chrono::synchrono::SynMPIManager Class Reference
Description
Concrete class using MPI AllGatherV calls to manage state synchronization.
#include <SynMPIManager.h>
Inheritance diagram for chrono::synchrono::SynMPIManager:
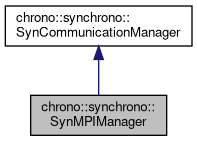
Collaboration diagram for chrono::synchrono::SynMPIManager:
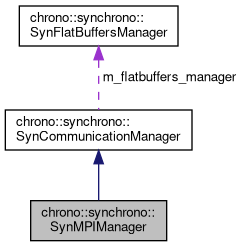
Public Member Functions | |
SynMPIManager (int argc, char *argv[], SynMPIConfig config=MPI_CONFIG_DEFAULT) | |
virtual void | Exit () |
virtual bool | Initialize () override |
Generate agent description messages and synchronize them to all agents. More... | |
virtual void | Synchronize () override |
Gather messages from all ranks and synchronize the simulation. | |
virtual void | Update () |
Update the zombie agents and process messages. More... | |
virtual void | Barrier () override |
Wrapper of MPI barrier. | |
SynMPIConfig & | GetConfig () |
Get the MPI Config. | |
![]() | |
SynCommunicationManager () | |
Constructs a new SynCommunicationManager object. | |
SynCommunicationManager (int msg_length) | |
Constructs a new SynCommunicationManager object with a starting message length. | |
~SynCommunicationManager () | |
Destory the SynCommunicationManager object. | |
virtual bool | AddAgent (std::shared_ptr< SynAgent > agent) |
Add the specified agent to this manager's list of agents. More... | |
virtual bool | AddAgent (std::shared_ptr< SynAgent > agent, int rank) |
Add the specified agent to this manager's list of agents at the provided rank. More... | |
virtual void | Advance (double time_to_next_sync) |
Advance the state of the agent simulated by this rank to the specified timestep. More... | |
virtual void | GenerateMessages () final |
Adds all messages to the flatbuffer manager and finishes it size-prefixed (if desired) | |
virtual void | GenerateAgentDescriptionMessage () final |
Adds all info messages to the flatbuffer manager and finishes it size-prefixed. | |
virtual void | ProcessMessage (const uint8_t *data) |
Processes all of the messages received. More... | |
virtual void | ProcessMessageBuffer (const SynFlatBuffers::Buffer *buffer) final |
Processes all messages inside a single buffer. More... | |
virtual std::shared_ptr< SynAgent > | AgentFromDescription (const SynFlatBuffers::Buffer *buffer) final |
Processes all messages inside a single buffer. More... | |
std::shared_ptr< SynAgent > | GetAgent (int rank) |
Get agent at specified rank. | |
virtual int | GetRank () final |
Get rank of current manager. | |
virtual int | GetNumRanks () final |
Get the number of ranks in the simulation. | |
virtual std::map< int, std::shared_ptr< SynAgent > > | GetAgentList () final |
Get the agent list. | |
bool | IsOk () |
Is the simulation still running? | |
double | GetHeartbeat () |
Get/Set the heartbeat. | |
void | SetHeartbeat (double heartbeat) |
double | GetEndTime () |
Get/Set the end time. | |
void | SetEndTime (double end_time) |
Protected Attributes | |
SynMPIConfig | m_config |
int | m_msg_length |
Maximum size of message that can be sent from any agent. | |
int | m_bcast_length |
Size of the buffer that gets broadcast to all agents (num_ranks - 1) * m_msg_length. | |
int | m_total_length |
Current length of messages from all ranks. | |
int * | m_msg_lengths |
Array of message lengths for all ranks. | |
int * | m_msg_displs |
Array of message displacements for all ranks. | |
std::vector< uint8_t > | m_rank_data |
std::vector< uint8_t > | m_all_data |
Buffer for receiving messages from all ranks. | |
![]() | |
bool | m_ok |
Is everything ok? | |
double | m_heartbeat |
double | m_end_time |
int | m_num_advances |
keeps track of the number of updates that have occured so far | |
bool | m_is_synchronized |
keeps track of the whether ranks are time synchronized | |
int | m_rank |
rank which this manager controls | |
int | m_num_ranks |
total number of ranks in this simulation | |
bool | m_initialized |
has the manager been initialized | |
std::shared_ptr< SynAgent > | m_agent |
handle to this rank's agent | |
std::map< int, std::shared_ptr< SynAgent > > | m_agent_list |
id to agent map on this rank | |
std::map< int, std::vector< SynMessage * > > | m_message_list |
received messages mapped to each rank | |
SynFlatBuffersManager | m_flatbuffers_manager |
flatbuffer manager for this rank | |
Member Function Documentation
◆ Initialize()
|
overridevirtual |
Generate agent description messages and synchronize them to all agents.
- Returns
- boolean indicated whether initialization function was successful
Reimplemented from chrono::synchrono::SynCommunicationManager.
◆ Update()
|
virtual |
Update the zombie agents and process messages.
Overriding classes should probably at a minimum call ProcessBufferedMessages. All worlds should be guaranteed to be synchronized after this function returns.
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono_synchrono/communication/mpi/SynMPIManager.h
- /builds/uwsbel/chrono/src/chrono_synchrono/communication/mpi/SynMPIManager.cpp