Description
Class to calculate force between SPH markers in Weakly Compressible SPH.
This is an abstract class that defines an interface that various SPH method should implement. The class owns a collision system fsi which takes care of GPU based proximity computation of the markers. It also holds a pointer to external data of SPH markers, proximity data, parameters, and numbers. Child class must implement Finalize and ForceSPH methods
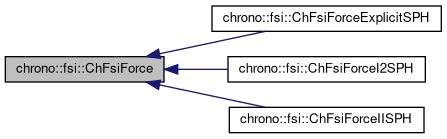
Public Member Functions | |
ChFsiForce (std::shared_ptr< ChBce > otherBceWorker, std::shared_ptr< SphMarkerDataD > otherSortedSphMarkersD, std::shared_ptr< ProximityDataD > otherMarkersProximityD, std::shared_ptr< FsiGeneralData > otherFsiGeneralData, std::shared_ptr< SimParams > otherParamsH, std::shared_ptr< NumberOfObjects > otherNumObjects) | |
Base constructor for FSI force class. More... | |
virtual | ~ChFsiForce () |
Destructor. Deletes the collision system. | |
virtual void | ForceSPH (std::shared_ptr< SphMarkerDataD > otherSphMarkersD, std::shared_ptr< FsiBodiesDataD > otherFsiBodiesD, std::shared_ptr< FsiMeshDataD > fsiMeshD)=0 |
Function calculate the force on SPH markers. More... | |
virtual void | Finalize () |
Synchronize the copy of the data (parameters and number of objects) between device (GPU) and host (CPU). More... | |
void | SetLinearSolver (ChFsiLinearSolver::SolverType other_solverType) |
Static Public Member Functions | |
static void | CopySortedToOriginal_Invasive_R3 (thrust::device_vector< Real3 > &original, thrust::device_vector< Real3 > &sorted, const thrust::device_vector< uint > &gridMarkerIndex) |
Copy sorted data into original data. More... | |
static void | CopySortedToOriginal_NonInvasive_R3 (thrust::device_vector< Real3 > &original, const thrust::device_vector< Real3 > &sorted, const thrust::device_vector< uint > &gridMarkerIndex) |
Copy sorted data into original data. More... | |
static void | CopySortedToOriginal_Invasive_R4 (thrust::device_vector< Real4 > &original, thrust::device_vector< Real4 > &sorted, const thrust::device_vector< uint > &gridMarkerIndex) |
Copy sorted data into original data. More... | |
static void | CopySortedToOriginal_NonInvasive_R4 (thrust::device_vector< Real4 > &original, thrust::device_vector< Real4 > &sorted, const thrust::device_vector< uint > &gridMarkerIndex) |
Copy sorted data into original data. More... | |
Public Attributes | |
std::shared_ptr< ChFsiLinearSolver > | myLinearSolver |
std::shared_ptr< ChBce > | bceWorker |
pointer to Boundary Condition Enforcing markers class. | |
std::shared_ptr< ChCollisionSystemFsi > | fsiCollisionSystem |
collision system; takes care of constructing neighbors list | |
std::shared_ptr< SphMarkerDataD > | sphMarkersD |
device copy of the sph markers data | |
std::shared_ptr< SphMarkerDataD > | sortedSphMarkersD |
device copy of the sorted sph markers data | |
std::shared_ptr< ProximityDataD > | markersProximityD |
pointer object that holds the proximity of the markers | |
std::shared_ptr< FsiGeneralData > | fsiGeneralData |
pointer to sph general data | |
std::shared_ptr< SimParams > | paramsH |
pointer to simulation parameters | |
std::shared_ptr< NumberOfObjects > | numObjectsH |
pointer to number of objects, fluid and boundary markers | |
thrust::device_vector< Real3 > | vel_vis_Sorted_D |
sorted visualization velocity data | |
thrust::device_vector< Real3 > | vel_XSPH_Sorted_D |
sorted xsph velocity data | |
thrust::device_vector< Real4 > | derivVelRhoD_Sorted_D |
sorted derivVelRhoD | |
Constructor & Destructor Documentation
◆ ChFsiForce()
chrono::fsi::ChFsiForce::ChFsiForce | ( | std::shared_ptr< ChBce > | otherBceWorker, |
std::shared_ptr< SphMarkerDataD > | otherSortedSphMarkersD, | ||
std::shared_ptr< ProximityDataD > | otherMarkersProximityD, | ||
std::shared_ptr< FsiGeneralData > | otherFsiGeneralData, | ||
std::shared_ptr< SimParams > | otherParamsH, | ||
std::shared_ptr< NumberOfObjects > | otherNumObjects | ||
) |
Base constructor for FSI force class.
The constructor instantiates the collision system (ChCollisionSystemFsi) and initializes the pointer to external data.
- Parameters
-
otherBceWorker Pointer to the ChBce object that handles BCE markers otherSortedSphMarkersD Information of markers in the sorted array on device otherMarkersProximityD Pointer to the object that holds the proximity of the markers on device otherFsiGeneralData Pointer to the sph general data otherParamsH Pointer to the simulation parameters on host otherNumObjects Pointer to number of objects, fluid and boundary markers, etc.
Member Function Documentation
◆ CopySortedToOriginal_Invasive_R3()
|
static |
Copy sorted data into original data.
This function copies the data that are sorted in the collision system, into the original data, where data is real3. The class is invasive, meaning that the sorted data will be modified (and will be equivalent to the original). Therefore, this function should be used whenever sorted data is not needed, but efficiency is preferred.
◆ CopySortedToOriginal_Invasive_R4()
|
static |
Copy sorted data into original data.
This function copies the data that are sorted in the collision system, into the original data, where data is real4. The class is invasive, meaning that the sorted data will be modified (and will be equivalent to the original). Therefore, this function should be used whenever sorted data is not needed, but efficiency is preferred.
◆ CopySortedToOriginal_NonInvasive_R3()
|
static |
Copy sorted data into original data.
This function copies the data that are sorted in the collision system, into the original data, where data is real3. The class is non-invasive, meaning that the sorted data will not be modified. This comes at the expense of lower efficiency.
◆ CopySortedToOriginal_NonInvasive_R4()
|
static |
Copy sorted data into original data.
This function copies the data that are sorted in the collision system, into the original data, where data is real4. The class is non-invasive, meaning that the sorted data will not be modified. This comes at the expense of lower efficiency.
◆ Finalize()
|
virtual |
Synchronize the copy of the data (parameters and number of objects) between device (GPU) and host (CPU).
This function needs to be called once the host data are modified.
Reimplemented in chrono::fsi::ChFsiForceI2SPH, chrono::fsi::ChFsiForceExplicitSPH, and chrono::fsi::ChFsiForceIISPH.
◆ ForceSPH()
|
pure virtual |
Function calculate the force on SPH markers.
This is a basic force computation relying on WCSPH approach. This is a virtual method that needs to be overridden by the child classes to compute fluid forces in an implicit integrator.
The documentation for this class was generated from the following file:
- /builds/uwsbel/chrono/src/chrono_fsi/physics/ChFsiForce.cuh