Description
Granular terrain model.
This class implements a rectangular patch of granular terrain with spherical particles. Boundary conditions (model of a container bin) are imposed through a custom collision detection object.
#include <GranularTerrain.h>
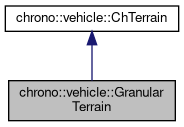
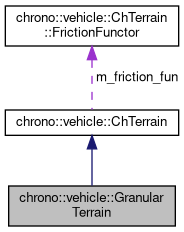
Public Member Functions | |
GranularTerrain (ChSystem *system) | |
Construct a default GranularTerrain. More... | |
void | SetContactFrictionCoefficient (float friction_coefficient) |
Set coefficient of friction. More... | |
void | SetContactRestitutionCoefficient (float restitution_coefficient) |
Set coefficient of restitution. More... | |
void | SetContactCohesion (float cohesion) |
Set the cohesion constant. More... | |
void | SetContactMaterialProperties (float young_modulus, float poisson_ratio) |
Set contact material properties. More... | |
void | SetContactMaterialCoefficients (float kn, float gn, float kt, float gt) |
Set contact material coefficients. More... | |
void | SetContactMaterialSMC (std::shared_ptr< ChMaterialSurfaceSMC > mat) |
Set contact material for SMC method. | |
void | SetContactMaterialNSC (std::shared_ptr< ChMaterialSurfaceNSC > mat) |
Set contact material for NSC method. | |
void | SetCollisionEnvelope (double envelope) |
Set outward collision envelope. More... | |
void | SetMinNumParticles (unsigned int min_num_particles) |
Set the minimum number of particles to be generated (default: 0). | |
void | EnableVerbose (bool val) |
Enable/disable verbose output (default: false). | |
void | EnableRoughSurface (int num_spheres_x, int num_spheres_y) |
Enable creation of particles fixed to bottom container. More... | |
void | EnableMovingPatch (std::shared_ptr< ChBody > body, double buffer_distance, double shift_distance, const ChVector<> &init_vel=ChVector<>()) |
Enable moving patch and set parameters. More... | |
void | SetStartIdentifier (int id) |
Set start value for body identifiers of generated particles (default: 1000000). More... | |
float | GetCoefficientFriction () const |
Get coefficient of friction for contact material. | |
float | GetCoefficientRestitution () const |
Get coefficient of restitution for contact material. | |
float | GetCohesion () const |
Get cohesion constant. | |
float | GetYoungModulus () const |
Get Young's modulus of elasticity for contact material. | |
float | GetPoissonRatio () const |
Get Poisson ratio for contact material. | |
float | GetKn () const |
Get normal stiffness coefficient for contact material. | |
float | GetKt () const |
Get tangential stiffness coefficient for contact material. | |
float | GetGn () const |
Get normal viscous damping coefficient for contact material. | |
float | GetGt () const |
Get tangential viscous damping coefficient for contact material. | |
void | EnableVisualization (bool val) |
Enable/disable visualization of boundaries (default: false). | |
bool | IsVisualizationEnabled () const |
void | SetColor (ChColor color) |
Set boundary visualization color. | |
std::shared_ptr< ChBody > | GetGroundBody () |
Return a handle to the ground body. | |
void | Initialize (const ChVector<> ¢er, double length, double width, unsigned int num_layers, double radius, double density, const ChVector<> &init_vel=ChVector<>()) |
Initialize the granular terrain system. More... | |
virtual void | Synchronize (double time) override |
Update the state of the terrain system at the specified time. | |
double | GetPatchFront () const |
Get current front boundary location (in positive X direction). | |
double | GetPatchRear () const |
Get current rear boundary location (in negative X direction). | |
double | GetPatchLeft () const |
Get left boundary location (in positive Y direction). | |
double | GetPatchRight () const |
Get right boundary location (in negative Y direction). | |
double | GetPatchBottom () const |
Get bottom boundary location. | |
bool | PatchMoved () const |
Report if the patch was moved during the last call to Synchronize(). | |
unsigned int | GetNumParticles () const |
Get the number of particles. | |
virtual double | GetHeight (double x, double y) const override |
Get the terrain height at the specified (x,y) location. More... | |
virtual chrono::ChVector | GetNormal (double x, double y) const override |
Get the terrain normal at the specified (x,y) location. | |
virtual float | GetCoefficientFriction (double x, double y) const override |
Get the terrain coefficient of friction at the specified (x,y) location. More... | |
![]() | |
virtual void | Advance (double step) |
Advance the state of the terrain system by the specified duration. | |
void | RegisterFrictionFunctor (FrictionFunctor *functor) |
Specify the functor object to provide the coefficient of friction at given (x,y) locations. | |
Friends | |
class | BoundaryContact |
Additional Inherited Members | |
![]() | |
FrictionFunctor * | m_friction_fun |
functor for location-dependent coefficient of friction | |
Constructor & Destructor Documentation
◆ GranularTerrain()
chrono::vehicle::GranularTerrain::GranularTerrain | ( | ChSystem * | system | ) |
Construct a default GranularTerrain.
