Description
Wrapper class for ChWheeledVehicles.
Functions here are related to initializing this as a zombie (setting visual representations, # of wheels)
#include <SynWheeledVehicle.h>
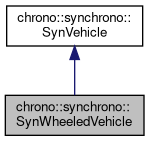
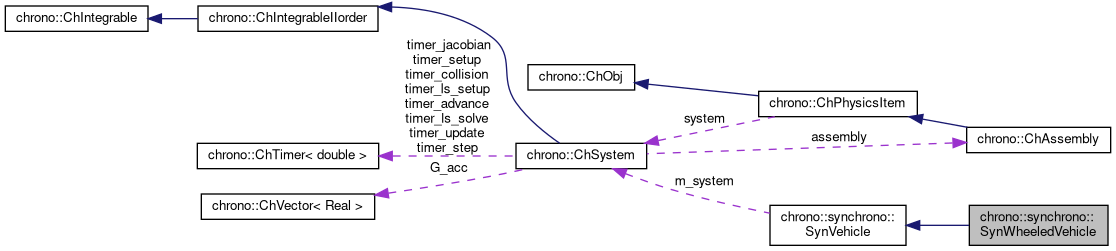
Public Member Functions | |
SynWheeledVehicle () | |
Default constructor for a SynWheeledVehicle. | |
SynWheeledVehicle (vehicle::ChWheeledVehicle *wheeled_vehicle) | |
Constructor for a SynWheeledVehicle where a vehicle pointer is passed In this case, the system is assumed to have been created by the vehicle, so the system will subsequently not be advanced or destroyed. More... | |
SynWheeledVehicle (const ChCoordsys<> &coord_sys, const std::string &filename, ChContactMethod contact_method) | |
Constructor for a wheeled vehicle specified through json and a contact method This constructor will create it's own system. More... | |
SynWheeledVehicle (const ChCoordsys<> &coord_sys, const std::string &filename, ChSystem *system) | |
Constructor for a wheeled vehicle specified through json and a contact method This vehicle will use the passed system and not create its own. More... | |
SynWheeledVehicle (const std::string &filename) | |
Construct a zombie SynWheeledVehicle from a json specification file. More... | |
virtual | ~SynWheeledVehicle () |
Destructor for a SynWheeledVehicle. | |
virtual void | InitializeZombie (ChSystem *system) override |
Initialize the zombie representation of the vehicle. More... | |
virtual void | SynchronizeZombie (SynMessage *message) override |
Synchronize the position and orientation of this vehicle with other ranks. More... | |
virtual void | Update () override |
Update the current state of this vehicle. | |
void | SetZombieVisualizationFiles (std::string chassis_vis_file, std::string wheel_vis_file, std::string tire_vis_file) |
Set the zombie visualization files. More... | |
void | SetNumWheels (int num_wheels) |
Set the number of wheels of the underlying vehicle. More... | |
void | Synchronize (double time, const vehicle::ChDriver::Inputs &driver_inputs, const vehicle::ChTerrain &terrain) |
Update the state of this vehicle at the current time. More... | |
virtual vehicle::ChVehicle & | GetVehicle () override |
Get the underlying vehicle. More... | |
![]() | |
SynVehicle (bool is_zombie=true) | |
Default constructor for a SynVehicle. More... | |
SynVehicle (const std::string &filename, ChContactMethod contact_method) | |
Constructor for vehicles specified through json and a contact method This constructor will create it's own system. More... | |
SynVehicle (const std::string &filename, ChSystem *system) | |
Constructor for vehicles specified through json and a contact method This vehicle will use the passed system and not create its own. More... | |
SynVehicle (const std::string &filename) | |
Constructor for zombie vehicles specified through json. More... | |
virtual | ~SynVehicle () |
Destroy the SynVehicle object. | |
bool | IsZombie () |
Get whether this vehicle is a zombie. More... | |
virtual void | Advance (double step) |
Advance the state of this vehicle by the time amount specified If the system is not owned by this vehicle, this method should be overriden. More... | |
ChSystem * | GetSystem () |
Get the ChSystem. More... | |
Protected Member Functions | |
virtual rapidjson::Document | ParseVehicleFileJSON (const std::string &filename) override |
Parse a JSON specification file that describes a vehicle. More... | |
virtual void | CreateVehicle (const ChCoordsys<> &coord_sys, const std::string &filename, ChSystem *system) override |
Helper method for creating a vehicle from a json specification file. More... | |
![]() | |
virtual void | CreateZombie (const std::string &filename) |
Construct a zombie from a json specification file. More... | |
std::shared_ptr< ChTriangleMeshShape > | CreateMeshZombieComponent (const std::string &filename) |
Helper method used to create a ChTriangleMeshShape to be used on as a zombie body. More... | |
void | CreateChassisZombieBody (const std::string &filename, ChSystem *system) |
Create a zombie chassis body. More... | |
Friends | |
class | SynWheeledVehicleAgent |
Additional Inherited Members | |
![]() | |
bool | m_is_zombie |
is this vehicle a zombie | |
bool | m_owns_vehicle |
has the vehicle been created by this object or passed by user | |
ChSystem * | m_system |
pointer to the chrono system | |
std::shared_ptr< ChBodyAuxRef > | m_zombie_body |
agent's zombie body reference | |
Constructor & Destructor Documentation
◆ SynWheeledVehicle() [1/4]
chrono::synchrono::SynWheeledVehicle::SynWheeledVehicle | ( | vehicle::ChWheeledVehicle * | wheeled_vehicle | ) |
Constructor for a SynWheeledVehicle where a vehicle pointer is passed In this case, the system is assumed to have been created by the vehicle, so the system will subsequently not be advanced or destroyed.
