Description
Base class for properties of beam sections of Cosserat type (with shear too).
A beam section can be shared between multiple beams. A beam section contains the models for elasticity, plasticity, damping, etc.
#include <ChBeamSectionCosserat.h>
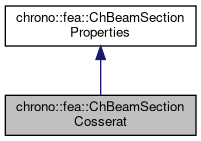
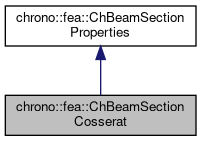
Public Member Functions | |
ChBeamSectionCosserat (std::shared_ptr< ChElasticityCosserat > melasticity) | |
ChBeamSectionCosserat (std::shared_ptr< ChElasticityCosserat > melasticity, std::shared_ptr< ChPlasticityCosserat > mplasticity) | |
ChBeamSectionCosserat (std::shared_ptr< ChElasticityCosserat > melasticity, std::shared_ptr< ChPlasticityCosserat > mplasticity, std::shared_ptr< ChDampingCosserat > mdamping) | |
virtual void | ComputeStress (ChVector<> &stress_n, ChVector<> &stress_m, const ChVector<> &strain_e, const ChVector<> &strain_k, ChBeamMaterialInternalData *mdata_new=nullptr, const ChBeamMaterialInternalData *mdata=nullptr) |
Compute the generalized cut force and cut torque, given the actual generalized section strain expressed as deformation vector e and curvature k, that is: {F,M}=f({e,k}), and given the actual material state required for plasticity if any (but if mdata=nullptr, computes only the elastic force). More... | |
virtual void | ComputeStiffnessMatrix (ChMatrixDynamic<> &K, const ChVector<> &strain_e, const ChVector<> &strain_k, const ChBeamMaterialInternalData *mdata=nullptr) |
Compute the 6x6 tangent material stiffness matrix [Km] = dσ/dε Compute the 6x6 tangent material stiffness matrix [Km] = dσ/dε at a given strain state, and at given internal data state (if mdata=nullptr, computes only the elastic tangent stiffenss, regardless of plasticity). More... | |
void | SetElasticity (std::shared_ptr< ChElasticityCosserat > melasticity) |
Set the elasticity model for this section. More... | |
std::shared_ptr< ChElasticityCosserat > | GetElasticity () |
Get the elasticity model for this section. More... | |
void | SetPlasticity (std::shared_ptr< ChPlasticityCosserat > mplasticity) |
Set the plasticity model for this section. More... | |
std::shared_ptr< ChPlasticityCosserat > | GetPlasticity () |
Get the elasticity model for this section, if any. More... | |
void | SetDamping (std::shared_ptr< ChDampingCosserat > mdamping) |
Set the damping model for this section. More... | |
std::shared_ptr< ChDampingCosserat > | GetDamping () |
Get the damping model for this section. More... | |
virtual void | SetAsRectangularSection (double width_y, double width_z) override |
Shortcut: set elastic and plastic constants at once, given the y and z widths of the beam assumed with rectangular shape. | |
virtual void | SetAsCircularSection (double diameter) override |
Shortcut: set elastic and plastic constants at once, given the diameter of the beam assumed with circular shape. | |
![]() | |
void | SetDrawThickness (double thickness_y, double thickness_z) |
Sets the rectangular thickness of the beam on y and z directions, only for drawing/rendering purposes (these thickness values do NOT have any meaning at a physical level, use SetAsRectangularSection() | |
double | GetDrawThicknessY () |
double | GetDrawThicknessZ () |
bool | IsCircular () |
Tells if the section must be drawn as a circular section instead than default rectangular. | |
void | SetCircular (bool ic) |
Set if the section must be drawn as a circular section instead than default rectangular. | |
void | SetDrawCircularRadius (double draw_rad) |
Sets the radius of the beam if in 'circular section' draw mode, only for drawing/rendering purposes (this radius value do NOT have any meaning at a physical level, use ChBeamSectionBasic::SetAsCircularSection() | |
double | GetDrawCircularRadius () |
void | SetArea (const double ma) |
Set the cross sectional area A of the beam (m^2) | |
double | GetArea () const |
void | SetDensity (double md) |
Set the density of the beam (kg/m^3) | |
double | GetDensity () const |
Additional Inherited Members | |
![]() | |
double | y_drawsize |
double | z_drawsize |
bool | is_circular |
double | Area |
double | density |