The user is responsible for calling various Set methods before Initialize.
- Parameters
-
[in] system pointer to the containing multibody system
Member Function Documentation
◆ EnableMovingPatch()
void chrono::vehicle::GranularTerrain::EnableMovingPatch | ( | std::shared_ptr< ChBody > | body, |
double | buffer_distance, | ||
double | shift_distance, | ||
const ChVector<> & | init_vel = ChVector<>() |
||
) |
Enable moving patch and set parameters.
- Parameters
-
body monitored body buffer_distance look-ahead distance shift_distance chunk size of relocated particles init_vel initial particle velocity
◆ EnableRoughSurface()
void chrono::vehicle::GranularTerrain::EnableRoughSurface | ( | int | num_spheres_x, |
int | num_spheres_y | ||
) |
Enable creation of particles fixed to bottom container.
- Parameters
-
num_spheres_x number of fixed spheres in X direction num_spheres_y number of fixed spheres in Y direction
◆ GetCoefficientFriction()
|
overridevirtual |
Get the terrain coefficient of friction at the specified (x,y) location.
This coefficient of friction value may be used by certain tire models to modify the tire characteristics, but it will have no effect on the interaction of the terrain with other objects (including tire models that do not explicitly use it). For GranularTerrain, this function defers to the user-provided functor object of type ChTerrain::FrictionFunctor, if one was specified. Otherwise, it returns the constant value specified through SetContactFrictionCoefficient.
Implements chrono::vehicle::ChTerrain.
◆ GetHeight()
|
overridevirtual |
Get the terrain height at the specified (x,y) location.
This function returns the highest point over all granular particles.
Implements chrono::vehicle::ChTerrain.
◆ Initialize()
void chrono::vehicle::GranularTerrain::Initialize | ( | const ChVector<> & | center, |
double | length, | ||
double | width, | ||
unsigned int | num_layers, | ||
double | radius, | ||
double | density, | ||
const ChVector<> & | init_vel = ChVector<>() |
||
) |
Initialize the granular terrain system.
The granular material is created in successive layers within the specified volume, using the specified generator, until the number of particles exceeds the specified minimum value (see SetMinNumParticles). The initial particle locations are obtained with Poisson Disk sampling, using the given minimum separation distance.
- Parameters
-
[in] center center of bottom [in] length patch dimension in X direction [in] width patch dimension in Y direction [in] num_layers number of layers [in] radius particle radius [in] density particle density [in] init_vel particle initial velocity
◆ SetCollisionEnvelope()
|
inline |
Set outward collision envelope.
This value is used for the internal custom collision detection for imposing boundary conditions. Note that if the underlying system is of SMC type (i.e., using a penalty-based contact method), the envelope is automatically set to 0. For NSC systems (i.e., when using a complementarity-based contact method), if the envelope is not specified, the default value is 5% of the particle radius.
◆ SetContactCohesion()
void chrono::vehicle::GranularTerrain::SetContactCohesion | ( | float | cohesion | ) |
Set the cohesion constant.
The default value is 0.
◆ SetContactFrictionCoefficient()
void chrono::vehicle::GranularTerrain::SetContactFrictionCoefficient | ( | float | friction_coefficient | ) |
Set coefficient of friction.
The default value is 0.9
◆ SetContactMaterialCoefficients()
void chrono::vehicle::GranularTerrain::SetContactMaterialCoefficients | ( | float | kn, |
float | gn, | ||
float | kt, | ||
float | gt | ||
) |
Set contact material coefficients.
These values are used directly to compute contact forces (if the containing system is so configured and if the SMC contact method is being used). The default values are: kn=2e5, gn=40, kt=2e5, gt=20
- Parameters
-
[in] kn normal contact stiffness [in] gn normal contact damping [in] kt tangential contact stiffness [in] gt tangential contact damping
◆ SetContactMaterialProperties()
void chrono::vehicle::GranularTerrain::SetContactMaterialProperties | ( | float | young_modulus, |
float | poisson_ratio | ||
) |
Set contact material properties.
These values are used to calculate contact material coefficients (if the containing system is so configured and if the SMC contact method is being used). The default values are: Y = 2e5 and nu = 0.3
- Parameters
-
[in] young_modulus Young's modulus of elasticity [in] poisson_ratio Poisson ratio
◆ SetContactRestitutionCoefficient()
void chrono::vehicle::GranularTerrain::SetContactRestitutionCoefficient | ( | float | restitution_coefficient | ) |
Set coefficient of restitution.
The default value is 0.
◆ SetStartIdentifier()
|
inline |
Set start value for body identifiers of generated particles (default: 1000000).
It is assumed that all bodies with a larger identifier are granular material particles.
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono_vehicle/terrain/GranularTerrain.h
- /builds/uwsbel/chrono/src/chrono_vehicle/terrain/GranularTerrain.cpp