Description
Geometric object representing an sequence of other ChLine objects, The ChLine objects are assumed to be properly concatenated and to have C0 continuity.
#include <ChLinePath.h>
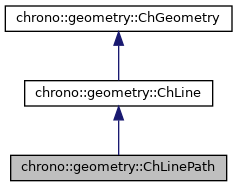
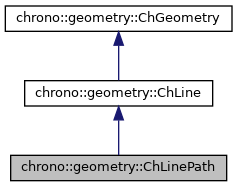
Public Member Functions | |
ChLinePath (const ChLinePath &source) | |
virtual ChLinePath * | Clone () const override |
"Virtual" copy constructor (covariant return type). | |
virtual Type | GetClassType () const override |
Get the class type as an enum. | |
virtual int | Get_complexity () const override |
Tell the complexity. | |
virtual double | Length (int sampling) const override |
Return curve length. More... | |
virtual ChVector | Evaluate (double U) const override |
Return a point on the line, given parametric coordinate U (in [0,1]). | |
virtual ChVector | GetEndA () const override |
Return the start point of the line. | |
virtual ChVector | GetEndB () const override |
Return the end point of the line. | |
size_t | GetSubLinesCount () const |
Get count of sub-lines that have been added. | |
std::shared_ptr< ChLine > | GetSubLineN (size_t n) const |
Access the nth sub-line. | |
double | GetSubLineDurationN (size_t n) const |
Get the nth sub-line duration. | |
void | SetSubLineDurationN (size_t n, double mduration) |
Set the nth sub-line duration. | |
void | AddSubLine (std::shared_ptr< ChLine > mline, double duration=1) |
Queue a line (push it back to the array of lines). More... | |
void | AddSubLine (ChLine &mline, double duration=1) |
Queue a line (push it back to the array of lines) More... | |
void | InsertSubLine (size_t n, std::shared_ptr< ChLine > mline, double duration=1) |
Insert a line at the specified index in line array. More... | |
void | InsertSubLine (size_t n, ChLine &mline, double duration=1) |
Insert a line at a specified index n in line array. More... | |
void | EraseSubLine (size_t n) |
Erase the line at the specified index n in line array. More... | |
double | GetPathDuration () const |
Tells the duration of the path, sum of the durations of all sub-lines. More... | |
void | SetPathDuration (double mUduration) |
Shrink or stretch all the durations of the sub-lines so that the total duration of the path is equal to a specified value. More... | |
double | GetContinuityMaxError () const |
Check if the path is topologically connected, i.e. More... | |
virtual void | ArchiveOut (ChArchiveOut &marchive) override |
Method to allow serialization of transient data to archives. | |
virtual void | ArchiveIn (ChArchiveIn &marchive) override |
Method to allow de-serialization of transient data from archives. | |
![]() | |
ChLine (const ChLine &source) | |
virtual ChVector | GetTangent (double parU) const |
Return the tangent unit vector at the parametric coordinate U (in [0,1]). More... | |
virtual bool | Get_closed () const |
Tell if the curve is closed. | |
virtual void | Set_closed (bool mc) |
virtual void | Set_complexity (int mc) |
virtual int | GetManifoldDimension () const override |
This is a line. | |
bool | FindNearestLinePoint (ChVector<> &point, double &resU, double approxU, double tol) const |
Find the parameter resU for the nearest point on curve to "point". | |
double | CurveCurveDist (ChLine *compline, int samples) const |
Returns adimensional information on "how much" this curve is similar to another in its overall shape (does not matter parametrization or start point). More... | |
double | CurveSegmentDist (ChLine *complinesegm, int samples) const |
Same as before, but returns "how near" is complinesegm to whatever segment of this line (does not matter the percentual of line). More... | |
double | CurveCurveDistMax (ChLine *compline, int samples) const |
Same as above, but instead of making average of the distances, these functions return the maximum of the distances... | |
