chrono::vehicle::ChChassisRear Class Referenceabstract
Description
Base class for a rear chassis vehicle subsystem.
A rear chassis is attached (through one of the provided ChassisConnector templates) to another chassis. As such, a rear chassis is initialized with a position relative to the other chassis.
#include <ChChassis.h>
Inheritance diagram for chrono::vehicle::ChChassisRear:

Collaboration diagram for chrono::vehicle::ChChassisRear:
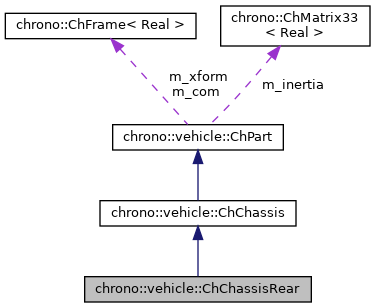
Public Member Functions | |
ChChassisRear (const std::string &name) | |
Construct a rear chassis subsystem with the specified name. | |
virtual const ChVector3d & | GetLocalPosFrontConnector () const =0 |
Get the location (in the local frame of this chassis) of the connection to the front chassis. | |
virtual void | Initialize (std::shared_ptr< ChChassis > chassis, int collision_family=0) |
Initialize the rear chassis relative to the specified front chassis. More... | |
![]() | |
ChChassis (const std::string &name, bool fixed=false) | |
Construct a chassis subsystem with the specified name. More... | |
virtual const ChVector3d | GetLocalPosRearConnector () const |
Get the location (in the local frame of this chassis) of the connection to a rear chassis. | |
std::shared_ptr< ChBodyAuxRef > | GetBody () const |
Get a handle to the vehicle's chassis body. | |
ChSystem * | GetSystem () const |
Get a pointer to the containing system. | |
const ChVector3d & | GetPos () const |
Get the global location of the chassis reference frame origin. | |
ChQuaternion | GetRot () const |
Get the orientation of the chassis reference frame. More... | |
ChVector3d | GetDriverPos () const |
Get the global location of the driver. | |
double | GetSpeed () const |
Get the vehicle speed. More... | |
double | GetCOMSpeed () const |
Get the speed of the chassis COM. More... | |
double | GetRollRate () const |
Get the roll rate of the chassis. More... | |
double | GetPitchRate () const |
Get the pitch rate of the chassis. More... | |
double | GetYawRate () const |
Get the yaw rate of the chassis. More... | |
double | GetTurnRate () const |
Get the turn rate of the chassis. More... | |
ChVector3d | GetPointLocation (const ChVector3d &locpos) const |
Get the global position of the specified point. More... | |
ChVector3d | GetPointVelocity (const ChVector3d &locpos) const |
Get the global velocity of the specified point. More... | |
ChVector3d | GetPointAcceleration (const ChVector3d &locpos) const |
Get the acceleration at the specified point. More... | |
virtual void | EnableCollision (bool state)=0 |
Enable/disable contact for the chassis. More... | |
void | SetFixed (bool val) |
Set the "fixed to ground" status of the chassis body. | |
bool | IsFixed () const |
Return true if the chassis body is fixed to ground. | |
bool | HasBushings () const |
Return true if the vehicle model contains bushings. | |
void | AddMarker (const std::string &name, const ChFrame<> &frame) |
Add a marker on the chassis body at the specified position (relative to the chassis reference frame). More... | |
const std::vector< std::shared_ptr< ChMarker > > & | GetMarkers () const |
void | SetAerodynamicDrag (double Cd, double area, double air_density) |
Set parameters and enable aerodynamic drag force calculation. More... | |
virtual void | Synchronize (double time) |
Update the state of the chassis subsystem. More... | |
void | AddJoint (std::shared_ptr< ChVehicleJoint > joint) |
Utility function to add a joint (kinematic or bushing) to the vehicle system. | |
void | AddExternalForceTorque (std::shared_ptr< ExternalForceTorque > load) |
Utility force to add an external load to the chassis body. | |
void | AddTerrainLoad (std::shared_ptr< ChLoadBase > terrain_load) |
Add a terrain load to the vehicle system. More... | |
virtual void | InitializeInertiaProperties () override |
Initialize subsystem inertia properties. More... | |
virtual void | UpdateInertiaProperties () override |
Update subsystem inertia properties. More... | |
![]() | |
const std::string & | GetName () const |
Get the name identifier for this subsystem. | |
void | SetName (const std::string &name) |
Set the name identifier for this subsystem. | |
virtual std::string | GetTemplateName () const =0 |
Get the name of the vehicle subsystem template. | |
bool | IsInitialized () const |
Return flag indicating whether or not the part is fully constructed. | |
double | GetMass () const |
Get the subsystem mass. More... | |
const ChFrame & | GetCOMFrame () const |
Get the current subsystem COM frame (relative to and expressed in the subsystem's reference frame). More... | |
const ChMatrix33 & | GetInertia () const |
Get the current subsystem inertia (relative to the subsystem COM frame). More... | |
const ChFrame & | GetTransform () const |
Get the current subsystem position relative to the global frame. More... | |
void | SetVisualizationType (VisualizationType vis) |
Set the visualization mode for this subsystem. | |
virtual void | AddVisualizationAssets (VisualizationType vis) |
Add visualization assets to this subsystem, for the specified visualization mode. | |
