Description
Base class for a run-time visualization system for SPH-based FSI systems.
Note that using run-time visualization for an FSI system incurs the penalty of collecting positions of all particles every time the Render() function is invoked.
#include <ChFsiVisualization.h>
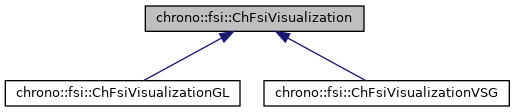
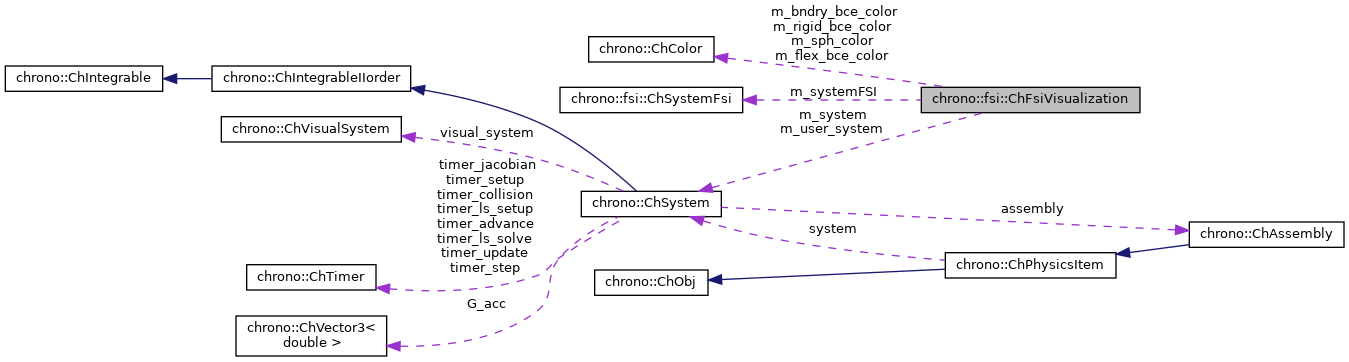
Classes | |
class | MarkerVisibilityCallback |
Class to be used as a callback interface for dynamic visibility of SPH particles or BCE markers. More... | |
class | ParticleColorCallback |
Class to be used as a callback interface for dynamic coloring of SPH particles. More... | |
Public Types | |
enum | RenderMode { POINTS, WIREFRAME, SOLID } |
Rendering mode for particles and mesh objects. | |
Public Member Functions | |
void | SetVerbose (bool verbose) |
Enable/disable information terminal output during initialization (default: false). | |
virtual void | SetTitle (const std::string &title) |
Set title of the visualization window (default: ""). | |
virtual void | SetSize (int width, int height) |
Set window dimensions (default: 1280x720). | |
virtual void | AddCamera (const ChVector3d &pos, const ChVector3d &target) |
Add a camera initially at the specified position and target (look at) point. | |
virtual void | UpdateCamera (const ChVector3d &pos, const ChVector3d &target) |
Set camera position and target (look at) point. | |
virtual void | SetCameraVertical (CameraVerticalDir up) |
Set camera up vector (default: Z). | |
virtual void | SetCameraMoveScale (float scale) |
Set scale for camera movement increments (default: 0.1). | |
virtual void | SetLightIntensity (double intensity) |
Set directional light intensity (default: 1). More... | |
virtual void | SetLightDirection (double azimuth, double elevation) |
Set azimuth and elevation of directional light in radians (default: 0, 0). More... | |
virtual void | SetParticleRenderMode (RenderMode mode) |
Set rendering mode for SPH particles. More... | |
virtual void | SetRenderMode (RenderMode mode) |
Set rendering mode for mesh objects (default: WIREFRAME). | |
void | SetColorFluidMarkers (const ChColor &col) |
Set default color for fluid SPH particles (default: [0.10, 0.40, 0.65]). | |
void | SetColorBoundaryMarkers (const ChColor &col) |
Set default color for boundary BCE markers (default: [0.65, 0.30, 0.03]). | |
void | SetColorRigidBodyMarkers (const ChColor &col) |
Set default color for rigid body BCE markers (default: [0.10, 0.60, 0.30]). | |
void | SetColorFlexBodyMarkers (const ChColor &col) |
Set default color for flex body BCE markers (default: [0.40, 0.10, 0.65]). | |
void | SetSPHColorCallback (std::shared_ptr< ParticleColorCallback > functor) |
Set a callback for dynamic coloring of SPH particles. More... | |
void | SetSPHVisibilityCallback (std::shared_ptr< MarkerVisibilityCallback > functor) |
Set a callback for dynamic visibility of SPH particles. More... | |
void | SetBCEVisibilityCallback (std::shared_ptr< MarkerVisibilityCallback > functor) |
Set a callback for dynamic visibility of boundary BCE markers. More... | |
void | SetImageOutputDirectory (const std::string &dir) |
Set output directory for saving frame snapshots (default: "."). | |
void | SetImageOutput (bool val) |
Enable/disable writing of frame snapshots to file. | |
virtual void | EnableInfoOverlay (bool val) |
Enable/disable information overlay (default: true). | |
void | EnableFluidMarkers (bool val) |
Enable/disable rendering of fluid SPH particles (default: true). | |
void | EnableRigidBodyMarkers (bool val) |
Enable/disable rendering of rigid-body BCE markers (default: true). | |
void | EnableFlexBodyMarkers (bool val) |
Enable/disable rendering of flex-body BCE markers (default: true). | |
void | EnableBoundaryMarkers (bool val) |
Enable/disable rendering of boundary BCE markers (default: false). | |
void | AttachSystem (ChSystem *system) |
Attach a user-provided Chrono system for rendering. More... | |
void | AddProxyBody (std::shared_ptr< ChBody > body) |
Add additional proxy body to supplemental system. More... | |
virtual void | Initialize () |
Initialize the run-time visualization system. | |
virtual bool | Render ()=0 |
Render the current state of the Chrono::Fsi system. More... | |
ChSystem * | GetSystem () const |
Return the internal Chrono system that holds visualization shapes. | |
virtual ChVisualSystem * | GetVisualSystem () const =0 |
