Description
Driver.
#include <AIDriver.h>
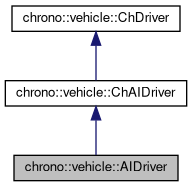

Public Member Functions | |
AIDriver (ChVehicle &vehicle, const std::string &filename) | |
Construct using data from the specified JSON file. More... | |
virtual double | CalculateSteering (double front_axle_angle, double rear_axle_angle) override |
Return the value of the vehicle steering input (in [-1,+1]) given the desired front and rear wheel angles. More... | |
![]() | |
ChAIDriver (ChVehicle &vehicle) | |
void | SetSpeedControllerGains (double Kp, double Ki, double Kd) |
Set speed controller PID gains. | |
void | SetThresholdThrottle (double val) |
Specify the throttle value below which braking is enabled. More... | |
ChSpeedController & | GetSpeedController () |
Get the underlying speed controller object. | |
void | Synchronize (double time, double long_acc, double front_axle_angle, double rear_axle_angle) |
Set the controls from the autonomy stack: longitudinal acceleration (m/s2) and the front & rear wheel angles (in radians). More... | |
virtual void | Advance (double step) override |
Advance the state of this driver system by the specified duration. | |
![]() | |
ChDriver (ChVehicle &vehicle) | |
double | GetThrottle () const |
Get the driver throttle input (in the range [0,1]) | |
double | GetSteering () const |
Get the driver steering input (in the range [-1,+1]) | |
double | GetBraking () const |
Get the driver braking input (in the range [0,1]) | |
Inputs | GetInputs () const |
Get all current inputs at once. | |
virtual void | Initialize () |
Initialize this driver system. | |
bool | LogInit (const std::string &filename) |
Initialize output file for recording driver inputs. | |
bool | Log (double time) |
Record the current driver inputs to the log file. | |
void | SetSteering (double val, double min_val=-1, double max_val=1) |
Overwrite the value for the driver steering input. | |
void | SetThrottle (double val, double min_val=0, double max_val=1) |
Overwrite the value for the driver throttle input. | |
void | SetBraking (double val, double min_val=0, double max_val=1) |
Overwrite the value for the driver braking input. | |
Additional Inherited Members | |
![]() | |
ChSpeedController | m_speedPID |
speed controller | |
double | m_throttle_threshold |
throttle value below which brakes are applied | |
double | m_target_speed |
current value of target speed | |
double | m_last_time |
last time | |
double | m_last_speed |
last target speed | |
![]() | |
ChVehicle & | m_vehicle |
reference to associated vehicle | |
double | m_throttle |
current value of throttle input | |
double | m_steering |
current value of steering input | |
double | m_braking |
current value of braking input | |
Constructor & Destructor Documentation
◆ AIDriver()
chrono::vehicle::AIDriver::AIDriver | ( | ChVehicle & | vehicle, |
const std::string & | filename | ||
) |
Construct using data from the specified JSON file.
- Parameters
-
vehicle associated vehicle filename name of JSON specification file
Member Function Documentation
◆ CalculateSteering()
|
overridevirtual |
Return the value of the vehicle steering input (in [-1,+1]) given the desired front and rear wheel angles.
The underlying assumption is of Ackermann steering of a bicycle model. The current implementation ignores the rear angle (always 0 for a front steering vehicle with Ackermann geometry) and uses a mapping from front angle to steering input. Note that the Chrono::Vehicle ISO reference frame convention implies that a positive front angle corresponds to a turn to the left (i.e., positive value of vehicle steering input).
Implements chrono::vehicle::ChAIDriver.
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono_vehicle/driver/AIDriver.h
- /builds/uwsbel/chrono/src/chrono_vehicle/driver/AIDriver.cpp