Description
Simple finite element with two nodes and a spring/damper between the two nodes.
This element is mass-less, so if used in dynamic analysis, the two nodes must be set with non-zero point mass.
#include <ChElementSpring.h>
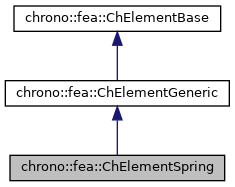
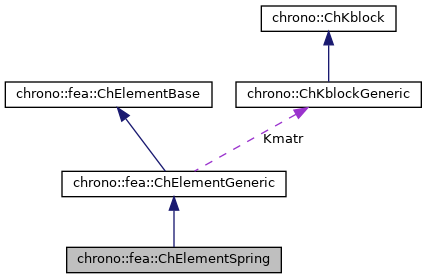
Public Member Functions | |
virtual int | GetNnodes () override |
Get the number of nodes used by this element. | |
virtual int | GetNdofs () override |
Get the number of coordinates in the field used by the referenced nodes. More... | |
virtual int | GetNodeNdofs (int n) override |
Get the number of coordinates from the specified node that are used by this element. More... | |
virtual std::shared_ptr< ChNodeFEAbase > | GetNodeN (int n) override |
Access the nth node. | |
virtual void | SetNodes (std::shared_ptr< ChNodeFEAxyz > nodeA, std::shared_ptr< ChNodeFEAxyz > nodeB) |
virtual void | GetStateBlock (ChVectorDynamic<> &mD) override |
Fills the D vector with the current field values at the nodes of the element, with proper ordering. More... | |
virtual void | ComputeKRMmatricesGlobal (ChMatrixRef H, double Kfactor, double Rfactor=0, double Mfactor=0) override |
Sets H as the global stiffness matrix K, scaled by Kfactor. More... | |
virtual void | ComputeInternalForces (ChVectorDynamic<> &Fi) override |
Computes the internal forces (ex. More... | |
virtual void | SetSpringK (double ms) |
Set the stiffness of the spring that connects the two nodes (N/m) | |
virtual double | GetSpringK () |
virtual void | SetDamperR (double md) |
Set the damping of the damper that connects the two nodes (Ns/M) | |
virtual double | GetDamperR () |
virtual double | GetCurrentForce () |
Get the current force transmitted along the spring direction, including the effect of the damper. More... | |
![]() | |
ChKblockGeneric & | Kstiffness () |
Access the proxy to stiffness, for sparse solver. | |
virtual void | EleIntLoadResidual_F (ChVectorDynamic<> &R, const double c) override |
Add the internal forces (pasted at global nodes offsets) into a global vector R, multiplied by a scaling factor c, as R += forces * c This default implementation is SLIGHTLY INEFFICIENT. | |
virtual void | EleIntLoadResidual_Mv (ChVectorDynamic<> &R, const ChVectorDynamic<> &w, const double c) override |
Add the product of element mass M by a vector w (pasted at global nodes offsets) into a global vector R, multiplied by a scaling factor c, as R += M * w * c This default implementation is VERY INEFFICIENT. | |
virtual void | EleIntLoadResidual_F_gravity (ChVectorDynamic<> &R, const ChVector<> &G_acc, const double c) override |
Add the contribution of gravity loads, multiplied by a scaling factor c, as: R += M * g * c This default implementation is VERY INEFFICIENT. More... | |
virtual void | ComputeGravityForces (ChVectorDynamic<> &Fg, const ChVector<> &G_acc) override |
Compute the gravitational forces. More... | |
virtual void | ComputeMmatrixGlobal (ChMatrixRef M) override |
Calculate the mass matrix, expressed in global reference. More... | |
virtual void | InjectKRMmatrices (ChSystemDescriptor &descriptor) override |
Tell to a system descriptor that there are item(s) of type ChKblock in this object (for further passing it to a solver) | |
virtual void | KRMmatricesLoad (double Kfactor, double Rfactor, double Mfactor) override |
Add the current stiffness K and damping R and mass M matrices in encapsulated ChKblock item(s), if any. More... | |
virtual void | VariablesFbLoadInternalForces (double factor=1.) override |
Add the internal forces, expressed as nodal forces, into the encapsulated ChVariables. | |
virtual void | VariablesFbIncrementMq () override |
Add M*q (internal masses multiplied current 'qb'). | |
![]() | |
virtual int | GetNdofs_active () |
Get the actual number of active degrees of freedom. More... | |
virtual int | GetNodeNdofs_active (int n) |
Get the actual number of active coordinates from the specified node that are used by this element. More... | |
virtual void | ComputeNodalMass () |
Compute element's nodal masses. | |
virtual void | Update () |
Update, called at least at each time step. More... | |
virtual void | EleDoIntegration () |
This is optionally implemented if there is some internal state that requires integration. | |
Additional Inherited Members | |
![]() | |
ChKblockGeneric | Kmatr |
Member Function Documentation
◆ ComputeInternalForces()
|
overridevirtual |
Computes the internal forces (ex.
the actual position of nodes is not in relaxed reference position) and set values in the Fi vector.
Implements chrono::fea::ChElementBase.
◆ ComputeKRMmatricesGlobal()
|
overridevirtual |
Sets H as the global stiffness matrix K, scaled by Kfactor.
Optionally, also superimposes global damping matrix R, scaled by Rfactor, and global mass matrix M multiplied by Mfactor. (For the spring matrix there is no need to corotate local matrices: we already know a closed form expression.)
Implements chrono::fea::ChElementBase.
◆ GetCurrentForce()
|
virtual |
Get the current force transmitted along the spring direction, including the effect of the damper.
Positive if pulled. (N)
◆ GetNdofs()
|
inlineoverridevirtual |
Get the number of coordinates in the field used by the referenced nodes.
This is for example the size (number of rows/columns) of the local stiffness matrix.
Implements chrono::fea::ChElementBase.
◆ GetNodeNdofs()
|
inlineoverridevirtual |
Get the number of coordinates from the specified node that are used by this element.
Note that this may be different from the value returned by GetNodeN(n)->GetNdofW().
Implements chrono::fea::ChElementBase.
◆ GetStateBlock()
|
overridevirtual |
Fills the D vector with the current field values at the nodes of the element, with proper ordering.
If the D vector has not the size of this->GetNdofs(), it will be resized.
Implements chrono::fea::ChElementBase.
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono/fea/ChElementSpring.h
- /builds/uwsbel/chrono/src/chrono/fea/ChElementSpring.cpp