Return the underlying visualization system. | |
Protected Member Functions | |
ChFsiVisualization (ChFsiSystemSPH *sysFSI) | |
Create a run-time FSI visualization object associated with a given Chrono::Fsi system. | |
ChFsiVisualization (ChFluidSystemSPH *sysSPH) | |
Create a run-time FSI visualization object associated with a given SPH fluid system. | |
Protected Attributes | |
ChFsiSystemSPH * | m_sysFSI |
associated FSI system | |
ChFluidSystemSPH * | m_sysSPH |
associated SPH system | |
ChSystem * | m_sysMBS |
internal Chrono system (holds proxies) | |
ChSystem * | m_user_system |
optional user-provided system | |
bool | m_sph_markers |
render fluid SPH particles? | |
bool | m_bndry_bce_markers |
render boundary BCE markers? | |
bool | m_rigid_bce_markers |
render rigid-body BCE markers? | |
bool | m_flex_bce_markers |
render flex-body markers? | |
std::shared_ptr< ChParticleCloud > | m_sph_cloud |
particle cloud proxy for SPH particles | |
std::shared_ptr< ChParticleCloud > | m_bndry_bce_cloud |
particle cloud proxy for boundary BCE markers | |
std::shared_ptr< ChParticleCloud > | m_rigid_bce_cloud |
particle cloud proxy for BCE markers on rigid bodies | |
std::shared_ptr< ChParticleCloud > | m_flex_bce_cloud |
particle cloud proxy for BCE markers on flex bodies | |
ChColor | m_sph_color |
color for SPH particles | |
ChColor | m_bndry_bce_color |
color for boundary BCE markers | |
ChColor | m_rigid_bce_color |
color for BCE markers on rigid bodies | |
ChColor | m_flex_bce_color |
color for BCE markers on flex bodies | |
std::shared_ptr< ParticleColorCallback > | m_color_fun |
color functor for SPH particles | |
std::shared_ptr< MarkerVisibilityCallback > | m_vis_sph_fun |
visibility functor for SPH particles | |
std::shared_ptr< MarkerVisibilityCallback > | m_vis_bndry_fun |
visibility functor for bndry BCE markers | |
bool | m_write_images |
if true, save snapshots | |
std::string | m_image_dir |
directory for image files | |
Member Function Documentation
◆ AddProxyBody()
void chrono::fsi::ChFsiVisualization::AddProxyBody | ( | std::shared_ptr< ChBody > | body | ) |
Add additional proxy body to supplemental system.
Must be called before Initialize(). The provided body is set fixed to ground and it is the caller's responsibility to update the position of this body before a call to Render().
◆ AttachSystem()
|
inline |
Attach a user-provided Chrono system for rendering.
By default, the FSI run-time visualization renders SPH particles and BCE markers. This function can be used to also render the mechanical system interacting with the SPH system.
◆ Render()
|
pure virtual |
Render the current state of the Chrono::Fsi system.
This function, typically invoked from within the main simulation loop, can only be called after construction of the FSI system was completed (i.e., the system was initialized). This function querries the positions of all particles in the FSI system in order to update the positions of the proxy bodies. Returns false if the visualization window was closed.
Implemented in chrono::fsi::ChFsiVisualizationVSG.
◆ SetBCEVisibilityCallback()
|
inline |
Set a callback for dynamic visibility of boundary BCE markers.
If none provided, all boundary BCE markers are visible.
◆ SetLightDirection()
|
virtual |
Set azimuth and elevation of directional light in radians (default: 0, 0).
The azimuth must be in [-pi,+pi], with 0 corresponding to the positive x direction. The elevation must be in [0, pi/2] with 0 corresponding to the horizontal.
Reimplemented in chrono::fsi::ChFsiVisualizationVSG.
◆ SetLightIntensity()
|
virtual |
Set directional light intensity (default: 1).
The light intensity must be in [0,1].
Reimplemented in chrono::fsi::ChFsiVisualizationVSG.
◆ SetParticleRenderMode()
|
virtual |
Set rendering mode for SPH particles.
Must be called before Initialize().
Reimplemented in chrono::fsi::ChFsiVisualizationVSG.
◆ SetSPHColorCallback()
|
inline |
Set a callback for dynamic coloring of SPH particles.
If none provided, SPH particles are rendered with a default color.
◆ SetSPHVisibilityCallback()
|
inline |
Set a callback for dynamic visibility of SPH particles.
If none provided, all SPH particles are visible.
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono_fsi/sph/visualization/ChFsiVisualization.h
- /builds/uwsbel/chrono/src/chrono_fsi/sph/visualization/ChFsiVisualization.cpp