Description
Interface base class for scalar functions of the type: y= f(x)
The ChFunction class defines the base class for all Chrono functions of type y=f(x), that is scalar functions of an input variable x (usually, the time). ChFunctions are often used to set time-dependent properties, for example to set motion laws in linear actuators, engines, etc. This base class just represent a constant function of the type y= C. Inherited classes must override at least the Get_y() method, in order to represent more complex functions.
#include <ChFunction_Base.h>
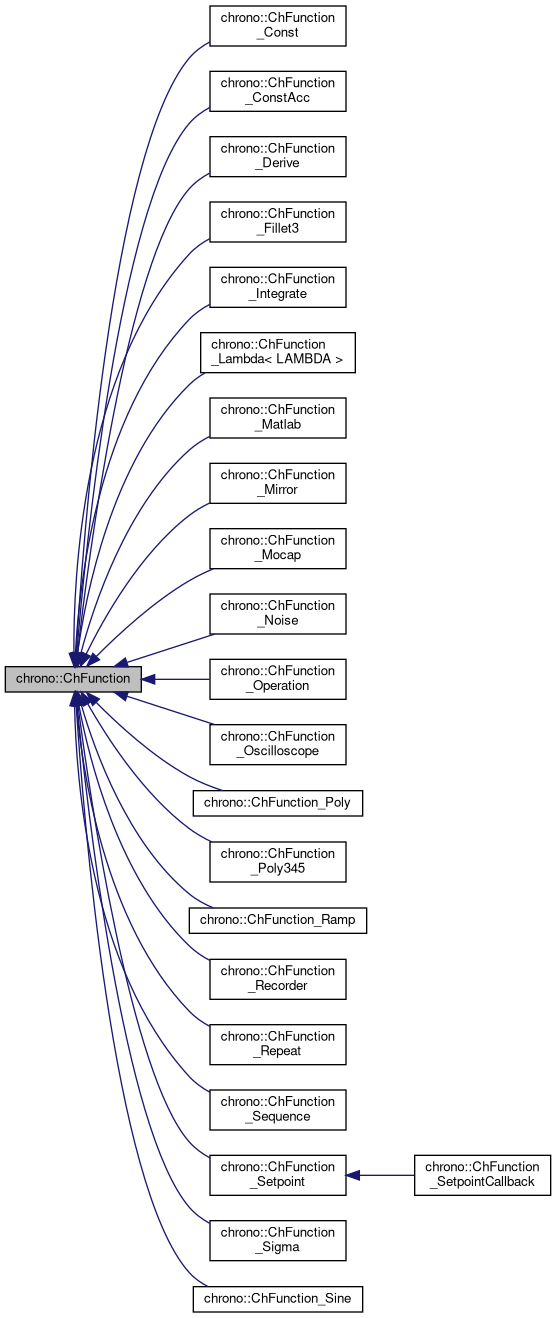
Public Types | |
enum | FunctionType { FUNCT_CUSTOM, FUNCT_CONST, FUNCT_CONSTACC, FUNCT_DERIVE, FUNCT_FILLET3, FUNCT_INTEGRATE, FUNCT_MATLAB, FUNCT_MIRROR, FUNCT_MOCAP, FUNCT_NOISE, FUNCT_OPERATION, FUNCT_OSCILLOSCOPE, FUNCT_POLY, FUNCT_POLY345, FUNCT_RAMP, FUNCT_RECORDER, FUNCT_REPEAT, FUNCT_SEQUENCE, FUNCT_SIGMA, FUNCT_SINE, FUNCT_LAMBDA } |
Enumeration of function types. | |
Public Member Functions | |
ChFunction (const ChFunction &other) | |
virtual ChFunction * | Clone () const =0 |
"Virtual" copy constructor. | |
virtual FunctionType | Get_Type () const |
Return the unique function type identifier. | |
virtual double | Get_y (double x) const =0 |
Return the y value of the function, at position x. | |
virtual double | Get_y_dx (double x) const |
Return the dy/dx derivative of the function, at position x. More... | |
virtual double | Get_y_dxdx (double x) const |
Return the ddy/dxdx double derivative of the function, at position x. More... | |
virtual double | Get_weight (double x) const |
Return the weight of the function (useful for applications where you need to mix different weighted ChFunctions) | |
virtual void | Estimate_x_range (double &xmin, double &xmax) const |
Return an estimate of the range of the function argument. More... | |
virtual void | Estimate_y_range (double xmin, double xmax, double &ymin, double &ymax, int derivate) const |
Return an estimate of the range of the function value. More... | |
virtual double | Get_y_dN (double x, int derivate) const |
Return the function derivative of specified order at the given point. More... | |
virtual void | Update (const double x) |
Update could be implemented by children classes, ex. to launch callbacks. | |
virtual double | Compute_max (double xmin, double xmax, double sampling_step, int derivate) const |
Compute the maximum of y(x) in a range xmin-xmax, using a sampling method. | |
virtual double | Compute_min (double xmin, double xmax, double sampling_step, int derivate) const |
Compute the minimum of y(x) in a range xmin-xmax, using a sampling method. | |
virtual double | Compute_mean (double xmin, double xmax, double sampling_step, int derivate) const |
Compute the mean value of y(x) in a range xmin-xmax, using a sampling method. | |
virtual double | Compute_sqrmean (double xmin, double xmax, double sampling_step, int derivate) const |
Compute the square mean val. of y(x) in a range xmin-xmax, using sampling. | |
virtual double | Compute_int (double xmin, double xmax, double sampling_step, int derivate) const |
Compute the integral of y(x) in a range xmin-xmax, using a sampling method. | |
virtual double | Get_Ca_pos () const |
Computes the positive acceleration coefficient (inherited classes should customize this). | |
virtual double | Get_Ca_neg () const |
Compute the positive acceleration coefficient (inherited classes should customize this). | |
virtual double | Get_Cv () const |
Compute the speed coefficient (inherited classes must customize this). | |
virtual int | HandleNumber () const |
Return the number of handles of the function. | |
virtual bool | HandleAccess (int handle_id, double mx, double my, bool set_mode) |
Get the x and y position of handle, given identifier. More... | |
virtual void | ArchiveOUT (ChArchiveOut &marchive) |
Method to allow serialization of transient data to archives. | |
virtual void | ArchiveIN (ChArchiveIn &marchive) |
Method to allow de-serialization of transient data from archives. More... | |
virtual int | FilePostscriptPlot (ChFile_ps *m_file, int plotY, int plotDY, int plotDDY) |
Plot function in graph space of the ChFile_ps postscript file where zoom factor, centering, color, thickness etc. More... | |
virtual int | FileAsciiPairsSave (ChStreamOutAscii &m_file, double xmin=0, double xmax=1, int msamples=200) |
Save function as X-Y pairs separated by space, with CR at each pair, into an ASCII file. More... | |
Member Function Documentation
◆ ArchiveIN()
|
virtual |
Method to allow de-serialization of transient data from archives.
