::shared_ptr< ChNodeFEAxyz > | edge_node2, | ||
std::shared_ptr< ChNodeFEAxyz > | edge_node3, | ||
bool | owns_node1, | ||
bool | owns_node2, | ||
bool | owns_node3, | ||
bool | owns_edge1, | ||
bool | owns_edge2, | ||
bool | owns_edge3, | ||
double | sphere_swept = 0.0 |
||
) |
Add the face specified by the three specified XYZ nodes to this collision mesh.
- Parameters
-
node1 face node1 node2 face node2 node3 face node3 edge_node1 edge node 1 (nullptr if no wing node) edge_node2 edge node 2 (nullptr if no wing node) edge_node3 edge node 3 (nullptr if no wing node) owns_node1 this collision face owns node1 owns_node2 this collision face owns node2 owns_node3 this collision face owns node3 owns_edge1 this collision face owns edge1 owns_edge2 this collision face owns edge2 owns_edge3 this collision face owns edge3 sphere_swept thickness (radius of sweeping sphere)
◆ AddFace() [2/2]
void chrono::fea::ChContactSurfaceMesh::AddFace | ( | std::shared_ptr< ChNodeFEAxyzrot > | node1, |
std::shared_ptr< ChNodeFEAxyzrot > | node2, | ||
std::shared_ptr< ChNodeFEAxyzrot > | node3, | ||
std::shared_ptr< ChNodeFEAxyzrot > | edge_node1, | ||
std::shared_ptr< ChNodeFEAxyzrot > | edge_node2, | ||
std::shared_ptr< ChNodeFEAxyzrot > | edge_node3, | ||
bool | owns_node1, | ||
bool | owns_node2, | ||
bool | owns_node3, | ||
bool | owns_edge1, | ||
bool | owns_edge2, | ||
bool | owns_edge3, | ||
double | sphere_swept = 0.0 |
||
) |
Add the face specified by the three given XYZROT nodes to this collision mesh.
- Parameters
-
node1 face node1 node2 face node2 node3 face node3 edge_node1 edge node 1 (nullptr if no wing node) edge_node2 edge node 2 (nullptr if no wing node) edge_node3 edge node 3 (nullptr if no wing node) owns_node1 this collision face owns node1 owns_node2 this collision face owns node2 owns_node3 this collision face owns node3 owns_edge1 this collision face owns edge1 owns_edge2 this collision face owns edge2 owns_edge3 this collision face owns edge3 sphere_swept thickness (radius of sweeping sphere)
◆ AddFacesFromBoundary()
void chrono::fea::ChContactSurfaceMesh::AddFacesFromBoundary | ( | const ChMesh & | mesh, |
double | sphere_swept = 0.0 , |
||
bool | ccw = true , |
||
bool | include_cable_elements = true , |
||
bool | include_beam_elements = true |
||
) |
Utility function to add all boundary faces of the specified FEA mesh to this collision surface.
The function scans all the finite elements already added in the parent ChMesh and adds the faces that are not shared (ie. the faces on the boundary 'skin'). For shells, the argument 'ccw' indicates whether the face vertices are provided in a counter-clockwise (default) or clockwise order(because shells collisions are oriented and might work only from the "outer" side). Currently supported elements that generate boundary skin:
- solids:
- ChElementTetrahedron: all solid tetrahedrons
- ChElementHexahedron: all solid hexahedrons
- shells:
- ChElementShellANCF_3423 ANCF: shells (only one side)
- ChElementShellANCF_3443 ANCF: shells (only one side)
- ChElementShellANCF_3833 ANCF: shells (only one side)
- ChElementShellReissner: Reissner 4-nodes shells (only one side)
- beams:
- ChElementCableANCF: ANCF beams (as sphere-swept lines, i.e. sequence of capsules)
- ChElementBeamEuler: Euler-Bernoulli beams (as sphere-swept lines, i.e. sequence of capsules)
- Parameters
-
mesh FEA mesh sphere_swept radius of swept sphere ccw indicate counterclockwise vertex ordering include_cable_elements create contact triangles for cable elements include_beam_elements create contact triangles for beam elements
◆ ConstructFromTrimesh()
void chrono::fea::ChContactSurfaceMesh::ConstructFromTrimesh | ( | std::shared_ptr< ChTriangleMeshConnected > | trimesh, |
double | sphere_swept = 0.0 |
||
) |
Construct a contact surface from a triangular mesh.
FEA nodes are created at the mesh vertex locations.
◆ OutputSimpleMesh()
void chrono::fea::ChContactSurfaceMesh::OutputSimpleMesh | ( | std::vector< ChVector3d > & | vert_pos, |
std::vector< ChVector3d > & | vert_vel, | ||
std::vector< ChVector3i > & | triangles, | ||
std::vector< ChVector3b > & | owns_node, | ||
std::vector< ChVector3b > & | owns_edge | ||
) | const |
Utility function for exporting the contact mesh in a pointer-less manner.
The mesh is specified as a set of 3D vertex points (with associated velocities) and a set of faces (indices into the vertex array). In addition, ownership of nodes and edges among the consitutent triangles is returned in 'owns_node' and 'owns_edge'.
- Parameters
-
vert_pos mesh vertices (absolute xyz positions) vert_vel vertex velocities (absolute xyz velocities) triangles triangle faces (indices in vertex array) owns_node node ownership for each triangular face owns_edge edge ownership for each triangular face
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono/fea/ChContactSurfaceMesh.h
- /builds/uwsbel/chrono/src/chrono/fea/ChContactSurfaceMesh.cpp