Description
Geometric object representing a NURBS surface.
#include <ChSurfaceNurbs.h>
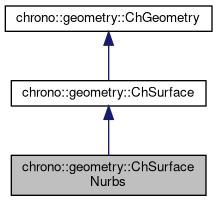
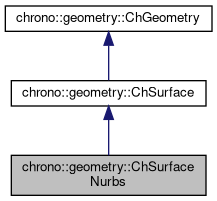
Public Member Functions | |
ChSurfaceNurbs () | |
Constructor. By default, a segment (order = 1, two points on X axis, at -1, +1) | |
ChSurfaceNurbs (int morder_u, int morder_v, ChMatrixDynamic< ChVector<>> &mpoints, ChVectorDynamic<> *mknots_u=0, ChVectorDynamic<> *mknots_v=0, ChMatrixDynamic<> *weights=0) | |
Constructor from a given array of control points. More... | |
ChSurfaceNurbs (const ChSurfaceNurbs &source) | |
virtual ChSurfaceNurbs * | Clone () const override |
"Virtual" copy constructor (covariant return type). | |
virtual void | Evaluate (ChVector<> &pos, const double parU, const double parV) const override |
Evaluates a point on the line, given parametric coordinate U. More... | |
double | ComputeUfromKnotU (const double u) const |
Evaluates normal. More... | |
double | ComputeKnotUfromU (const double U) const |
When using Evaluate() etc. More... | |
double | ComputeVfromKnotV (const double v) const |
When using Evaluate() etc. More... | |
double | ComputeKnotVfromV (const double V) const |
When using Evaluate() etc. More... | |
ChMatrixDynamic< ChVector<> > & | Points () |
Access the points. | |
ChMatrixDynamic & | Weights () |
Access the weights. | |
ChVectorDynamic & | Knots_u () |
Access the U knots. | |
ChVectorDynamic & | Knots_v () |
Access the U knots. | |
int | GetOrder_u () |
Get the order in u direction. | |
int | GetOrder_v () |
Get the order in v direction. | |
virtual void | SetupData (int morder_u, int morder_v, ChMatrixDynamic< ChVector<> > &mpoints, ChVectorDynamic<> *mknots_u=0, ChVectorDynamic<> *mknots_v=0, ChMatrixDynamic<> *weights=0) |
Initial easy setup from a given array of control points. More... | |
virtual void | ArchiveOUT (ChArchiveOut &marchive) override |
Method to allow serialization of transient data to archives. | |
virtual void | ArchiveIN (ChArchiveIn &marchive) override |
Method to allow de-serialization of transient data from archives. | |
![]() | |
ChSurface (const ChSurface &source) | |
virtual void | Normal (ChVector<> &dir, const double parU, const double parV) const |
Evaluates a normal versor, given parametric coordinates U,V. More... | |
virtual bool | Get_closed_U () const |
Tell if the surface is closed (periodic) on U. | |
virtual bool | Get_closed_V () const |
Tell if the surface is closed (periodic) on V. | |
virtual int | GetManifoldDimension () const override |
This is a surface, so manifold dimension=2. | |
bool | IsWireframe () |
Tell if the visualization is done only as UV isolines. | |
void | SetWireframe (bool mw) |
Set if the visualization is done only as UV isolines. | |
![]() | |
ChGeometry (const ChGeometry &source) | |
virtual GeometryType | GetClassType () const |
Get the class type as unique numerical ID (faster than using ChronoRTTI mechanism). More... | |
virtual void | GetBoundingBox (double &xmin, double &xmax, double &ymin, double &ymax, double &zmin, double &zmax, ChMatrix33<> *Rot=nullptr) const |
Compute bounding box. More... | |
virtual void | InflateBoundingBox (double &xmin, double &xmax, double &ymin, double &ymax, double &zmin, double &zmax, ChMatrix33<> *Rot=NULL) const |
Enlarge a previous existing bounding box. More... | |
virtual double | Size () const |
Returns the radius of the sphere which can enclose the geometry. | |
virtual ChVector | Baricenter () const |
Compute center of mass It should be overridden by inherited classes. | |
virtual void | CovarianceMatrix (ChMatrix33<> &C) const |
Compute the 3x3 covariance matrix (only the diagonal and upper part) It should be overridden by inherited classes. | |
