chrono::vehicle::ChDataDriverSTR Class Reference
Description
Driver inputs for a suspension test rig from data file.
A driver model based on user inputs provided as time series. If provided as a text file, each line in the file must contain 4 values:
time left_post right_post steering
It is assumed that the time values are unique. If the time values are not sorted, this must be specified at construction. Inputs for post_left, post_right, and steering are assumed to be normalized in the range [-1,1]. Driver inputs at intermediate times are obtained through cubic spline interpolation.
#include <ChDataDriverSTR.h>
Inheritance diagram for chrono::vehicle::ChDataDriverSTR:
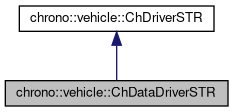
Collaboration diagram for chrono::vehicle::ChDataDriverSTR:
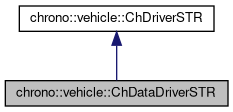
Public Member Functions | |
ChDataDriverSTR (const std::string &filename) | |
Construct using data from the specified file. | |
virtual bool | Ended () const override |
Return true when driver stopped producing inputs (end of data). | |
![]() | |
double | GetDisplacementLeft () const |
Get the left post vertical displacement (in the range [-1,+1]) | |
double | GetDisplacementRight () const |
Get the right post vertical displacement (in the range [-1,+1]) | |
double | GetDisplacementSpeedLeft () const |
Get the left post displacement rate of change. | |
double | GetDisplacementSpeedRight () const |
Get the right post displacement rate of change. | |
double | GetSteering () const |
Get the driver steering input (in the range [-1,+1]) | |
bool | Started () const |
Return false while driver inputs are ignored (while the rig is reaching the ride height configuration) and true otherwise. More... | |
bool | LogInit (const std::string &filename) |
Initialize output file for recording driver inputs. | |
bool | Log (double time) |
Record the current driver inputs to the log file. | |
Additional Inherited Members | |
![]() | |
virtual void | Initialize () |
Initialize this driver system. | |
void | SetDisplacementLeft (double val, double min_val=-1, double max_val=1) |
Set the value for the driver left post displacement input. | |
void | SetDisplacementRight (double val, double min_val=-1, double max_val=1) |
Set the value for the driver right post displacement input. | |
void | SetSteering (double val, double min_val=-1, double max_val=1) |
Set the value for the driver steering input. | |
![]() | |
double | m_displLeft |
current value of left post displacement | |
double | m_displRight |
current value of right post displacement | |
double | m_displSpeedLeft |
current value of left post displacement rate of change | |
double | m_displSpeedRight |
current value of right post displacement rate of change | |
double | m_steering |
current value of steering input | |
double | m_delay |
time delay before generating inputs | |
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono_vehicle/wheeled_vehicle/test_rig/ChDataDriverSTR.h
- /builds/uwsbel/chrono/src/chrono_vehicle/wheeled_vehicle/test_rig/ChDataDriverSTR.cpp