Description
Class for a FEA mesh visualization.
It converts tetrahedrons, etc. into a colored triangle mesh asset of class ChTriangleMeshShape that is contained in its sublevel, so that it can be rendered or postprocessed.
#include <ChVisualizationFEAmesh.h>
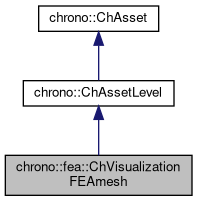
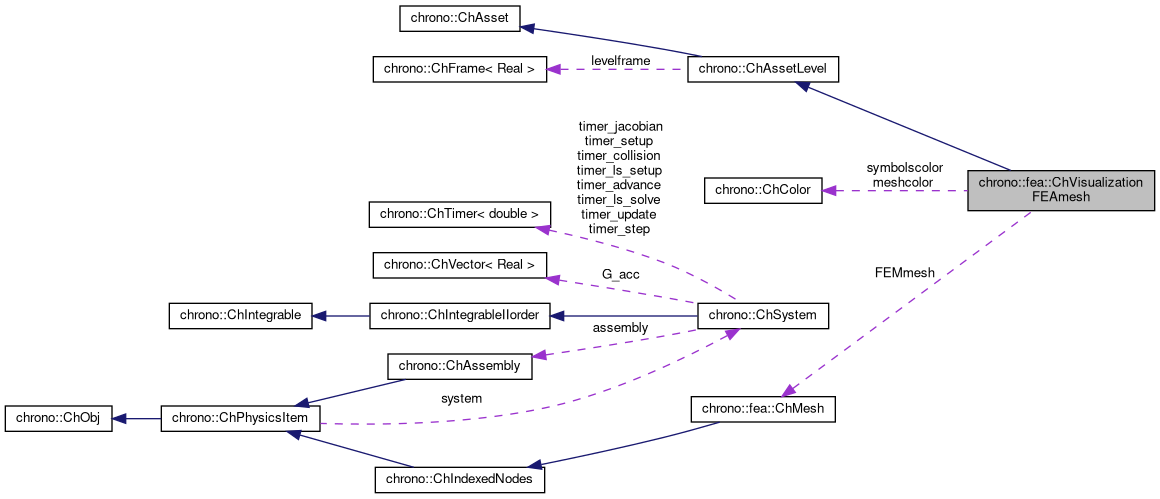
Public Member Functions | |
ChVisualizationFEAmesh (ChMesh &mymesh) | |
virtual ChMesh & | GetMesh () |
eChFemDataType | GetFEMdataType () |
void | SetFEMdataType (eChFemDataType mdata) |
eChFemGlyphs | GetFEMglyphType () |
void | SetFEMglyphType (eChFemGlyphs mdata) |
void | SetColorscaleMinMax (double mmin, double mmax) |
void | SetSymbolsScale (double mscale) |
double | GetSymbolsScale () |
void | SetSymbolsThickness (double mthick) |
double | GetSymbolsThickness () |
void | SetBeamResolution (int mres) |
Set the resolution of beam triangulated drawing, along direction of beam. | |
int | GetBeamResolution () |
void | SetBeamResolutionSection (int mres) |
Set the resolution of beam triangulated drawing, along the section (i.e. More... | |
int | GetBeamResolutionSection () |
void | SetShellResolution (int mres) |
Set the resolution of shell triangulated drawing. | |
int | GetShellResolution () |
void | SetShrinkElements (bool mshrink, double mfact) |
void | SetWireframe (bool mwireframe) |
void | SetBackfaceCull (bool mbc) |
void | SetZbufferHide (bool mhide) |
void | SetDefaultMeshColor (ChColor mcolor) |
void | SetDefaultSymbolsColor (ChColor mcolor) |
void | SetSmoothFaces (bool msmooth) |
void | SetDrawInUndeformedReference (bool mdu) |
virtual void | Update (ChPhysicsItem *updater, const ChCoordsys<> &coords) |
Updates all children assets, if any. More... | |
![]() | |
ChFrame & | GetFrame () |
Access the coordinate system information of the level, for setting/getting its position and rotation respect to its parent. | |
std::vector< std::shared_ptr< ChAsset > > & | GetAssets () |
Access to the list of children assets. | |
std::shared_ptr< ChAsset > | GetAssetN (unsigned int num) |
Get the Nth asset in list. | |
void | AddAsset (std::shared_ptr< ChAsset > masset) |
Add an asset to this level. | |
virtual void | ArchiveOUT (ChArchiveOut &marchive) override |
Method to allow serialization of transient data to archives. | |
virtual void | ArchiveIN (ChArchiveIn &marchive) override |
Method to allow de-serialization of transient data from archives. | |
Protected Attributes | |
ChMesh * | FEMmesh |
eChFemDataType | fem_data_type |
eChFemGlyphs | fem_glyph |
double | colorscale_min |
double | colorscale_max |
double | symbols_scale |
double | symbols_thickness |
bool | shrink_elements |
double | shrink_factor |
bool | wireframe |
bool | backface_cull |
bool | zbuffer_hide |
bool | smooth_faces |
bool | undeformed_reference |
int | beam_resolution |
int | beam_resolution_section |
int | shell_resolution |
ChColor | meshcolor |
ChColor | symbolscolor |
std::vector< int > | normal_accumulators |
![]() | |
ChFrame | levelframe |
std::vector< std::shared_ptr< ChAsset > > | assets |
Member Function Documentation
◆ SetBeamResolutionSection()
|
inline |
Set the resolution of beam triangulated drawing, along the section (i.e.
for circular section= number of points along the circle)
◆ Update()
|
virtual |
Updates all children assets, if any.
Note that when calling Update() on children assets, their 'coords' will be the result of concatenating this frame csys and 'coords'.
Reimplemented from chrono::ChAssetLevel.
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono/fea/ChVisualizationFEAmesh.h
- /builds/uwsbel/chrono/src/chrono/fea/ChVisualizationFEAmesh.cpp