Description
Deformable terrain model.
This class implements a deformable terrain based on the Soil Contact Model. Unlike RigidTerrain, the vertical coordinates of this terrain mesh can be deformed due to interaction with ground vehicles or other collision shapes.
#include <SCMDeformableTerrain.h>
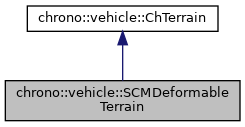
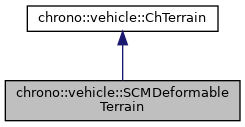
Classes | |
struct | NodeInfo |
Information at SCM node. More... | |
class | SoilParametersCallback |
Class to be used as a callback interface for location-dependent soil parameters. More... | |
Public Types | |
enum | DataPlotType { PLOT_NONE, PLOT_LEVEL, PLOT_LEVEL_INITIAL, PLOT_SINKAGE, PLOT_SINKAGE_ELASTIC, PLOT_SINKAGE_PLASTIC, PLOT_STEP_PLASTIC_FLOW, PLOT_PRESSURE, PLOT_PRESSURE_YELD, PLOT_SHEAR, PLOT_K_JANOSI, PLOT_IS_TOUCHED, PLOT_ISLAND_ID, PLOT_MASSREMAINDER } |
typedef std::pair< ChVector2< int >, double > | NodeLevel |
Node height level at a given grid location. | |
Public Member Functions | |
SCMDeformableTerrain (ChSystem *system, bool visualization_mesh=true) | |
Construct a default SCM deformable terrain. More... | |
void | SetPlane (const ChCoordsys<> &plane) |
Set the plane reference. More... | |
const ChCoordsys & | GetPlane () const |
Get the current reference plane. More... | |
void | SetSoilParameters (double Bekker_Kphi, double Bekker_Kc, double Bekker_n, double Mohr_cohesion, double Mohr_friction, double Janosi_shear, double elastic_K, double damping_R) |
Set the properties of the SCM soil model. More... | |
void | EnableBulldozing (bool mb) |
Enable/disable the creation of soil inflation at the side of the ruts (bulldozing effects). | |
void | SetBulldozingParameters (double erosion_angle, double flow_factor=1.0, int erosion_iterations=3, int erosion_propagations=10) |
Set parameters controlling the creation of side ruts (bulldozing effects). More... | |
void | SetTestHeight (double offset) |
Set the vertical level up to which collision is tested (relative to the reference level at the sample point). More... | |
double | GetTestHeight () const |
Return the current test height level. | |
void | SetPlotType (DataPlotType plot_type, double min_val, double max_val) |
Set the color plot type for the soil mesh. More... | |
void | SetColor (const ChColor &color) |
Set visualization color. | |
void | SetTexture (const std::string tex_file, float scale_x=1, float scale_y=1) |
Set texture properties. More... | |
void | AddMovingPatch (std::shared_ptr< ChBody > body, const ChVector<> &OOBB_center, const ChVector<> &OOBB_dims) |
Add a new moving patch. More... | |
void | RegisterSoilParametersCallback (std::shared_ptr< SoilParametersCallback > cb) |
Specify the callback object to set the soil parameters at given (x,y) locations. More... | |
double | GetInitHeight (const ChVector<> &loc) const |
Get the initial (undeformed) terrain height below the specified location. | |
ChVector | GetInitNormal (const ChVector<> &loc) const |
Get the initial (undeformed) terrain normal at the point below the specified location. | |
virtual double | GetHeight (const ChVector<> &loc) const override |
Get the terrain height below the specified location. | |
virtual ChVector | GetNormal (const ChVector<> &loc) const override |
Get the terrain normal at the point below the specified location. | |
virtual float | GetCoefficientFriction (const ChVector<> &loc) const override |
Get the terrain coefficient of friction at the point below the specified location. More... | |
NodeInfo | GetNodeInfo (const ChVector<> &loc) const |
Get SCM information at the node closest to the specified location. | |
std::shared_ptr< ChTriangleMeshShape > | GetMesh () const |
Get the visualization triangular mesh. | |
void | SetMeshWireframe (bool val) |
Set the visualization mesh as wireframe or as solid (default: wireframe). More... | |
void | WriteMesh (const std::string &filename) const |
Save the visualization mesh as a Wavefront OBJ file. | |
void | Initialize (double sizeX, double sizeY, double delta) |
Initialize the terrain system (flat). More... | |