virtual void | RemoveVisualizationAssets () |
Remove all visualization assets from this subsystem. | |
virtual void | SetOutput (bool state) |
Enable/disable output for this subsystem. | |
bool | OutputEnabled () const |
Return the output state for this subsystem. | |
virtual void | ExportComponentList (rapidjson::Document &jsonDocument) const |
Export this subsystem's component list to the specified JSON object. More... | |
virtual void | Output (ChVehicleOutput &database) const |
Output data for this subsystem's component list to the specified database. | |
Additional Inherited Members | |
![]() | |
static void | RemoveJoint (std::shared_ptr< ChVehicleJoint > joint) |
Utility function to remove a joint (kinematic or bushing) from the vehicle system. | |
![]() | |
static ChMatrix33 | TransformInertiaMatrix (const ChVector3d &moments, const ChVector3d &products, const ChMatrix33<> &vehicle_rot, const ChMatrix33<> &body_rot) |
Utility function for transforming inertia tensors between centroidal frames. More... | |
![]() | |
virtual double | GetBodyMass () const =0 |
virtual ChFrame | GetBodyCOMFrame () const =0 |
virtual ChMatrix33 | GetBodyInertia () const =0 |
![]() | |
ChPart (const std::string &name) | |
Construct a vehicle subsystem with the specified name. | |
void | AddMass (double &mass) |
Add this subsystem's mass. More... | |
void | AddInertiaProperties (ChVector3d &com, ChMatrix33<> &inertia) |
Add this subsystem's inertia properties. More... | |
virtual void | Create (const rapidjson::Document &d) |
Create a vehicle subsystem from JSON data. More... | |
void | ExportBodyList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChBody >> bodies) const |
Export the list of bodies to the specified JSON document. | |
void | ExportShaftList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChShaft >> shafts) const |
Export the list of shafts to the specified JSON document. | |
void | ExportJointList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChLink >> joints) const |
Export the list of joints to the specified JSON document. | |
void | ExportCouplesList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChShaftsCouple >> couples) const |
Export the list of shaft couples to the specified JSON document. | |
void | ExportMarkerList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChMarker >> markers) const |
Export the list of markers to the specified JSON document. | |
void | ExportLinSpringList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChLinkTSDA >> springs) const |
Export the list of translational springs to the specified JSON document. | |
void | ExportRotSpringList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChLinkRSDA >> springs) const |
Export the list of rotational springs to the specified JSON document. | |
void | ExportBodyLoadList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChLoadBodyBody >> loads) const |
Export the list of body-body loads to the specified JSON document. | |
![]() | |
static void | RemoveVisualizationAssets (std::shared_ptr< ChPhysicsItem > item) |
Erase all visual shapes from the visual model associated with the specified physics item (if any). | |
static void | RemoveVisualizationAsset (std::shared_ptr< ChPhysicsItem > item, std::shared_ptr< ChVisualShape > shape) |
Erase the given shape from the visual model associated with the specified physics item (if any). | |
![]() | |
std::shared_ptr< ChBodyAuxRef > | m_body |
handle to the chassis body | |
std::shared_ptr< ChLoadContainer > | m_container_bushings |
load container for vehicle bushings | |
std::shared_ptr< ChLoadContainer > | m_container_external |
load container for external forces | |
std::shared_ptr< ChLoadContainer > | m_container_terrain |
load container for terrain forces | |
std::vector< std::shared_ptr< ExternalForceTorque > > | m_external_loads |
external loads | |
std::vector< std::shared_ptr< ChMarker > > | m_markers |
list of user-defined markers | |
bool | m_fixed |
is the chassis body fixed to ground? | |
![]() | |
std::string | m_name |
subsystem name | |
bool | m_initialized |
specifies whether ot not the part is fully constructed | |
bool | m_output |
specifies whether or not output is generated for this subsystem | |
std::shared_ptr< ChPart > | m_parent |
parent subsystem (empty if parent is vehicle) | |
double | m_mass |
subsystem mass | |
ChMatrix33 | m_inertia |
inertia tensor (relative to subsystem COM) | |
ChFrame | m_com |
COM frame (relative to subsystem reference frame) | |
ChFrame | m_xform |
subsystem frame expressed in the global frame | |
Member Function Documentation
◆ Initialize()
|
virtual |
Initialize the rear chassis relative to the specified front chassis.
The orientation is set to be the same as that of the front chassis while the location is based on the connector position on the front and rear chassis.
- Parameters
-
[in] chassis front chassis [in] collision_family chassis collision family
Reimplemented in chrono::vehicle::ChRigidChassisRear.
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono_vehicle/ChChassis.h
- /builds/uwsbel/chrono/src/chrono_vehicle/ChChassis.cpp