Description
Base class for generic collision engine.
#include <ChCollisionSystem.h>

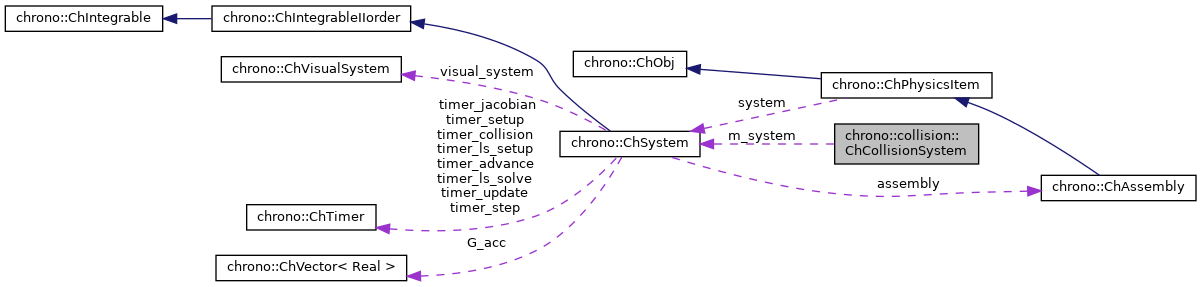
Classes | |
class | BroadphaseCallback |
Class to be used as a callback interface for user-defined actions to be performed for each 'near enough' pair of collision shapes found by the broad-phase collision step. More... | |
struct | ChRayhitResult |
Recover results from RayHit() raycasting. More... | |
class | NarrowphaseCallback |
Class to be used as a callback interface for user-defined actions to be performed at each collision pair found during the narrow-phase collision step. More... | |
class | VisualizationCallback |
Class to be used as a callback interface for user-defined visualization of collision shapes. More... | |
Public Types | |
enum | VisualizationModes { VIS_None = 0, VIS_Shapes = 1 << 0, VIS_Aabb = 1 << 1, VIS_Contacts = 1 << 2, VIS_MAX_MODES } |
Enumeration of supported flags for collision debug visualization. More... | |
Public Member Functions | |
virtual ChCollisionSystemType | GetType () const =0 |
Return the type of this collision system. | |
virtual void | Clear (void)=0 |
Clears all data instanced by this algorithm if any (like persistent contact manifolds) | |
virtual void | Add (ChCollisionModel *model)=0 |
Adds a collision model to the collision engine (custom data may be allocated). | |
virtual void | Remove (ChCollisionModel *model)=0 |
Removes a collision model from the collision engine (custom data may be deallocated). | |
virtual void | PreProcess () |
Removes all collision models from the collision engine (custom data may be deallocated). More... | |
virtual void | Run ()=0 |
Run the collision detection and finds the contacts. More... | |
virtual void | PostProcess () |
Optional synchronization operations, invoked after running the collision detection. | |
virtual void | GetBoundingBox (ChVector<> &aabb_min, ChVector<> &aabb_max) const =0 |
Return an AABB bounding all collision shapes in the system. | |
virtual double | GetTimerCollisionBroad () const =0 |
Return the time (in seconds) for broadphase collision detection. | |
virtual double | GetTimerCollisionNarrow () const =0 |
Return the time (in seconds) for narrowphase collision detection. | |
virtual void | ResetTimers () |
Reset any timers associated with collision detection. | |
virtual void | SetNumThreads (int nthreads) |
Set the number of OpenMP threads for collision detection. More... | |
virtual void | ReportContacts (ChContactContainer *mcontactcontainer)=0 |
After the Run() has completed, you can call this function to fill a 'contact container', that is an object inherited from class ChContactContainer. More... | |
virtual void | ReportProximities (ChProximityContainer *mproximitycontainer)=0 |
After the Run() has completed, you can call this function to fill a 'proximity container' (container of narrow phase pairs), that is an object inherited from class ChProximityContainer. More... | |
void | RegisterBroadphaseCallback (std::shared_ptr< BroadphaseCallback > callback) |
Specify a callback object to be used each time a pair of 'near enough' collision shapes is found by the broad-phase collision step. More... | |
void | RegisterNarrowphaseCallback (std::shared_ptr< NarrowphaseCallback > callback) |
Specify a callback object to be used each time a collision pair is found during the narrow-phase collision detection step. More... | |
virtual bool | RayHit (const ChVector<> &from, const ChVector<> &to, ChRayhitResult &result) const =0 |
Perform a ray-hit test with the collision models. | |
virtual bool | RayHit (const ChVector<> &from, const ChVector<> &to, ChCollisionModel *model, ChRayhitResult &result) const =0 |
Perform a ray-hit test with the specified collision model. | |
virtual void | RegisterVisualizationCallback (std::shared_ptr< VisualizationCallback > callback) |
Specify a callback object to be used for debug rendering of collision shapes. | |
virtual void | Visualize (int flags) |
Method to trigger debug visualization of collision shapes. More... | |
virtual void | ArchiveOut (ChArchiveOut &marchive) |
Method to allow serialization of transient data to archives. | |
virtual void | ArchiveIn (ChArchiveIn &marchive) |
Method to allow de-serialization of transient data from archives. | |
void | SetSystem (ChSystem *sys) |
Set associated Chrono system. | |
Protected Attributes | |
ChSystem * | m_system |
associated Chrono system | |
std::shared_ptr< BroadphaseCallback > | broad_callback |
user callback for each near-enough pair of shapes | |
std::shared_ptr< NarrowphaseCallback > | narrow_callback |
user callback for each collision pair | |
std::shared_ptr< VisualizationCallback > | vis_callback |
user callback for debug visualization | |
int | m_vis_flags |
Member Enumeration Documentation
◆ VisualizationModes
Member Function Documentation
◆ PreProcess()
|
inlinevirtual |
Removes all collision models from the collision engine (custom data may be deallocated).
