Description
Cubic fillet function.
Cubic polynomial y(x)
that smoothly connects two points with given start and end derivatives.
y(0)
= GetStartVal()y(width)
= GetEndVal()der(y)(0)
= GetStartDer()der(y)(width)
= GetEndDer()
#include <ChFunctionFillet3.h>
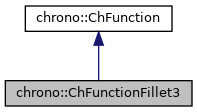
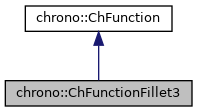
Public Member Functions | |
ChFunctionFillet3 (const ChFunctionFillet3 &other) | |
virtual ChFunctionFillet3 * | Clone () const override |
"Virtual" copy constructor (covariant return type). | |
virtual Type | GetType () const override |
Return the unique function type identifier. | |
virtual double | GetVal (double x) const override |
Return the function output for input x. More... | |
virtual double | GetDer (double x) const override |
Return the first derivative of the function. More... | |
virtual double | GetDer2 (double x) const override |
Return the second derivative of the function. More... | |
void | SetWidth (double width) |
double | GetWidth () |
void | Setup () |
Setup the function after parameter changes. More... | |
void | SetStartVal (double val_start) |
Set the initial value of the function. | |
void | SetEndVal (double val_end) |
Set the end value of the function. | |
void | SetStartDer (double der_start) |
Set the initial derivative of the function. | |
void | SetEndDer (double der_end) |
Set the end derivative of the function. | |
double | GetStartVal () |
Get the initial value of the function. | |
double | GetEndVal () |
Get the end value of the function. | |
double | GetStartDer () |
Get the initial derivative of the function. | |
double | GetEndDer () |
Get the end derivative of the function. | |
virtual void | ArchiveOut (ChArchiveOut &archive_out) override |
Method to allow serialization of transient data to archives. | |
virtual void | ArchiveIn (ChArchiveIn &archive_in) override |
Method to allow de-serialization of transient data from archives. | |
![]() | |
ChFunction (const ChFunction &other) | |
virtual double | GetDer3 (double x) const |
Return the third derivative of the function. More... | |
virtual double | GetDerN (double x, int der_order) const |
Return the Nth derivative of the function (up to 3rd derivative). More... | |
virtual double | GetWeight (double x) const |
Return the weight of the function. More... | |
virtual void | Update (double x) |
Update the function at the provided value of its argument. | |
virtual double | GetMax (double xmin, double xmax, double sampling_step, int der_order) const |
Estimate the maximum of the function (or its der_order derivative) in the range [xmin, xmax]. More... | |
virtual double | GetMin (double xmin, double xmax, double sampling_step, int der_order) const |
Estimate the minimum of the function (or its der_order derivative) in the range [xmin, xmax]. More... | |
virtual double | GetMean (double xmin, double xmax, double sampling_step, int der_order) const |
Estimate the mean of the function (or its der_order derivative) in the range [xmin, xmax]. More... | |
virtual double | GetSquaredMean (double xmin, double xmax, double sampling_step, int der_order) const |
Estimate the squared mean of the function (or its der_order derivative) in the range [xmin, xmax]. More... | |
virtual double | GetIntegral (double xmin, double xmax, double sampling_step, int der_order) const |
Estimate the integral of the function (or its der_order derivative) over the range [xmin, xmax]. More... | |
virtual double | GetPositiveAccelerationCoeff () const |
Compute the positive acceleration coefficient. More... | |
virtual double | GetNegativeAccelerationCoeff () const |
Compute the negative acceleration coefficient. More... | |
virtual double | GetVelocityCoefficient () const |
Compute the velocity coefficient. More... | |
virtual void | OutputToASCIIFile (std::ostream &file, double xmin, double xmax, int samples, char delimiter) |
Store X-Y pairs to an ASCII File. More... | |
virtual ChMatrixDynamic | SampleUpToDerN (double xmin, double xmax, double step, int derN=0) |
Sample function on given interval [xmin, xmax], up to derN derivative (0 being the function ouput itself). More... | |
double | operator() (double arg) const |
Alias operator of the GetVal function. | |
Additional Inherited Members | |
![]() | |
enum | Type { BSPLINE, CONSTANT, CONSTACC, CONSTJERK, CUSTOM, CYCLOIDAL, DERIVATIVE, FILLET3, INTEGRAL, INTERP, LAMBDA, MIRROR, OPERATOR, POLY, POLY23, POLY345, RAMP, REPEAT, SEQUENCE, SINE, SINE_STEP } |
Enumeration of function types. | |
Member Function Documentation
◆ GetDer()
|
overridevirtual |
Return the first derivative of the function.
Default implementation computes a numerical differentiation. Inherited classes may override this method with a more efficient implementation (e.g. analytical solution).
Reimplemented from chrono::ChFunction.
◆ GetDer2()
|
overridevirtual |
Return the second derivative of the function.
Default implementation computes a numerical differentiation. Inherited classes may override this method with a more efficient implementation (e.g. analytical solution).
Reimplemented from chrono::ChFunction.
◆ GetVal()
|
overridevirtual |
Return the function output for input x.
Must be overridden by specialized classes.
Implements chrono::ChFunction.
◆ Setup()
void chrono::ChFunctionFillet3::Setup | ( | ) |
Setup the function after parameter changes.
This must be called by the user after the parameters are changed.
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono/functions/ChFunctionFillet3.h
- /builds/uwsbel/chrono/src/chrono/functions/ChFunctionFillet3.cpp