void | Initialize (const std::string &heightmap_file, double sizeX, double sizeY, double hMin, double hMax, double delta) |
Initialize the terrain system (height map). More... | |
void | Initialize (const std::string &mesh_file, double delta) |
Initialize the terrain system (mesh). More... | |
std::vector< NodeLevel > | GetModifiedNodes (bool all_nodes=false) const |
Get the heights of all modified grid nodes. More... | |
void | SetModifiedNodes (const std::vector< NodeLevel > &nodes) |
Modify the level of grid nodes from the given list. | |
TerrainForce | GetContactForce (std::shared_ptr< ChBody > body) const |
Return the current cumulative contact force on the specified body (due to interaction with the SCM terrain). | |
int | GetNumRayCasts () const |
Return the number of rays cast at last step. | |
int | GetNumRayHits () const |
Return the number of ray hits at last step. | |
int | GetNumContactPatches () const |
Return the number of contact patches at last step. | |
int | GetNumErosionNodes () const |
Return the number of nodes in the erosion domain at last step (bulldosing effects). | |
double | GetTimerMovingPatches () const |
Return time for updating moving patches at last step (ms). | |
double | GetTimerRayTesting () const |
Return time for geometric ray intersection tests at last step (ms). | |
double | GetTimerRayCasting () const |
Return time for ray casting at last step (ms). Includes time for ray intersectin testing. | |
double | GetTimerContactPatches () const |
Return time for computing contact patches at last step (ms). | |
double | GetTimerContactForces () const |
Return time for computing contact forces at last step (ms). | |
double | GetTimerBulldozing () const |
Return time for computing bulldozing effects at last step (ms). | |
double | GetTimerVisUpdate () const |
Return time for visualization assets update at last step (ms). | |
void | PrintStepStatistics (std::ostream &os) const |
Print timing and counter information for last step. | |
std::shared_ptr< SCMDeformableSoil > | GetGroundObject () const |
![]() | |
virtual void | Synchronize (double time) |
Update the state of the terrain system at the specified time. | |
virtual void | Advance (double step) |
Advance the state of the terrain system by the specified duration. | |
virtual void | GetProperties (const ChVector<> &loc, double &height, ChVector<> &normal, float &friction) const |
Get all terrain characteristics at the point below the specified location. | |
void | RegisterHeightFunctor (std::shared_ptr< HeightFunctor > functor) |
Specify the functor object to provide the terrain height at given locations. More... | |
void | RegisterNormalFunctor (std::shared_ptr< NormalFunctor > functor) |
Specify the functor object to provide the terrain normal at given locations. More... | |
void | RegisterFrictionFunctor (std::shared_ptr< FrictionFunctor > functor) |
Specify the functor object to provide the coefficient of friction at given locations. | |
Additional Inherited Members | |
![]() | |
std::shared_ptr< HeightFunctor > | m_height_fun |
functor for location-dependent terrain height | |
std::shared_ptr< NormalFunctor > | m_normal_fun |
functor for location-dependent terrain normal | |
std::shared_ptr< FrictionFunctor > | m_friction_fun |
functor for location-dependent coefficient of friction | |
Constructor & Destructor Documentation
◆ SCMDeformableTerrain()
chrono::vehicle::SCMDeformableTerrain::SCMDeformableTerrain | ( | ChSystem * | system, |
bool | visualization_mesh = true |
||
) |
Construct a default SCM deformable terrain.
The user is responsible for calling various Set methods before Initialize.
- Parameters
-
[in] system pointer to the containing multibody system [in] visualization_mesh enable/disable visualization asset
Member Function Documentation
◆ AddMovingPatch()
void chrono::vehicle::SCMDeformableTerrain::AddMovingPatch | ( | std::shared_ptr< ChBody > | body, |
const ChVector<> & | OOBB_center, | ||
const ChVector<> & | OOBB_dims | ||
) |
Add a new moving patch.
