Description
Base class for representing constraints (bilateral or unilateral).
These constraints are used with variational inequality or DAE solvers for problems including equalities, inequalities, nonlinearities, etc.
See ChSystemDescriptor for more information about the overall problem and data representation.
The Jacobian matrix [Cq] is built row by row from individual constraint Jacobians [Cq_i]. [E] optionally includes 'cfm_i' terms on the diagonal.
In general, typical bilateral constraints must be solved to have residual c_i = 0 and unilaterals to have c_i>0, where the following linearization is introduced:
c_i= [Cq_i]*q + b_i
The base class introduces just the minimum requirements for the solver, that is the basic methods that will be called by the solver.
#include <ChConstraint.h>
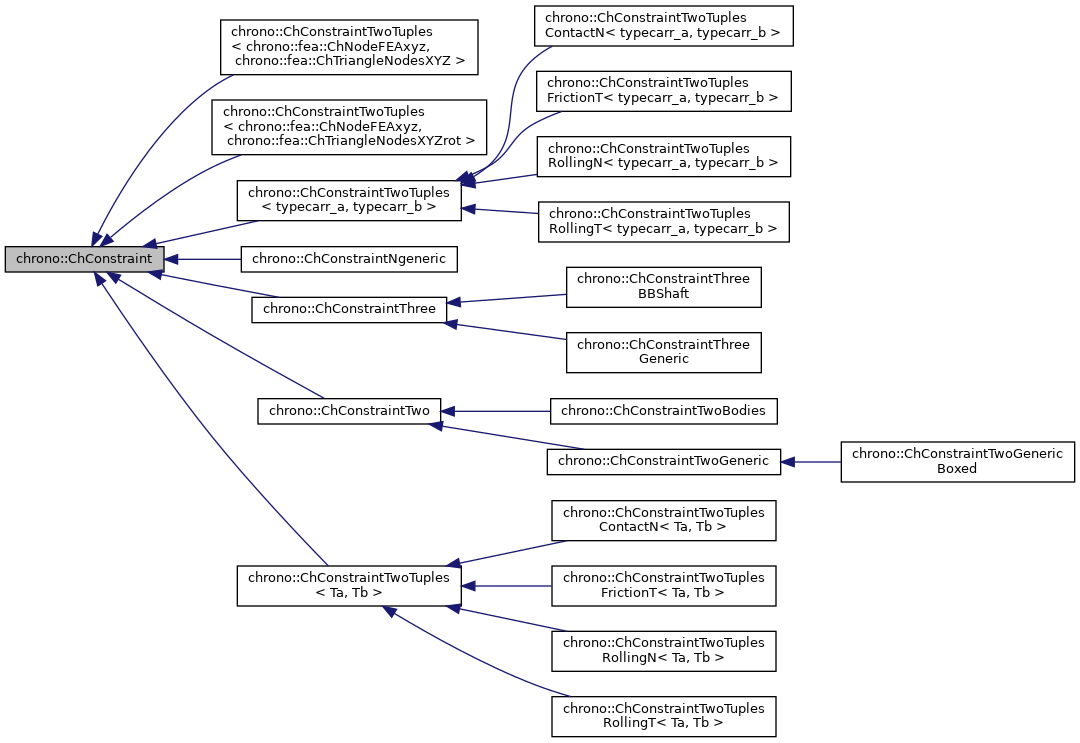
Public Types | |
enum | Mode { Mode::FREE, Mode::LOCK, Mode::UNILATERAL, Mode::FRICTION } |
Constraint mode. More... | |
Public Member Functions | |
ChConstraint (const ChConstraint &other) | |
virtual ChConstraint * | Clone () const =0 |
"Virtual" copy constructor. | |
ChConstraint & | operator= (const ChConstraint &other) |
Assignment operator: copy from other object. | |
bool | operator== (const ChConstraint &other) const |
Comparison (compares only flags, not the Jacobians). | |
bool | IsValid () const |
Indicate if the constraint data is currently valid. | |
void | SetValid (bool mon) |
Set the "valid" state of this constraint. | |
bool | IsDisabled () const |
Indicate if the constraint is currently turned on or off. | |
void | SetDisabled (bool mon) |
bool | IsRedundant () const |
Indicate if the constraint is redundant or singular. | |
void | SetRedundant (bool mon) |
Mark the constraint as redundant. | |
bool | IsBroken () const |
Indicate if the constraint is broken, due to excess pulling/pushing. | |
void | SetBroken (bool mon) |
Set the constraint as broken. More... | |
virtual bool | IsUnilateral () const |
Indicate if the constraint is unilateral (typical complementarity constraint). | |
virtual bool | IsLinear () const |
Indicate if the constraint is linear. | |
Mode | GetMode () const |
Get the mode of the constraint. More... | |
void | SetMode (Mode mmode) |
Set the mode of the constraint. | |
bool | IsActive () const |
Indicate whether the constraint is currently active. More... | |
void | SetActive (bool isactive) |
Set the status of the constraint to active. | |
virtual double | ComputeResidual () |
Compute the residual of the constraint using the linear expression. More... | |
double | GetResidual () const |
Return the residual of this constraint. | |
void | SetRightHandSide (const double mb) |
Sets the known term b_i in [Cq_i]*q + b_i = 0, where: c_i = [Cq_i]*q + b_i = 0. | |
double | GetRightHandSide () const |
Return the known term b_i in [Cq_i]*q + b_i = 0, where: c_i= [Cq_i]*q + b_i = 0. | |
void | SetComplianceTerm (const double mcfm) |
Set the constraint force mixing term (default=0). More... | |
double | GetComplianceTerm () const |
Return the constraint force mixing term. | |
void | SetLagrangeMultiplier (double ml_i) |
Set the value of the corresponding Lagrange multiplier (constraint reaction). | |