virtual void | Update () |
Generic update of internal data. More... | |
Public Attributes | |
ChMatrixDynamic< ChVector<> > | points |
ChMatrixDynamic | weights |
ChVectorDynamic | knots_u |
ChVectorDynamic | knots_v |
int | p_u |
int | p_v |
Additional Inherited Members | |
![]() | |
enum | GeometryType { NONE, SPHERE, BOX, CYLINDER, TRIANGLE, CAPSULE, CONE, LINE, LINE_ARC, LINE_BEZIER, LINE_CAM, LINE_PATH, LINE_POLY, LINE_SEGMENT, ROUNDED_BOX, ROUNDED_CYLINDER, ROUNDED_CONE, TRIANGLEMESH, TRIANGLEMESH_CONNECTED, TRIANGLEMESH_SOUP } |
Enumeration of geometric objects. | |
![]() | |
bool | wireframe |
Constructor & Destructor Documentation
◆ ChSurfaceNurbs()
chrono::geometry::ChSurfaceNurbs::ChSurfaceNurbs | ( | int | morder_u, |
int | morder_v, | ||
ChMatrixDynamic< ChVector<>> & | mpoints, | ||
ChVectorDynamic<> * | mknots_u = 0 , |
||
ChVectorDynamic<> * | mknots_v = 0 , |
||
ChMatrixDynamic<> * | weights = 0 |
||
) |
Constructor from a given array of control points.
Input data is copied. If the knots are not provided, a uniformly spaced knot vector is made. If the weights are not provided, a constant weight vector is made.
- Parameters
-
morder_u order pu: 1= linear, 2=quadratic, etc. morder_v order pv: 1= linear, 2=quadratic, etc. mpoints control points, size nuxnv. Required: nu at least pu+1, same for v mknots_u knots, size ku. Required ku=nu+pu+1. If not provided, initialized to uniform. mknots_v knots, size kv. Required ku=nu+pu+1. If not provided, initialized to uniform. weights weights, size nuxnv. If not provided, all weights as 1.
Member Function Documentation
◆ ComputeKnotUfromU()
|
inline |
When using Evaluate() etc.
you need U parameter to be in 0..1 range, but knot range is not necessarily in 0..1. So you can convert U->u, where u is in knot range, calling this:
◆ ComputeKnotVfromV()
|
inline |
When using Evaluate() etc.
you need V parameter to be in 0..1 range, but knot range is not necessarily in 0..1. So you can convert V->v, where v is in knot range, calling this:
◆ ComputeUfromKnotU()
|
inline |
Evaluates normal.
When using Evaluate() etc. you need U parameter to be in 0..1 range, but knot range is not necessarily in 0..1. So you can convert u->U, where u is in knot range, calling this:
◆ ComputeVfromKnotV()
|
inline |
When using Evaluate() etc.
you need V parameter to be in 0..1 range, but knot range is not necessarily in 0..1. So you can convert v->V, where v is in knot range, calling this:
◆ Evaluate()
|
overridevirtual |
Evaluates a point on the line, given parametric coordinate U.
Parameter U always work in 0..1 range, even if knots are not in 0..1 range. So if you want to use u' in knot range, use ComputeUfromKnotU(). Computed value goes into the 'pos' reference. It must be implemented by inherited classes.
Implements chrono::geometry::ChSurface.
◆ SetupData()
|
virtual |
Initial easy setup from a given array of control points.
Input data is copied. If the knots are not provided, a uniformly spaced knot vector is made. If the weights are not provided, a constant weight vector is made.
- Parameters
-
morder_u order pu: 1= linear, 2=quadratic, etc. morder_v order pv: 1= linear, 2=quadratic, etc. mpoints control points, size nuxnv. Required: at least nu >= pu+1, same for v mknots_u knots u, size ku. Required ku=nu+pu+1. If not provided, initialized to uniform. mknots_v knots v, size kv. Required ku=nu+pu+1. If not provided, initialized to uniform. weights weights, size nuxnv. If not provided, all weights as 1.
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono/geometry/ChSurfaceNurbs.h
- /builds/uwsbel/chrono/src/chrono/geometry/ChSurfaceNurbs.cpp