Description
Agent wrapper of a wheeled vehicle, in particular holds a pointer to a ChWheeledVehicle and sends out SynWheeledVehicleMessage-s to synchronize its state.
#include <SynWheeledVehicleAgent.h>
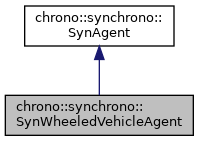
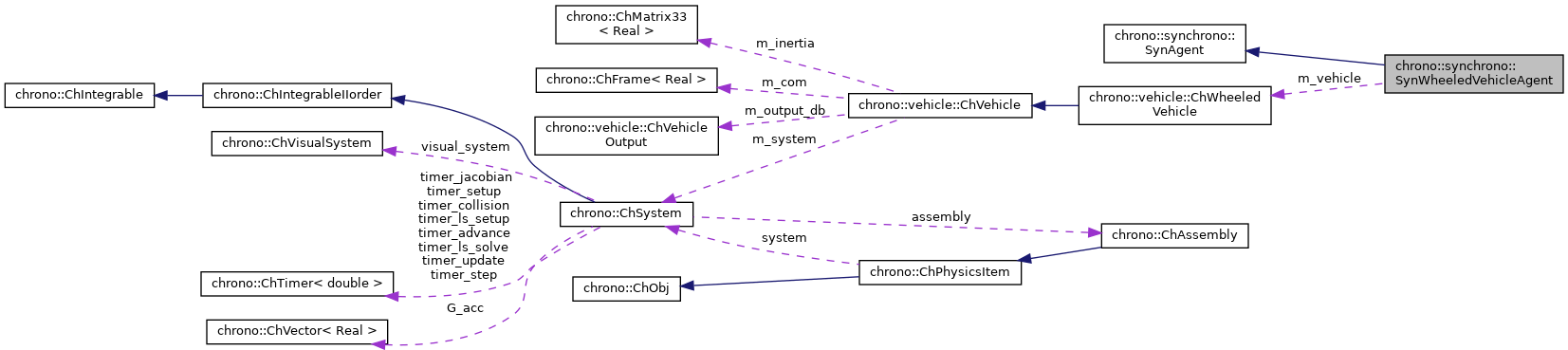
Public Member Functions | |
SynWheeledVehicleAgent (chrono::vehicle::ChWheeledVehicle *vehicle=nullptr, const std::string &filename="") | |
Construct a wheeled vehicle agent with optionally a vehicle. More... | |
virtual | ~SynWheeledVehicleAgent () |
Destructor. | |
virtual void | InitializeZombie (ChSystem *system) override |
Initialize this agents zombie representation Bodies are added and represented in the lead agent's world. More... | |
virtual void | SynchronizeZombie (std::shared_ptr< SynMessage > message) override |
Synchronize this agents zombie with the rest of the simulation. More... | |
virtual void | Update () override |
Update this agent Typically used to update the state representation of the agent to be distributed to other agents. More... | |
virtual void | GatherMessages (SynMessageList &messages) override |
Generates messages to be sent to other nodes Will create or get messages and pass them into the referenced message vector. More... | |
virtual void | GatherDescriptionMessages (SynMessageList &messages) override |
Get the description messages for this agent A single agent may have multiple description messages. More... | |
void | SetZombieVisualizationFilesFromJSON (const std::string &filename) |
Set the zombie visualization files from a JSON specification file. More... | |
void | SetZombieVisualizationFiles (std::string chassis_vis_file, std::string wheel_vis_file, std::string tire_vis_file) |
Set the zombie visualization files. More... | |
void | SetNumWheels (int num_wheels) |
Set the number of wheels of the underlying vehicle. More... | |
virtual void | SetKey (AgentKey agent_key) override |
Set the Agent ID. More... | |
![]() | |
SynAgent (AgentKey agent_key={0, 0}) | |
Construct a agent with the specified node_id. More... | |
virtual | ~SynAgent () |
Destructor. | |
virtual void | ProcessMessage (std::shared_ptr< SynMessage > msg) |
Process an incoming message. More... | |
virtual void | RegisterZombie (std::shared_ptr< SynAgent > zombie) |
Register a new zombie. More... | |
void | SetProcessMessageCallback (std::function< void(std::shared_ptr< SynMessage >)> callback) |
int | GetID () |
AgentKey | GetKey () |
Protected Member Functions | |
std::shared_ptr< ChTriangleMeshShape > | CreateMeshZombieComponent (const std::string &filename) |
Helper method used to create a ChTriangleMeshShape to be used on as a zombie body. More... | |
std::shared_ptr< ChBodyAuxRef > | CreateChassisZombieBody (const std::string &filename, ChSystem *system) |
Create a zombie chassis body. More... | |
Protected Attributes | |
chrono::vehicle::ChWheeledVehicle * | m_vehicle |
Pointer to the ChWheeledVehicle this class wraps. | |
std::shared_ptr< SynWheeledVehicleStateMessage > | m_state |
State of the vehicle (See SynWheeledVehicleMessage) | |
std::shared_ptr< SynWheeledVehicleDescriptionMessage > | m_description |
Description for zombie creation on discovery. | |
std::shared_ptr< ChBodyAuxRef > | m_zombie_body |
agent's zombie body reference | |
std::vector< std::shared_ptr< ChBodyAuxRef > > | m_wheel_list |
vector of this agent's zombie wheels | |
![]() | |
AgentKey | m_agent_key |
std::function< void(std::shared_ptr< SynMessage >)> | m_process_message_callback |