double | GetLagrangeMultiplier () const |
Return the corresponding Lagrange multiplier (constraint reaction). | |
virtual void | Update_auxiliary () |
This function updates the following auxiliary data: More... | |
double | GetSchurComplement () const |
Return the 'g_i' product, that is [Cq_i]*[invM_i]*[Cq_i]' (+cfm) | |
void | SetSchurComplement (double m_g_i) |
Usually you should not use the SetSchurComplement function, because g_i should be automatically computed during the Update_auxiliary() . | |
virtual double | ComputeJacobianTimesState ()=0 |
Compute the product between the Jacobian of this constraint, [Cq_i], and the vector of variables. More... | |
virtual void | IncrementState (double deltal)=0 |
Increment the vector of variables with the quantity [invM]*[Cq_i]'*deltal. More... | |
virtual void | AddJacobianTimesVectorInto (double &result, ChVectorConstRef vect) const =0 |
Add the product of the corresponding block in the system matrix by 'vect' and add to result. More... | |
virtual void | AddJacobianTransposedTimesScalarInto (ChVectorRef result, double l) const =0 |
Add the product of the corresponding transposed block in the system matrix by 'l' and add to result. More... | |
virtual void | Project () |
Project the value of a possible 'l_i' value of constraint reaction onto admissible orthant/set. More... | |
virtual double | Violation (double mc_i) |
Return the constraint violation. More... | |
virtual void | PasteJacobianInto (ChSparseMatrix &mat, unsigned int start_row, unsigned int start_col) const =0 |
Write the constraint Jacobian into the specified global matrix at the offsets of the associated variables. More... | |
virtual void | PasteJacobianTransposedInto (ChSparseMatrix &mat, unsigned int start_row, unsigned int start_col) const =0 |
Write the transposed constraint Jacobian into the specified global matrix at the offsets of the associated variables. More... | |
void | SetOffset (unsigned int off) |
Set offset in global q vector (set automatically by ChSystemDescriptor) | |
unsigned int | GetOffset () const |
Get offset in global q vector. | |
virtual void | ArchiveOut (ChArchiveOut &archive_out) |
Method to allow serialization of transient data to archives. | |
virtual void | ArchiveIn (ChArchiveIn &archive_in) |
Method to allow de-serialization of transient data from archives. | |
Protected Attributes | |
double | c_i |
constraint residual (if satisfied, c must be 0) | |
double | l_i |
Lagrange multiplier (reaction) | |
double | b_i |
right-hand side term in [Cq_i]*q+b_i=0 , note: c_i= [Cq_i]*q + b_i | |
double | cfm_i |
Constraint force mixing if needed to add some numerical 'compliance' in the constraint. More... | |
Mode | mode |
mode of the constraint | |
double | g_i |
product [Cq_i]*[invM_i]*[Cq_i]' (+cfm) | |
unsigned int | offset |
offset in global "l" state vector (needed by some solvers) | |
Member Enumeration Documentation
◆ Mode
|
strong |
Member Function Documentation
◆ AddJacobianTimesVectorInto()
|
pure virtual |
Add the product of the corresponding block in the system matrix by 'vect' and add to result.
Note: 'vect' is assumed to be of proper size; the procedure uses the ChVariable offsets to index in 'vect'.
