Description
This class is inherited from the base ChConstraintTwoGeneric, which can be used for most pairwise constraints, and adds the feature that the multiplier must be l_min < l < l_max that is, the multiplier is 'boxed'.
Note that, if l_min = 0 and l_max = infinite, this can work also as an unilateral constraint.. Before starting the solver one must provide the proper values in constraints (and update them if necessary), i.e. must set at least the c_i and b_i values, and jacobians.
#include <ChConstraintTwoGenericBoxed.h>
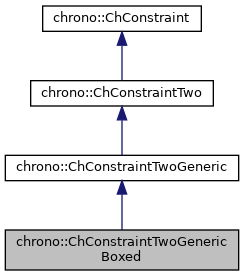
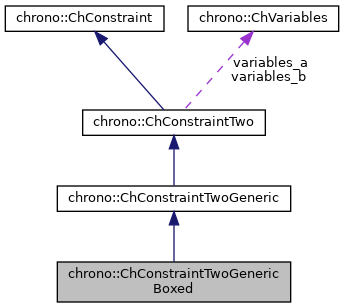
Public Member Functions | |
ChConstraintTwoGenericBoxed () | |
Default constructor. | |
ChConstraintTwoGenericBoxed (ChVariables *mvariables_a, ChVariables *mvariables_b) | |
Construct and immediately set references to variables. | |
ChConstraintTwoGenericBoxed (const ChConstraintTwoGenericBoxed &other) | |
Copy constructor. | |
virtual ChConstraintTwoGenericBoxed * | Clone () const override |
"Virtual" copy constructor (covariant return type). | |
ChConstraintTwoGenericBoxed & | operator= (const ChConstraintTwoGenericBoxed &other) |
Assignment operator: copy from other object. | |
void | SetBoxedMinMax (double mmin, double mmax) |
Set lower/upper limit for the multiplier. | |
double | GetBoxedMin () |
Get the lower limit for the multiplier. | |
double | GetBoxedMax () |
Get the upper limit for the multiplier. | |
virtual void | Project () override |
For iterative solvers: project the value of a possible 'l_i' value of constraint reaction onto admissible orthant/set. More... | |
virtual double | Violation (double mc_i) override |
Given the residual of the constraint computed as the linear map mc_i = [Cq]*q + b_i + cfm*l_i , returns the violation of the constraint, considering inequalities, etc. | |
virtual void | ArchiveOut (ChArchiveOut &archive_out) override |
Method to allow serialization of transient data to archives. | |
virtual void | ArchiveIn (ChArchiveIn &archive_in) override |
Method to allow de-serialization of transient data from archives. | |
![]() | |
ChConstraintTwoGeneric () | |
Default constructor. | |
ChConstraintTwoGeneric (ChVariables *mvariables_a, ChVariables *mvariables_b) | |
Construct and immediately set references to variables. | |
ChConstraintTwoGeneric (const ChConstraintTwoGeneric &other) | |
Copy constructor. | |
ChConstraintTwoGeneric & | operator= (const ChConstraintTwoGeneric &other) |
Assignment operator: copy from other object. | |
virtual ChRowVectorRef | Get_Cq_a () override |
Access Jacobian vector. | |
virtual ChRowVectorRef | Get_Cq_b () override |
Access Jacobian vector. | |
virtual ChVectorRef | Get_Eq_a () override |
Access auxiliary vector (ex: used by iterative solvers). | |
virtual ChVectorRef | Get_Eq_b () override |
Access auxiliary vector (ex: used by iterative solvers). | |
virtual void | SetVariables (ChVariables *mvariables_a, ChVariables *mvariables_b) override |
Set references to the constrained ChVariables objects, automatically creating/resizing Jacobians as needed. | |
virtual void | Update_auxiliary () override |
This function updates the following auxiliary data: More... | |
virtual double | ComputeJacobianTimesState () override |
Compute the product between the Jacobian of this constraint, [Cq_i], and the vector of variables. More... | |
virtual void | IncrementState (double deltal) override |
Increment the vector of variables with the quantity [invM]*[Cq_i]'*deltal. More... | |
virtual void | AddJacobianTimesVectorInto (double &result, ChVectorConstRef vect) const override |
Add the product of the corresponding block in the system matrix by 'vect' and add to result. More... | |
virtual void | AddJacobianTransposedTimesScalarInto (ChVectorRef result, double l) const override |
Add the product of the corresponding transposed block in the system matrix by 'l' and add to result. More... | |
virtual void | PasteJacobianInto (ChSparseMatrix &mat, unsigned int start_row, unsigned int start_col) const override |
Write the constraint Jacobian into the specified global matrix at the offsets of the associated variables. More... | |
virtual void | PasteJacobianTransposedInto (ChSparseMatrix &mat, unsigned int start_row, unsigned int start_col) const override |
Write the transposed constraint Jacobian into the specified global matrix at the offsets of the associated variables. More... | |
![]() | |
ChConstraintTwo () | |
Default constructor. | |
ChConstraintTwo (const ChConstraintTwo &other) | |
Copy constructor. | |
ChConstraintTwo & | operator= (const ChConstraintTwo &other) |