double | CurveSegmentDistMax (ChLine *complinesegm, int samples) const |
virtual bool | DrawPostscript (ChFile_ps *mfle, int markpoints, int bezier_interpolate) |
Draw into the current graph viewport of a ChFile_ps file. | |
![]() | |
ChGeometry (const ChGeometry &source) | |
virtual ChAABB | GetBoundingBox () const |
Compute bounding box along the directions of the shape definition frame. More... | |
void | InflateBoundingBox (ChAABB &bbox) const |
Enlarge the given existing bounding box with the bounding box of this object. | |
virtual double | GetBoundingSphereRadius () const |
Returns the radius of a bounding sphere for this geometry. More... | |
virtual ChVector | Baricenter () const |
Compute center of mass. | |
virtual void | Update () |
Generic update of internal data. | |
Public Attributes | |
std::vector< std::shared_ptr< ChLine > > | lines |
std::vector< double > | end_times |
std::vector< double > | durations |
Additional Inherited Members | |
![]() | |
enum | Type { NONE, SPHERE, ELLIPSOID, BOX, CYLINDER, TRIANGLE, CAPSULE, CONE, LINE, LINE_ARC, LINE_BEZIER, LINE_CAM, LINE_PATH, LINE_POLY, LINE_SEGMENT, ROUNDED_BOX, ROUNDED_CYLINDER, TRIANGLEMESH, TRIANGLEMESH_CONNECTED, TRIANGLEMESH_SOUP } |
Enumeration of geometric object types. | |
![]() | |
bool | closed |
int | complexityU |
Member Function Documentation
◆ AddSubLine() [1/2]
void chrono::geometry::ChLinePath::AddSubLine | ( | ChLine & | mline, |
double | duration = 1 |
||
) |
Queue a line (push it back to the array of lines)
- Parameters
-
mline line to add duration duration of the abscyssa when calling the Evaluate() function
◆ AddSubLine() [2/2]
void chrono::geometry::ChLinePath::AddSubLine | ( | std::shared_ptr< ChLine > | mline, |
double | duration = 1 |
||
) |
Queue a line (push it back to the array of lines).
- Parameters
-
mline line to add duration duration of the abscyssa when calling the Evaluate() function
◆ EraseSubLine()
void chrono::geometry::ChLinePath::EraseSubLine | ( | size_t | n | ) |
Erase the line at the specified index n in line array.
Note that n cannot be higher than GetLineCount().
◆ GetContinuityMaxError()
double chrono::geometry::ChLinePath::GetContinuityMaxError | ( | ) | const |
Check if the path is topologically connected, i.e.
if all the sub lines are queued to have C0 continuity
◆ GetPathDuration()
double chrono::geometry::ChLinePath::GetPathDuration | ( | ) | const |
Tells the duration of the path, sum of the durations of all sub-lines.
This is useful because ifyou use the Evaluate() function on the path, the U parameter should range between 0 and the max duration.
◆ InsertSubLine() [1/2]
void chrono::geometry::ChLinePath::InsertSubLine | ( | size_t | n, |
ChLine & | mline, | ||
double | duration = 1 |
||
) |
Insert a line at a specified index n in line array.
Note that n cannot be higher than GetLineCount().
- Parameters
-
n index of line, 0 is first, etc. mline line to add duration duration of the abscyssa when calling the Evaluate() function
◆ InsertSubLine() [2/2]
void chrono::geometry::ChLinePath::InsertSubLine | ( | size_t | n, |
std::shared_ptr< ChLine > | mline, | ||
double | duration = 1 |
||
) |
Insert a line at the specified index in line array.
- Parameters
-
n index in line array mline line to add duration duration of the abscyssa when calling the Evaluate() function
◆ Length()
|
overridevirtual |
◆ SetPathDuration()
void chrono::geometry::ChLinePath::SetPathDuration | ( | double | mUduration | ) |
Shrink or stretch all the durations of the sub-lines so that the total duration of the path is equal to a specified value.
For example, you can normalize to 1 so you can use Evaluate() with U in the 0..1 range like with other lines.
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono/geometry/ChLinePath.h
- /builds/uwsbel/chrono/src/chrono/geometry/ChLinePath.cpp