Implemented in chrono::ChConstraintTwoGeneric, chrono::ChConstraintTwoTuples< Ta, Tb >, chrono::ChConstraintTwoTuples< chrono::fea::ChNodeFEAxyz, chrono::fea::ChTriangleNodesXYZ >, chrono::ChConstraintTwoTuples< chrono::fea::ChNodeFEAxyz, chrono::fea::ChTriangleNodesXYZrot >, chrono::ChConstraintTwoTuples< typecarr_a, typecarr_b >, chrono::ChConstraintThreeGeneric, chrono::ChConstraintThreeBBShaft, chrono::ChConstraintTwoBodies, and chrono::ChConstraintNgeneric.
◆ AddJacobianTransposedTimesScalarInto()
|
pure virtual |
Add the product of the corresponding transposed block in the system matrix by 'l' and add to result.
Note: 'result' is assumed to be of proper size; the procedure uses the ChVariable offsets to index in 'result'.
Implemented in chrono::ChConstraintTwoTuples< Ta, Tb >, chrono::ChConstraintTwoTuples< chrono::fea::ChNodeFEAxyz, chrono::fea::ChTriangleNodesXYZ >, chrono::ChConstraintTwoTuples< chrono::fea::ChNodeFEAxyz, chrono::fea::ChTriangleNodesXYZrot >, chrono::ChConstraintTwoTuples< typecarr_a, typecarr_b >, chrono::ChConstraintTwoGeneric, chrono::ChConstraintThreeGeneric, chrono::ChConstraintThreeBBShaft, chrono::ChConstraintTwoBodies, and chrono::ChConstraintNgeneric.
◆ ComputeJacobianTimesState()
|
pure virtual |
Compute the product between the Jacobian of this constraint, [Cq_i], and the vector of variables.
In other words, perform the operation:
CV = [Cq_i] * v
Implemented in chrono::ChConstraintTwoGeneric, chrono::ChConstraintTwoTuples< Ta, Tb >, chrono::ChConstraintTwoTuples< chrono::fea::ChNodeFEAxyz, chrono::fea::ChTriangleNodesXYZ >, chrono::ChConstraintTwoTuples< chrono::fea::ChNodeFEAxyz, chrono::fea::ChTriangleNodesXYZrot >, chrono::ChConstraintTwoTuples< typecarr_a, typecarr_b >, chrono::ChConstraintThreeGeneric, chrono::ChConstraintThreeBBShaft, chrono::ChConstraintTwoBodies, and chrono::ChConstraintNgeneric.
◆ ComputeResidual()
|
inlinevirtual |
Compute the residual of the constraint using the linear expression.
c_i= [Cq_i]*q + cfm_i*l_i + b_i
For a satisfied bilateral constraint, this residual must be near zero.
◆ GetMode()
|
inline |
Get the mode of the constraint.
A typical constraint has LOCK mode (bilateral) by default.
◆ IncrementState()
|
pure virtual |
Increment the vector of variables with the quantity [invM]*[Cq_i]'*deltal.
In other words, perform the operation:
v += [invM] * [Cq_i]' * deltal or else v+=[Eq_i] * deltal
Implemented in chrono::ChConstraintTwoGeneric, chrono::ChConstraintTwoTuples< Ta, Tb >, chrono::ChConstraintTwoTuples< chrono::fea::ChNodeFEAxyz, chrono::fea::ChTriangleNodesXYZ >, chrono::ChConstraintTwoTuples< chrono::fea::ChNodeFEAxyz, chrono::fea::ChTriangleNodesXYZrot >, chrono::ChConstraintTwoTuples< typecarr_a, typecarr_b >, chrono::ChConstraintThreeGeneric, chrono::ChConstraintThreeBBShaft, chrono::ChConstraintTwoBodies, and chrono::ChConstraintNgeneric.
◆ IsActive()
|
inline |
Indicate whether the constraint is currently active.
In general, this indicates if it must be included into the system solver or not. This method acumulates the effect of all flags, so a constraint may be inactive either because it is 'disabled', 'broken', 'redundant', or not 'valid'.
◆ PasteJacobianInto()
|
pure virtual |
Write the constraint Jacobian into the specified global matrix at the offsets of the associated variables.
The (start_row, start_col) pair specifies the top-left corner of the system-level constraint Jacobian in the provided matrix.
Implemented in chrono::ChConstraintTwoTuples< Ta, Tb >, chrono::ChConstraintTwoTuples< chrono::fea::ChNodeFEAxyz, chrono::fea::ChTriangleNodesXYZ >, chrono::ChConstraintTwoTuples< chrono::fea::ChNodeFEAxyz, chrono::fea::ChTriangleNodesXYZrot >, chrono::ChConstraintTwoTuples< typecarr_a, typecarr_b >, chrono::ChConstraintTwoGeneric, chrono::ChConstraintThreeGeneric, chrono::ChConstraintThreeBBShaft, chrono::ChConstraintTwoBodies, and chrono::ChConstraintNgeneric.
