Description
Base class for a system for fluid-solid interaction problems.
This class is used to represent fluid-solid interaction problems consisting of fluid dynamics and multibody system. Each of the two underlying physics is an independent object owned and instantiated by this class. The FSI system owns other objects to handle the interface between the two systems, boundary condition enforcing markers, and data.
#include <ChFsiSystem.h>
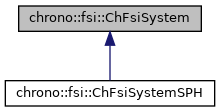
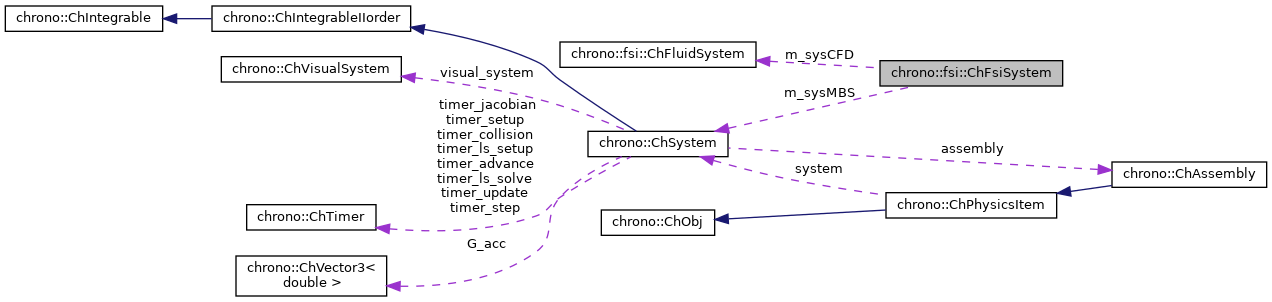
Classes | |
class | MBDCallback |
Class to specify step dynamics for the associated multibody system. More... | |
Public Member Functions | |
virtual | ~ChFsiSystem () |
Destructor for the FSI system. | |
ChFluidSystem & | GetFluidSystem () const |
Access the associated fluid system. | |
ChSystem & | GetMultibodySystem () const |
Access the associated multibody system. | |
ChFsiInterface & | GetFsiInterface () const |
Access the associated FSI interface. | |
void | SetVerbose (bool verbose) |
Enable/disable verbose terminal output (default: true). More... | |
void | SetGravitationalAcceleration (const ChVector3d &gravity) |
Set gravitational acceleration for the FSI syatem. More... | |
void | SetStepSizeCFD (double step) |
Set integration step size for fluid dynamics. | |
void | SetStepsizeMBD (double step) |
Set integration step size for multibody dynamics. More... | |
size_t | AddFsiBody (std::shared_ptr< ChBody > body) |
Add a rigid body to the FSI system. More... | |
void | AddFsiMesh (std::shared_ptr< fea::ChMesh > mesh) |
Add an FEA mesh to the FSI system. More... | |
virtual void | Initialize () |
Initialize the FSI system. More... | |
void | RegisterMBDCallback (std::shared_ptr< MBDCallback > callback) |
Set a custom function for advancing the dynamics of the associated multibody system. More... | |
void | DisableMBD () |
Disable automatic integration of the associated multibody system. More... | |
void | DoStepDynamics (double step) |
Function to advance the FSI system combined state. More... | |
double | GetSimTime () const |
Get current simulation time. | |
double | GetStepSizeMBD () const |
Get the integration step size for multibody dynamics. | |
double | GetStepSizeCFD () const |
Get the integration step size for fluid dynamics. | |
double | GetRtf () const |
Get current estimated RTF (real time factor) for the coupled problem. | |
void | SetRtf (double rtf) |
Set RTF value for the coupled problem. More... | |
double | GetRtfCFD () const |
Get current estimated RTF (real time factor) for the fluid system. | |
double | GetRtfMBD () const |
Get current estimated RTF (real time factor) for the multibody system. | |
double | GetRatioMBD () const |
Get ratio of simulation time spent in MBS integration. | |
double | GetTimerStep () const |
Return the time in seconds for for simulating the last step. | |
double | GetTimerCFD () const |
Return the time in seconds for fluid dynamics over the last step. | |
double | GetTimerMBD () const |
Return the time in seconds for multibody dynamics over the last step. | |
double | GetTimerFSI () const |
Return the time in seconds for data exchange between phases over the last step. | |
const ChVector3d & | GetFsiBodyForce (size_t i) const |
Return the FSI applied force on the body with specified index (as returned by AddFsiBody). More... | |
const ChVector3d & | GetFsiBodyTorque (size_t i) const |
Return the FSI applied torque on the body with specified index (as returned by AddFsiBody). More... | |
Protected Member Functions | |
ChFsiSystem (ChSystem &sysMBS, ChFluidSystem &sysCFD) | |
Protected Attributes | |
ChSystem & | m_sysMBS |
multibody system | |
ChFluidSystem & | m_sysCFD |
FSI fluid solver. | |
std::shared_ptr< ChFsiInterface > | m_fsi_interface |
FSI interface system. | |
bool | m_verbose |
enable/disable m_verbose terminal output | |
bool | m_is_initialized |
set to true once the Initialize function is called | |
Member Function Documentation
◆ AddFsiBody()
size_t chrono::fsi::ChFsiSystem::AddFsiBody | ( | std::shared_ptr< ChBody > | body | ) |
Add a rigid body to the FSI system.
