Description
Chrono custom multicore collision system.
Contains both the broadphase and the narrow phase methods.
#include <ChCollisionSystemChronoMulticore.h>
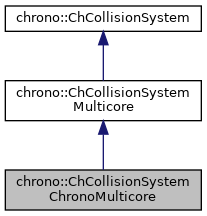
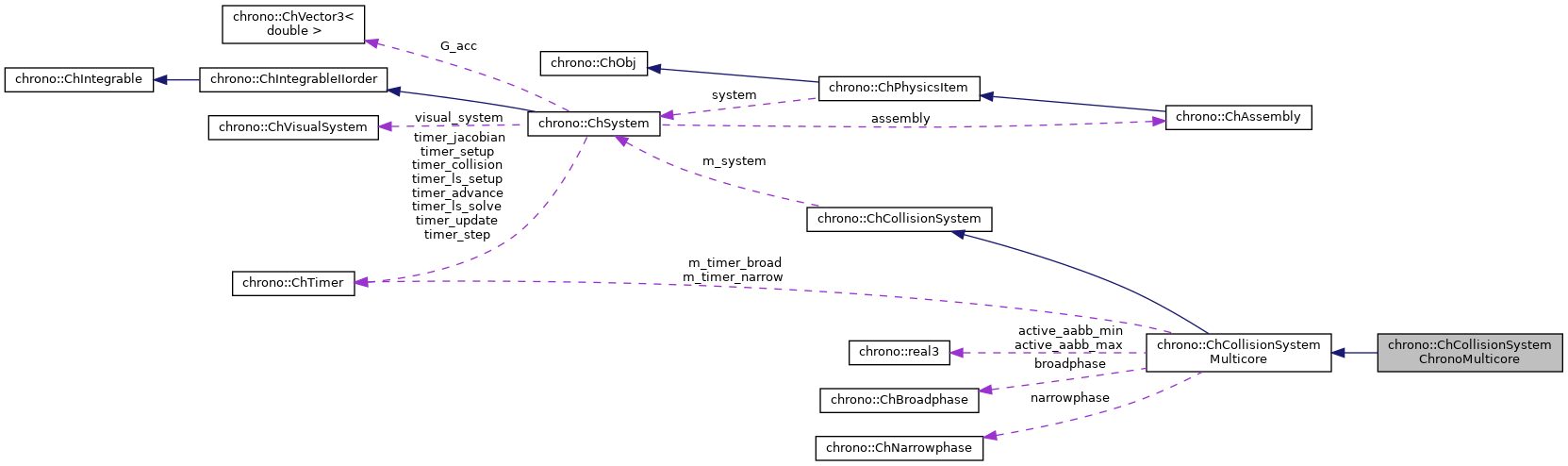
Public Member Functions | |
ChCollisionSystemChronoMulticore (ChMulticoreDataManager *dc) | |
virtual void | SetNumThreads (int nthreads) override |
Set the number of OpenMP threads for collision detection. | |
virtual void | PreProcess () override |
Synchronization operations, invoked before running the collision detection. More... | |
virtual void | PostProcess () override |
Synchronization operations, invoked after running the collision detection. | |
virtual void | ReportContacts (ChContactContainer *container) override |
Fill in the provided contact container with collision information after Run(). More... | |
virtual void | ReportProximities (ChProximityContainer *mproximitycontainer) override |
Fill in the provided proximity container with near point information after Run(). More... | |
![]() | |
virtual void | Clear () override |
Clears all data instanced by this algorithm if any (like persistent contact manifolds) | |
virtual void | Add (std::shared_ptr< ChCollisionModel > model) override |
Add the specified collision model to the collision engine. | |
virtual void | Remove (std::shared_ptr< ChCollisionModel > model) override |
Remove the specified collision model from the collision engine. | |
void | SetEnvelope (double envelope) |
Set collision envelope for rigid shapes (default: ChCollisionModel::GetDefaultSuggestedEnvelope). More... | |
void | SetBroadphaseGridResolution (const ChVector3i &num_bins) |
Set a fixed number of grid bins (default 10x10x10). More... | |
void | SetBroadphaseGridSize (const ChVector3d &bin_size) |
Set a variable number of grids, such that each bin has roughly the specified size. More... | |
void | SetBroadphaseGridDensity (double density) |
Set a variable number of grid bins, such that there are roughly density collision shapes per bin. More... | |
void | SetNarrowphaseAlgorithm (ChNarrowphase::Algorithm algorithm) |
Set the narrowphase algorithm (default: ChNarrowphase::Algorithm::HYBRID). More... | |
void | EnableActiveBoundingBox (const ChVector3d &aabb_min, const ChVector3d &aabb_max) |
Enable monitoring of shapes outside active bounding box (default: false). More... | |
bool | GetActiveBoundingBox (ChVector3d &aabb_min, ChVector3d &aabb_max) const |
Get the dimensions of the "active" box. More... | |
virtual void | Run () override |
Run the algorithm and finds all the contacts. | |
virtual ChAABB | GetBoundingBox () const override |
Return an AABB bounding all collision shapes in the system. | |
virtual void | ResetTimers () override |
Reset any timers associated with collision detection. | |
virtual double | GetTimerCollisionBroad () const override |
Return the time (in seconds) for broadphase collision detection. | |
virtual double | GetTimerCollisionNarrow () const override |
Return the time (in seconds) for narrowphase collision detection. | |
virtual bool | RayHit (const ChVector3d &from, const ChVector3d &to, ChRayhitResult &result) const override |
Perform a ray-hit test with all collision models. More... | |
virtual bool | RayHit (const ChVector3d &from, const ChVector3d &to, ChCollisionModel *model, ChRayhitResult &result) const override |
Perform a ray-hit test with the specified collision model. More... | |
virtual void | Visualize (int flags) override |
Method to trigger debug visualization of collision shapes. More... | |
virtual std::vector< vec2 > | GetOverlappingPairs () |
Return the pairs of IDs for overlapping contact shapes. | |
virtual void | ArchiveOut (ChArchiveOut &archive_out) override |
Method to allow serialization of transient data to archives. | |
virtual void | ArchiveIn (ChArchiveIn &archive_in) override |
Method to allow deserialization of transient data from archives. | |
![]() | |
bool | IsInitialized () const |
Test if the collision system was initialized. | |
virtual void | Initialize () |