◆ PasteJacobianTransposedInto()
|
pure virtual |
Write the transposed constraint Jacobian into the specified global matrix at the offsets of the associated variables.
The (start_row, start_col) pair specifies the top-left corner of the system-level constraint Jacobian in the provided matrix.
Implemented in chrono::ChConstraintTwoTuples< Ta, Tb >, chrono::ChConstraintTwoTuples< chrono::fea::ChNodeFEAxyz, chrono::fea::ChTriangleNodesXYZ >, chrono::ChConstraintTwoTuples< chrono::fea::ChNodeFEAxyz, chrono::fea::ChTriangleNodesXYZrot >, chrono::ChConstraintTwoTuples< typecarr_a, typecarr_b >, chrono::ChConstraintTwoGeneric, chrono::ChConstraintThreeGeneric, chrono::ChConstraintThreeBBShaft, chrono::ChConstraintTwoBodies, and chrono::ChConstraintNgeneric.
◆ Project()
|
virtual |
Project the value of a possible 'l_i' value of constraint reaction onto admissible orthant/set.
Default behavior: if constraint is unilateral and l_i<0, reset l_i=0 Note: This function MAY BE OVERRIDDEN by specialized inherited classes. For example,
- a bilateral constraint does nothing
- a unilateral constraint: l_i= std::max(0., l_i)
- a 'boxed constraint': l_i= std::min(std::max(min., l_i), max)
Reimplemented in chrono::ChConstraintTwoTuplesRollingN< Ta, Tb >, chrono::ChConstraintTwoTuplesRollingN< typecarr_a, typecarr_b >, chrono::ChConstraintTwoTuplesContactN< Ta, Tb >, chrono::ChConstraintTwoTuplesContactN< typecarr_a, typecarr_b >, and chrono::ChConstraintTwoGenericBoxed.
◆ SetBroken()
|
inline |
Set the constraint as broken.
By default, constraints never break.
◆ SetComplianceTerm()
|
inline |
Set the constraint force mixing term (default=0).
This adds artificial 'elasticity' to the constraint, as c_i= [Cq_i]*q + b_i + cfm*l_i = 0.
◆ Update_auxiliary()
|
inlinevirtual |
This function updates the following auxiliary data:
- the Eq_a and Eq_b matrices
- the g_i product This function is often called by solvers at the beginning of the solution process. Note: This function must be overriden by specialized derived classes, which have some Jacobians.
Reimplemented in chrono::ChConstraintTwoGeneric, chrono::ChConstraintThreeGeneric, chrono::ChConstraintThreeBBShaft, chrono::ChConstraintTwoTuples< Ta, Tb >, chrono::ChConstraintTwoTuples< chrono::fea::ChNodeFEAxyz, chrono::fea::ChTriangleNodesXYZ >, chrono::ChConstraintTwoTuples< chrono::fea::ChNodeFEAxyz, chrono::fea::ChTriangleNodesXYZrot >, chrono::ChConstraintTwoTuples< typecarr_a, typecarr_b >, chrono::ChConstraintTwoBodies, and chrono::ChConstraintNgeneric.
◆ Violation()
|
virtual |
Return the constraint violation.
The function receives as input the linear map
mc_i = [Cq]*q + b_i + cfm*l_i
For bilateral constraint, violation = mc_i. For unilateral constraint, violation = min(mc_i, 0), For boxed constraints and similar, inherited class should override this implementation.
Reimplemented in chrono::ChConstraintTwoGenericBoxed, chrono::ChConstraintTwoTuplesFrictionT< Ta, Tb >, chrono::ChConstraintTwoTuplesFrictionT< typecarr_a, typecarr_b >, chrono::ChConstraintTwoTuplesRollingT< Ta, Tb >, and chrono::ChConstraintTwoTuplesRollingT< typecarr_a, typecarr_b >.
Member Data Documentation
◆ cfm_i
|
protected |
Constraint force mixing if needed to add some numerical 'compliance' in the constraint.
With this, the equation becomes: c_i= [Cq_i]*q + b_i + cfm*l_i =0. For example, this additional term could be: cfm = [k * h^2](^-1), where k is stiffness. Usually set to 0.
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono/solver/ChConstraint.h
- /builds/uwsbel/chrono/src/chrono/solver/ChConstraint.cpp