Constructor & Destructor Documentation
◆ SynWheeledVehicleAgent()
chrono::synchrono::SynWheeledVehicleAgent::SynWheeledVehicleAgent | ( | chrono::vehicle::ChWheeledVehicle * | vehicle = nullptr , |
const std::string & | filename = "" |
||
) |
Construct a wheeled vehicle agent with optionally a vehicle.
- Parameters
-
vehicle the vehicle this agent is responsible for (will be null if agent's a zombie) filename json specification file for zombie visualization (will query vehicle if not passed)
Member Function Documentation
◆ CreateChassisZombieBody()
|
protected |
Create a zombie chassis body.
All ChVehicles have a chassis, so this can be defined here
- Parameters
-
filename the filename that describes the ChTriangleMeshShape that should represent the chassis system the system to add the body to
◆ CreateMeshZombieComponent()
|
protected |
Helper method used to create a ChTriangleMeshShape to be used on as a zombie body.
- Parameters
-
filename the file to generate a ChTriangleMeshShape from
- Returns
- std::shared_ptr<ChTriangleMeshShape>
◆ GatherDescriptionMessages()
|
inlineoverridevirtual |
Get the description messages for this agent A single agent may have multiple description messages.
- Parameters
-
messages a referenced vector containing messages to be distributed from this rank
Implements chrono::synchrono::SynAgent.
◆ GatherMessages()
|
inlineoverridevirtual |
Generates messages to be sent to other nodes Will create or get messages and pass them into the referenced message vector.
- Parameters
-
messages a referenced vector containing messages to be distributed from this rank
Implements chrono::synchrono::SynAgent.
◆ InitializeZombie()
|
overridevirtual |
Initialize this agents zombie representation Bodies are added and represented in the lead agent's world.
- Parameters
-
system the ChSystem used to initialize the zombie
Implements chrono::synchrono::SynAgent.
◆ SetKey()
|
overridevirtual |
Set the Agent ID.
Reimplemented from chrono::synchrono::SynAgent.
◆ SetNumWheels()
|
inline |
Set the number of wheels of the underlying vehicle.
- Parameters
-
num_wheels number of wheels of the underlying vehicle
◆ SetZombieVisualizationFiles()
|
inline |
Set the zombie visualization files.
- Parameters
-
chassis_vis_file the file used for chassis visualization wheel_vis_file the file used for wheel visualization tire_vis_file the file used for tire visualization
◆ SetZombieVisualizationFilesFromJSON()
void chrono::synchrono::SynWheeledVehicleAgent::SetZombieVisualizationFilesFromJSON | ( | const std::string & | filename | ) |
Set the zombie visualization files from a JSON specification file.
- Parameters
-
filename the json specification file
◆ SynchronizeZombie()
|
overridevirtual |
Synchronize this agents zombie with the rest of the simulation.
Updates agent based on the passed message. Any message can be passed, so a check should be done to ensure this message was intended for this agent.
- Parameters
-
message the message to process and is used to update the position of the zombie
Implements chrono::synchrono::SynAgent.
◆ Update()
|
overridevirtual |
Update this agent Typically used to update the state representation of the agent to be distributed to other agents.
Implements chrono::synchrono::SynAgent.
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono_synchrono/agent/SynWheeledVehicleAgent.h
- /builds/uwsbel/chrono/src/chrono_synchrono/agent/SynWheeledVehicleAgent.cpp