Assignment operator: copy from other object. | |
ChVariables * | GetVariables_a () |
Access the first variable object. | |
ChVariables * | GetVariables_b () |
Access the second variable object. | |
![]() | |
ChConstraint (const ChConstraint &other) | |
ChConstraint & | operator= (const ChConstraint &other) |
Assignment operator: copy from other object. | |
bool | operator== (const ChConstraint &other) const |
Comparison (compares only flags, not the Jacobians). | |
bool | IsValid () const |
Indicate if the constraint data is currently valid. | |
void | SetValid (bool mon) |
Set the "valid" state of this constraint. | |
bool | IsDisabled () const |
Indicate if the constraint is currently turned on or off. | |
void | SetDisabled (bool mon) |
bool | IsRedundant () const |
Indicate if the constraint is redundant or singular. | |
void | SetRedundant (bool mon) |
Mark the constraint as redundant. | |
bool | IsBroken () const |
Indicate if the constraint is broken, due to excess pulling/pushing. | |
void | SetBroken (bool mon) |
Set the constraint as broken. More... | |
virtual bool | IsUnilateral () const |
Indicate if the constraint is unilateral (typical complementarity constraint). | |
virtual bool | IsLinear () const |
Indicate if the constraint is linear. | |
Mode | GetMode () const |
Get the mode of the constraint. More... | |
void | SetMode (Mode mmode) |
Set the mode of the constraint. | |
bool | IsActive () const |
Indicate whether the constraint is currently active. More... | |
void | SetActive (bool isactive) |
Set the status of the constraint to active. | |
virtual double | ComputeResidual () |
Compute the residual of the constraint using the linear expression. More... | |
double | GetResidual () const |
Return the residual of this constraint. | |
void | SetRightHandSide (const double mb) |
Sets the known term b_i in [Cq_i]*q + b_i = 0, where: c_i = [Cq_i]*q + b_i = 0. | |
double | GetRightHandSide () const |
Return the known term b_i in [Cq_i]*q + b_i = 0, where: c_i= [Cq_i]*q + b_i = 0. | |
void | SetComplianceTerm (const double mcfm) |
Set the constraint force mixing term (default=0). More... | |
double | GetComplianceTerm () const |
Return the constraint force mixing term. | |
void | SetLagrangeMultiplier (double ml_i) |
Set the value of the corresponding Lagrange multiplier (constraint reaction). | |
double | GetLagrangeMultiplier () const |
Return the corresponding Lagrange multiplier (constraint reaction). | |
double | GetSchurComplement () const |
Return the 'g_i' product, that is [Cq_i]*[invM_i]*[Cq_i]' (+cfm) | |
void | SetSchurComplement (double m_g_i) |
Usually you should not use the SetSchurComplement function, because g_i should be automatically computed during the Update_auxiliary() . | |
void | SetOffset (unsigned int off) |
Set offset in global q vector (set automatically by ChSystemDescriptor) | |
unsigned int | GetOffset () const |
Get offset in global q vector. | |
Protected Attributes | |
double | l_min |
double | l_max |
![]() | |
ChRowVectorDynamic< double > | Cq_a |
The [Cq_a] jacobian of the constraint. | |
ChRowVectorDynamic< double > | Cq_b |
The [Cq_b] jacobian of the constraint. | |
ChVectorDynamic< double > | Eq_a |
The [Eq_a] product [Eq_a]=[invM_a]*[Cq_a]'. | |
ChVectorDynamic< double > | Eq_b |
The [Eq_a] product [Eq_b]=[invM_b]*[Cq_b]'. | |
![]() | |
ChVariables * | variables_a |
The first constrained object. | |
ChVariables * | variables_b |
The second constrained object. | |
![]() | |
double | c_i |
constraint residual (if satisfied, c must be 0) | |
double | l_i |
Lagrange multiplier (reaction) | |
double | b_i |
right-hand side term in [Cq_i]*q+b_i=0 , note: c_i= [Cq_i]*q + b_i | |
double | cfm_i |
Constraint force mixing if needed to add some numerical 'compliance' in the constraint. More... | |
Mode | mode |
mode of the constraint | |
double | g_i |
product [Cq_i]*[invM_i]*[Cq_i]' (+cfm) | |
unsigned int | offset |
offset in global "l" state vector (needed by some solvers) | |
Additional Inherited Members | |
![]() | |
enum | Mode { Mode::FREE, Mode::LOCK, Mode::UNILATERAL, Mode::FRICTION } |
Constraint mode. More... | |
Member Function Documentation
◆ Project()
|
overridevirtual |
For iterative solvers: project the value of a possible 'l_i' value of constraint reaction onto admissible orthant/set.
This 'boxed implementation overrides the default do-nothing case.
Reimplemented from chrono::ChConstraint.
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono/solver/ChConstraintTwoGenericBoxed.h
- /builds/uwsbel/chrono/src/chrono/solver/ChConstraintTwoGenericBoxed.cpp