Returns the index of the FSI body in the internal list.
◆ AddFsiMesh()
void chrono::fsi::ChFsiSystem::AddFsiMesh | ( | std::shared_ptr< fea::ChMesh > | mesh | ) |
Add an FEA mesh to the FSI system.
Contact surfaces (of type segment_set or tri_mesh) already defined for the FEA mesh are used to generate the interface between the solid and fluid phases. If none are defined, one contact surface of each type is created (as needed), but these are not attached to the given FEA mesh.
◆ DisableMBD()
|
inline |
Disable automatic integration of the associated multibody system.
Notes:
- By default, DoStepDynamics integrates both the multibody and fluid systems.
- If MBD integration is disabled, it is the caller's responsibility to advance the dynamics of the associated multibody system, separate from the call to ChFsiSystem::DoStepDynamics (which, in this case, only performs the data exchange between the two systems and advances the dynamics of the fluid system).
- The multibody system dynamics must be advanced after the call to ChFsiSystem::DoStepDynamics.
◆ DoStepDynamics()
void chrono::fsi::ChFsiSystem::DoStepDynamics | ( | double | step | ) |
Function to advance the FSI system combined state.
This implements an explicit force-displacement co-simulation step:
- advance fluid dynamics to new data exchange point
- apply fluid forces on solid objects
- advance multibody dynamics to new data exchange point
- extract new states for FSI solid objects If enbaled, the multibody step dynamics is ran in a separate thread and does not block execution.
◆ GetFsiBodyForce()
const ChVector3d & chrono::fsi::ChFsiSystem::GetFsiBodyForce | ( | size_t | i | ) | const |
Return the FSI applied force on the body with specified index (as returned by AddFsiBody).
The force is applied at the body COM and is expressed in the absolute frame.
◆ GetFsiBodyTorque()
const ChVector3d & chrono::fsi::ChFsiSystem::GetFsiBodyTorque | ( | size_t | i | ) | const |
Return the FSI applied torque on the body with specified index (as returned by AddFsiBody).
The torque is expressed in the absolute frame.
◆ Initialize()
|
virtual |
Initialize the FSI system.
A call to this function marks the completion of system construction. The default implementation simply checks that an associated FSI interface was created and a value for integration step size was provided.
◆ RegisterMBDCallback()
|
inline |
Set a custom function for advancing the dynamics of the associated multibody system.
If not provided, the MBS system is integrated with the specified step size (see SetStepsizeMBD).
◆ SetGravitationalAcceleration()
void chrono::fsi::ChFsiSystem::SetGravitationalAcceleration | ( | const ChVector3d & | gravity | ) |
Set gravitational acceleration for the FSI syatem.
This function sets gravity for both the fluid and multibody systems.
◆ SetRtf()
|
inline |
Set RTF value for the coupled problem.
This is necessary for correct reporting if automatic integration of the multibody system dynamics is disabled and performed externally.
◆ SetStepsizeMBD()
void chrono::fsi::ChFsiSystem::SetStepsizeMBD | ( | double | step | ) |
Set integration step size for multibody dynamics.
If a value is not provided, the MBS system is integrated with the same step used for fluid dynamics.
◆ SetVerbose()
void chrono::fsi::ChFsiSystem::SetVerbose | ( | bool | verbose | ) |
Enable/disable verbose terminal output (default: true).
The default implementation sets the verbose mode for the FSI system and the underlying FSI interface.
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono_fsi/ChFsiSystem.h
- /builds/uwsbel/chrono/src/chrono_fsi/ChFsiSystem.cpp