Optional synchronization operations, invoked before running the collision detection.
Reimplemented in chrono::collision::ChCollisionSystemChrono, and chrono::collision::ChCollisionSystemChronoMulticore.
◆ RegisterBroadphaseCallback()
|
inline |
Specify a callback object to be used each time a pair of 'near enough' collision shapes is found by the broad-phase collision step.
The OnBroadphase() method of the provided callback object will be called for each pair of 'near enough' shapes.
◆ RegisterNarrowphaseCallback()
|
inline |
Specify a callback object to be used each time a collision pair is found during the narrow-phase collision detection step.
The OnNarrowphase() method of the provided callback object will be called for each collision pair found during narrow phase.
◆ ReportContacts()
|
pure virtual |
After the Run() has completed, you can call this function to fill a 'contact container', that is an object inherited from class ChContactContainer.
For instance ChSystem, after each Run() collision detection, calls this method multiple times for all contact containers in the system, Children classes must implement this. The basic behavior of the implementation should be the following: collision system will call in sequence the functions BeginAddContact(), AddContact() (x n times), EndAddContact() of the contact container. In case a specialized implementation (ex. a GPU parallel collision engine) finds that the contact container is a specialized one (ex with a GPU buffer) it can call more performant methods to add directly the contacts in batches, for instance by recognizing that he can call, say, some special AddAllContactsAsGpuBuffer() instead of many AddContact().
Implemented in chrono::collision::ChCollisionSystemBulletMulticore, chrono::collision::ChCollisionSystemBullet, chrono::collision::ChCollisionSystemChrono, and chrono::collision::ChCollisionSystemChronoMulticore.
◆ ReportProximities()
|
pure virtual |
After the Run() has completed, you can call this function to fill a 'proximity container' (container of narrow phase pairs), that is an object inherited from class ChProximityContainer.
For instance ChSystem, after each Run() collision detection, calls this method multiple times for all proximity containers in the system, Children classes must implement this. The basic behavior of the implementation should be the following: collision system will call in sequence the functions BeginAddProximities(), AddProximity() (x n times), EndAddProximities() of the proximity container. In case a specialized implementation (ex. a GPU parallel collision engine) finds that the proximity container is a specialized one (ex with a GPU buffer) it can call more performant methods to add directly the proximities in batches, for instance by recognizing that he can call, say, some special AddAllProximitiesAsGpuBuffer() instead of many AddProximity().
Implemented in chrono::collision::ChCollisionSystemChrono, chrono::collision::ChCollisionSystemBullet, chrono::collision::ChCollisionSystemBulletMulticore, and chrono::collision::ChCollisionSystemChronoMulticore.
◆ Run()
|
pure virtual |
Run the collision detection and finds the contacts.
This function will be called at each simulation step.
Implemented in chrono::collision::ChCollisionSystemChrono, chrono::collision::ChCollisionSystemBulletMulticore, and chrono::collision::ChCollisionSystemBullet.
◆ SetNumThreads()
|
inlinevirtual |
Set the number of OpenMP threads for collision detection.
The default implementation does nothing. Derived classes implement this function as applicable.
Reimplemented in chrono::collision::ChCollisionSystemChrono, chrono::collision::ChCollisionSystemBulletMulticore, chrono::collision::ChCollisionSystemBullet, and chrono::collision::ChCollisionSystemChronoMulticore.
◆ Visualize()
|
inlinevirtual |
Method to trigger debug visualization of collision shapes.
The 'flags' argument can be any of the VisualizationModes enums, or a combination thereof (using bit-wise operators). The calling program must invoke this function from within the simulation loop. A derived class should implement a no-op if a visualization callback was not specified.
Reimplemented in chrono::collision::ChCollisionSystemChrono, and chrono::collision::ChCollisionSystemBullet.
The documentation for this class was generated from the following file:
- /builds/uwsbel/chrono/src/chrono/collision/ChCollisionSystem.h