Multiple calls to this function can be made, each of them adding a new active patch area. If no patches are defined, ray-casting is performed for every single node of the underlying SCM grid. If at least one patch is defined, ray-casting is performed only for mesh nodes within the AABB of the body OOBB projection onto the SCM plane.
- Parameters
-
[in] body monitored body [in] OOBB_center OOBB center, relative to body [in] OOBB_dims OOBB dimensions
◆ GetCoefficientFriction()
|
overridevirtual |
Get the terrain coefficient of friction at the point below the specified location.
This coefficient of friction value may be used by certain tire models to modify the tire characteristics, but it will have no effect on the interaction of the terrain with other objects (including tire models that do not explicitly use it). For SCMDeformableTerrain, this function defers to the user-provided functor object of type ChTerrain::FrictionFunctor, if one was specified. Otherwise, it returns the constant value of 0.8.
Reimplemented from chrono::vehicle::ChTerrain.
◆ GetModifiedNodes()
std::vector< SCMDeformableTerrain::NodeLevel > chrono::vehicle::SCMDeformableTerrain::GetModifiedNodes | ( | bool | all_nodes = false | ) | const |
Get the heights of all modified grid nodes.
If 'all_nodes = true', return modified nodes from the start of simulation. Otherwise, return only the nodes modified over the last step.
◆ GetPlane()
const ChCoordsys & chrono::vehicle::SCMDeformableTerrain::GetPlane | ( | ) | const |
Get the current reference plane.
The SCM terrain patch is in the (x,y) plane with normal along the Z axis.
◆ Initialize() [1/3]
void chrono::vehicle::SCMDeformableTerrain::Initialize | ( | const std::string & | heightmap_file, |
double | sizeX, | ||
double | sizeY, | ||
double | hMin, | ||
double | hMax, | ||
double | delta | ||
) |
Initialize the terrain system (height map).
The initial undeformed terrain profile is provided via the specified image file as a height map. The terrain patch is scaled in the horizontal plane of the SCM frame to sizeX x sizeY, while the initial height is scaled between hMin and hMax (with the former corresponding to a pure balck pixel and the latter to a pure white pixel). The SCM grid resolution is specified through 'delta' and initial heights at grid points are obtained through interpolation (outside the terrain patch, the SCM node height is initialized to the height of the closest image pixel). For visualization purposes, a triangular mesh is also generated from the provided image file.
- Parameters
-
[in] heightmap_file filename for the height map (image file) [in] sizeX terrain dimension in the X direction [in] sizeY terrain dimension in the Y direction [in] hMin minimum height (black level) [in] hMax maximum height (white level) [in] delta grid spacing (may be slightly decreased)
◆ Initialize() [2/3]
void chrono::vehicle::SCMDeformableTerrain::Initialize | ( | const std::string & | mesh_file, |
double | delta | ||
) |
Initialize the terrain system (mesh).
The initial undeformed terrain profile is provided via the specified Wavefront OBJ mesh file. The dimensions of the terrain patch in the horizontal plane of the SCM frame is set to the range of the x and y mesh vertex coordinates, respectively. The SCM grid resolution is specified through 'delta' and initial heights at grid points are obtained through linear interpolation (outside the mesh footprint, the height of a grid node is set to the height of the closest point on the mesh). A visualization mesh is created from the original mesh resampled at the grid node points.
- Parameters
-
[in] mesh_file filename for the height map (image file) [in] delta grid spacing (may be slightly decreased)
◆ Initialize() [3/3]
void chrono::vehicle::SCMDeformableTerrain::Initialize | ( | double | sizeX, |
double | sizeY, | ||
double | delta | ||
) |
Initialize the terrain system (flat).
This version creates a flat array of points.
- Parameters
-
[in] sizeX terrain dimension in the X direction [in] sizeY terrain dimension in the Y direction [in] delta grid spacing (may be slightly decreased)
◆ RegisterSoilParametersCallback()
void chrono::vehicle::SCMDeformableTerrain::RegisterSoilParametersCallback | ( | std::shared_ptr< SoilParametersCallback > | cb | ) |
Specify the callback object to set the soil parameters at given (x,y) locations.