Initialize the collision system. More... | |
virtual void | BindAll () |
Process all collision models in the associated Chrono system. More... | |
virtual void | BindItem (std::shared_ptr< ChPhysicsItem > item) |
Process the collision models associated with the specified Chrono physics item. More... | |
void | UnbindItem (std::shared_ptr< ChPhysicsItem > item) |
Remove any collision models associated with the specified physics item from the collision engine. | |
void | RegisterBroadphaseCallback (std::shared_ptr< BroadphaseCallback > callback) |
Specify a callback object to be used each time a pair of 'near enough' collision shapes is found by the broad-phase collision step. More... | |
void | RegisterNarrowphaseCallback (std::shared_ptr< NarrowphaseCallback > callback) |
Specify a callback object to be used each time a collision pair is found during the narrow-phase collision detection step. More... | |
virtual void | RegisterVisualizationCallback (std::shared_ptr< VisualizationCallback > callback) |
Specify a callback object to be used for debug rendering of collision shapes. | |
void | SetSystem (ChSystem *sys) |
Set associated Chrono system. | |
Friends | |
class | chrono::ChSystemMulticore |
Additional Inherited Members | |
![]() | |
enum | Type { Type::BULLET, Type::MULTICORE } |
Supported collision systems. More... | |
enum | VisualizationModes { VIS_None = 0, VIS_Shapes = 1 << 0, VIS_Aabb = 1 << 1, VIS_Contacts = 1 << 2, VIS_MAX_MODES } |
Enumeration of supported flags for collision debug visualization. More... | |
![]() | |
virtual void | GetOverlappingAABB (std::vector< char > &active_id, real3 Amin, real3 Amax) |
Mark bodies whose AABB is contained within the specified box. | |
void | GenerateAABB () |
Generate the current axis-aligned bounding boxes of collision shapes. | |
void | VisualizeShapes () |
Visualize collision shapes (wireframe). | |
void | VisualizeAABB () |
Visualize collision shape AABBs. | |
void | VisualizeContacts () |
Visualize contact points and normals. | |
![]() | |
std::vector< std::shared_ptr< ChCollisionModelMulticore > > | ct_models |
std::shared_ptr< ChCollisionData > | cd_data |
ChBroadphase | broadphase |
methods for broad-phase collision detection | |
ChNarrowphase | narrowphase |
methods for narrow-phase collision detection | |
std::vector< char > | body_active |
bool | use_aabb_active |
enable freezing of objects outside the active bounding box | |
real3 | active_aabb_min |
lower corner of active bounding box | |
real3 | active_aabb_max |
upper corner of active bounding box | |
ChTimer | m_timer_broad |
ChTimer | m_timer_narrow |
![]() | |
bool | m_initialized |
ChSystem * | m_system |
associated Chrono system | |
std::shared_ptr< BroadphaseCallback > | broad_callback |
user callback for each near-enough pair of shapes | |
std::shared_ptr< NarrowphaseCallback > | narrow_callback |
user callback for each collision pair | |
std::shared_ptr< VisualizationCallback > | vis_callback |
user callback for debug visualization | |
int | m_vis_flags |
Member Function Documentation
◆ PreProcess()
|
overridevirtual |
Synchronization operations, invoked before running the collision detection.
Different from the base class function, this overrides points to already allocated contactable state information (in the Chrono::Multicore data manager).
Reimplemented from chrono::ChCollisionSystemMulticore.
◆ ReportContacts()
|
overridevirtual |
Fill in the provided contact container with collision information after Run().
Different from the base class function, this override loads into a ChContactContainerMulticore.
Reimplemented from chrono::ChCollisionSystemMulticore.
◆ ReportProximities()
|
inlineoverridevirtual |
Fill in the provided proximity container with near point information after Run().
Not used.
Reimplemented from chrono::ChCollisionSystemMulticore.
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono_multicore/collision/ChCollisionSystemChronoMulticore.h
- /builds/uwsbel/chrono/src/chrono_multicore/collision/ChCollisionSystemChronoMulticore.cpp