- Parameters
-
wheeled_vehicle the vehicle to wrap
◆ SynWheeledVehicle() [2/4]
chrono::synchrono::SynWheeledVehicle::SynWheeledVehicle | ( | const ChCoordsys<> & | coord_sys, |
const std::string & | filename, | ||
ChContactMethod | contact_method | ||
) |
Constructor for a wheeled vehicle specified through json and a contact method This constructor will create it's own system.
- Parameters
-
coord_sys the initial position and orientation of the vehicle filename the json specification file to build the vehicle from contact_method the contact method used for the Chrono dynamics
◆ SynWheeledVehicle() [3/4]
chrono::synchrono::SynWheeledVehicle::SynWheeledVehicle | ( | const ChCoordsys<> & | coord_sys, |
const std::string & | filename, | ||
ChSystem * | system | ||
) |
Constructor for a wheeled vehicle specified through json and a contact method This vehicle will use the passed system and not create its own.
- Parameters
-
coord_sys the initial position and orientation of the vehicle filename the json specification file to build the vehicle from system the system to be used for Chrono dynamics and to add bodies to
◆ SynWheeledVehicle() [4/4]
chrono::synchrono::SynWheeledVehicle::SynWheeledVehicle | ( | const std::string & | filename | ) |
Construct a zombie SynWheeledVehicle from a json specification file.
- Parameters
-
filename the json specification file to build the zombie from
Member Function Documentation
◆ CreateVehicle()
|
overrideprotectedvirtual |
Helper method for creating a vehicle from a json specification file.
- Parameters
-
coord_sys the initial position and orientation of the vehicle filename the json specification file system a ChSystem to be used when constructing a vehicle
Implements chrono::synchrono::SynVehicle.
◆ GetVehicle()
|
inlineoverridevirtual |
◆ InitializeZombie()
|
overridevirtual |
Initialize the zombie representation of the vehicle.
Will create and add bodies that are visual representation of the vehicle. The position and orientation is updated by SynChrono and the passed messages
- Parameters
-
system the system used to add bodies to for consistent visualization
Implements chrono::synchrono::SynVehicle.
◆ ParseVehicleFileJSON()
|
overrideprotectedvirtual |
Parse a JSON specification file that describes a vehicle.
- Parameters
-
filename the json specification file
Reimplemented from chrono::synchrono::SynVehicle.
◆ SetNumWheels()
|
inline |
Set the number of wheels of the underlying vehicle.
- Parameters
-
num_wheels number of wheels of the underlying vehicle
◆ SetZombieVisualizationFiles()
void chrono::synchrono::SynWheeledVehicle::SetZombieVisualizationFiles | ( | std::string | chassis_vis_file, |
std::string | wheel_vis_file, | ||
std::string | tire_vis_file | ||
) |
Set the zombie visualization files.
- Parameters
-
chassis_vis_file the file used for chassis visualization wheel_vis_file the file used for wheel visualization tire_vis_file the file used for tire visualization
◆ Synchronize()
void chrono::synchrono::SynWheeledVehicle::Synchronize | ( | double | time, |
const vehicle::ChDriver::Inputs & | driver_inputs, | ||
const vehicle::ChTerrain & | terrain | ||
) |
Update the state of this vehicle at the current time.
- Parameters
-
time the time to synchronize to driver_inputs the driver inputs (i.e. throttle, braking, steering) terrain reference to the terrain the vehicle should contact
◆ SynchronizeZombie()
|
overridevirtual |
Synchronize the position and orientation of this vehicle with other ranks.
Any message can be passed, so a check should be done to ensure this message was intended for this agent
- Parameters
-
message the received message that describes the position and orientation
Implements chrono::synchrono::SynVehicle.
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono_synchrono/vehicle/SynWheeledVehicle.h
- /builds/uwsbel/chrono/src/chrono_synchrono/vehicle/SynWheeledVehicle.cpp