Method to allow de serialization of transient data from archives.
Reimplemented in chrono::ChFunction_Sequence, chrono::ChFunction_ConstAcc, chrono::ChFunction_Fillet3, chrono::ChFunction_Oscilloscope, chrono::ChFunction_Integrate, chrono::ChFunction_Setpoint, chrono::ChFunction_Recorder, chrono::ChFunction_Mocap, chrono::ChFunction_Operation, chrono::ChFunction_Poly, chrono::ChFunction_Repeat, chrono::ChFunction_Poly345, chrono::ChFunction_Sine, chrono::ChFunction_Sigma, chrono::ChFunction_Noise, chrono::ChFunction_Derive, chrono::ChFunction_Ramp, chrono::ChFunction_Mirror, chrono::ChFunction_Const, and chrono::ChFunction_Matlab.
◆ Estimate_x_range()
|
inlinevirtual |
Return an estimate of the range of the function argument.
(Can be used for automatic zooming in a GUI)
Reimplemented in chrono::ChFunction_Sequence, chrono::ChFunction_ConstAcc, chrono::ChFunction_Fillet3, chrono::ChFunction_Oscilloscope, chrono::ChFunction_Integrate, chrono::ChFunction_Recorder, chrono::ChFunction_Mocap, chrono::ChFunction_Operation, chrono::ChFunction_Repeat, chrono::ChFunction_Poly345, chrono::ChFunction_Sigma, chrono::ChFunction_Derive, and chrono::ChFunction_Mirror.
◆ Estimate_y_range()
|
virtual |
Return an estimate of the range of the function value.
(Can be used for automatic zooming in a GUI)
◆ FileAsciiPairsSave()
|
virtual |
Save function as X-Y pairs separated by space, with CR at each pair, into an ASCII file.
The output file can be later loaded into Excel, GnuPlot or other tools. The function is 'sampled' for nsteps times, from xmin to xmax.
◆ FilePostscriptPlot()
|
virtual |
Plot function in graph space of the ChFile_ps postscript file where zoom factor, centering, color, thickness etc.
are already defined. If plotDY=true, plots also the derivative, etc.
◆ Get_y_dN()
|
virtual |
Return the function derivative of specified order at the given point.
Note that only order = 0, 1, or 2 is supported.
◆ Get_y_dx()
|
inlinevirtual |
Return the dy/dx derivative of the function, at position x.
Note that inherited classes may also avoid overriding this method, because this base method already provide a general-purpose numerical differentiation to get dy/dx only from the Get_y() function. (however, if the analytical derivative is known, it may better to implement a custom method).
Reimplemented in chrono::ChFunction_Sequence, chrono::ChFunction_Recorder, chrono::ChFunction_Setpoint, chrono::ChFunction_Lambda< LAMBDA >, chrono::ChFunction_Fillet3, chrono::ChFunction_ConstAcc, chrono::ChFunction_Mocap, chrono::ChFunction_Sine, chrono::ChFunction_Poly345, chrono::ChFunction_Sigma, chrono::ChFunction_Const, chrono::ChFunction_Ramp, chrono::ChFunction_Poly, and chrono::ChFunction_Matlab.
◆ Get_y_dxdx()
|
inlinevirtual |
Return the ddy/dxdx double derivative of the function, at position x.
Note that inherited classes may also avoid overriding this method, because this base method already provide a general-purpose numerical differentiation to get ddy/dxdx only from the Get_y() function. (however, if the analytical derivative is known, it may be better to implement a custom method).
Reimplemented in chrono::ChFunction_Sequence, chrono::ChFunction_Recorder, chrono::ChFunction_Setpoint, chrono::ChFunction_Lambda< LAMBDA >, chrono::ChFunction_Fillet3, chrono::ChFunction_ConstAcc, chrono::ChFunction_Mocap, chrono::ChFunction_Sine, chrono::ChFunction_Poly345, chrono::ChFunction_Sigma, chrono::ChFunction_Const, chrono::ChFunction_Ramp, chrono::ChFunction_Poly, and chrono::ChFunction_Matlab.
◆ HandleAccess()
|
inlinevirtual |
Get the x and y position of handle, given identifier.
If set mode, x and y values are stored. Return false if handle not found.
Reimplemented in chrono::ChFunction_Sequence.
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono/motion_functions/ChFunction_Base.h
- /builds/uwsbel/chrono/src/chrono/motion_functions/ChFunction_Base.cpp