To use constant soil parameters throughout the entire patch, use SetSoilParameters.
◆ SetBulldozingParameters()
void chrono::vehicle::SCMDeformableTerrain::SetBulldozingParameters | ( | double | erosion_angle, |
double | flow_factor = 1.0 , |
||
int | erosion_iterations = 3 , |
||
int | erosion_propagations = 10 |
||
) |
Set parameters controlling the creation of side ruts (bulldozing effects).
- Parameters
-
erosion_angle angle of erosion of the displaced material [degrees] flow_factor growth of lateral volume relative to pressed volume erosion_iterations number of erosion refinements per timestep erosion_propagations number of concentric vertex selections subject to erosion
◆ SetMeshWireframe()
void chrono::vehicle::SCMDeformableTerrain::SetMeshWireframe | ( | bool | val | ) |
Set the visualization mesh as wireframe or as solid (default: wireframe).
Note: in wireframe mode, normals for the visualization mesh are not calculated.
◆ SetPlane()
void chrono::vehicle::SCMDeformableTerrain::SetPlane | ( | const ChCoordsys<> & | plane | ) |
Set the plane reference.
By default, the reference plane is horizontal with Z up (ISO vehicle reference frame). To set as Y up, call SetPlane(ChCoordys(VNULL, Q_from_AngX(-CH_C_PI_2)));
◆ SetPlotType()
void chrono::vehicle::SCMDeformableTerrain::SetPlotType | ( | DataPlotType | plot_type, |
double | min_val, | ||
double | max_val | ||
) |
Set the color plot type for the soil mesh.
When a scalar plot is used, also define the range in the pseudo-color colormap.
◆ SetSoilParameters()
void chrono::vehicle::SCMDeformableTerrain::SetSoilParameters | ( | double | Bekker_Kphi, |
double | Bekker_Kc, | ||
double | Bekker_n, | ||
double | Mohr_cohesion, | ||
double | Mohr_friction, | ||
double | Janosi_shear, | ||
double | elastic_K, | ||
double | damping_R | ||
) |
Set the properties of the SCM soil model.
These parameters are described in: "Parameter Identification of a Planetary Rover Wheel-Soil Contact Model via a Bayesian Approach", A.Gallina, R. Krenn et al. Note that the original SCM model does not include the K and R coefficients. A very large value of K and R=0 reproduce the original SCM.
- Parameters
-
Bekker_Kphi Kphi, frictional modulus in Bekker model Bekker_Kc Kc, cohesive modulus in Bekker model Bekker_n n, exponent of sinkage in Bekker model (usually 0.6...1.8) Mohr_cohesion Cohesion for shear failure [Pa] Mohr_friction Friction angle for shear failure [degree] Janosi_shear Shear parameter in Janosi-Hanamoto formula [m] elastic_K elastic stiffness K per unit area, [Pa/m] (must be larger than Kphi) damping_R vertical damping R per unit area [Pa.s/m] (proportional to vertical speed)
◆ SetTestHeight()
void chrono::vehicle::SCMDeformableTerrain::SetTestHeight | ( | double | offset | ) |
Set the vertical level up to which collision is tested (relative to the reference level at the sample point).
Since the contact is unilateral, this could be zero. However, when computing bulldozing flow, one might also need to know if in the surrounding there is some potential future contact: so it might be better to use a positive value (but not higher than the max. expected height of the bulldozed rubble, to avoid slowdown of collision tests). Default: 0.1 m.
◆ SetTexture()
void chrono::vehicle::SCMDeformableTerrain::SetTexture | ( | const std::string | tex_file, |
float | scale_x = 1 , |
||
float | scale_y = 1 |
||
) |
Set texture properties.
- Parameters
-
[in] tex_file texture filename [in] scale_x texture X scale [in] scale_y texture Y scale
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono_vehicle/terrain/SCMDeformableTerrain.h
- /builds/uwsbel/chrono/src/chrono_vehicle/terrain/SCMDeformableTerrain.cpp