Constructor & Destructor Documentation
◆ ChBeamSectionCosserat() [1/3]
chrono::fea::ChBeamSectionCosserat::ChBeamSectionCosserat | ( | std::shared_ptr< ChElasticityCosserat > | melasticity | ) |
- Parameters
-
melasticity elasticity model for this section
◆ ChBeamSectionCosserat() [2/3]
chrono::fea::ChBeamSectionCosserat::ChBeamSectionCosserat | ( | std::shared_ptr< ChElasticityCosserat > | melasticity, |
std::shared_ptr< ChPlasticityCosserat > | mplasticity | ||
) |
- Parameters
-
melasticity elasticity model for this section mplasticity plasticity model for this section, if any
◆ ChBeamSectionCosserat() [3/3]
chrono::fea::ChBeamSectionCosserat::ChBeamSectionCosserat | ( | std::shared_ptr< ChElasticityCosserat > | melasticity, |
std::shared_ptr< ChPlasticityCosserat > | mplasticity, | ||
std::shared_ptr< ChDampingCosserat > | mdamping | ||
) |
- Parameters
-
melasticity elasticity model for this section mplasticity plasticity model for this section, if any mdamping damping model for this section, if any
Member Function Documentation
◆ ComputeStiffnessMatrix()
|
virtual |
Compute the 6x6 tangent material stiffness matrix [Km] = dσ/dε Compute the 6x6 tangent material stiffness matrix [Km] = dσ/dε at a given strain state, and at given internal data state (if mdata=nullptr, computes only the elastic tangent stiffenss, regardless of plasticity).
- Parameters
-
K 6x6 stiffness matrix strain_e strain (deformation part): x= elongation, y and z are shear strain_k strain (curvature part), x= torsion, y and z are line curvatures mdata material internal variables, at this point, if any, including {p_strain_e, p_strain_k, p_strain_acc}
◆ ComputeStress()
|
virtual |
Compute the generalized cut force and cut torque, given the actual generalized section strain expressed as deformation vector e and curvature k, that is: {F,M}=f({e,k}), and given the actual material state required for plasticity if any (but if mdata=nullptr, computes only the elastic force).
If there is plasticity, the stress is clamped by automatically performing an implicit return mapping. In sake of generality, if possible this is the function that should be used by beam finite elements to compute internal forces, ex.by some Gauss quadrature.
- Parameters
-
stress_n stress (generalized force F), x component = traction along beam stress_m stress (generalized torque M), x component = torsion torque along beam strain_e strain (deformation part e): x= elongation, y and z are shear strain_k strain (curvature part k), x= torsion, y and z are line curvatures mdata_new updated material internal variables, at this point, including {p_strain_e, p_strain_k, p_strain_acc} mdata current material internal variables, at this point, including {p_strain_e, p_strain_k, p_strain_acc}
◆ GetDamping()
|
inline |
Get the damping model for this section.
By default no damping.
◆ GetElasticity()
|
inline |
Get the elasticity model for this section.
Use this function to access parameters such as stiffness, Young modulus, etc. By default it uses a simple centered linear elastic model.
◆ GetPlasticity()
|
inline |
Get the elasticity model for this section, if any.
Use this function to access parameters such as yeld limit, etc.
◆ SetDamping()
void chrono::fea::ChBeamSectionCosserat::SetDamping | ( | std::shared_ptr< ChDampingCosserat > | mdamping | ) |
Set the damping model for this section.
By default no damping.
◆ SetElasticity()
void chrono::fea::ChBeamSectionCosserat::SetElasticity | ( | std::shared_ptr< ChElasticityCosserat > | melasticity | ) |
Set the elasticity model for this section.
By default it uses a simple centered linear elastic model, but you can set more complex models.
◆ SetPlasticity()
void chrono::fea::ChBeamSectionCosserat::SetPlasticity | ( | std::shared_ptr< ChPlasticityCosserat > | mplasticity | ) |
Set the plasticity model for this section.
This is independent from the elasticity model. Note that by default there is no plasticity model, so by default plasticity never happens.
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono/fea/ChBeamSectionCosserat.h
- /builds/uwsbel/chrono/src/chrono/fea/ChBeamSectionCosserat.cpp