Description
Derived communicator used to establish and facilitate communication between nodes.
Uses the Data Distribution Service (DDS) standard
#include <SynDDSCommunicator.h>
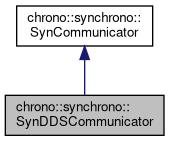
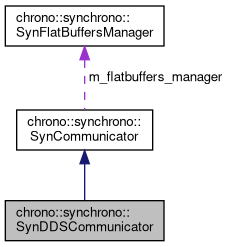
Public Member Functions | |
SynDDSCommunicator (int node_id, const std::string &prefix=default_prefix) | |
SynDDSCommunicator (const std::string &name, const std::string &prefix=default_prefix) | |
Default constructor. More... | |
SynDDSCommunicator (eprosima::fastdds::dds::DomainParticipantQos &qos, const std::string &prefix=default_prefix) | |
Set the QoS directly from the constructor. More... | |
virtual | ~SynDDSCommunicator () |
Destructor. More... | |
virtual void | Initialize () override |
Initialization function typically responsible for establishing a connection. More... | |
virtual void | Synchronize () override |
This function is responsible for continuous synchronization steps This function, depending on it's implementation, could be blocking or non-blocking. More... | |
virtual void | Barrier () override |
This function is responsible for blocking until an action is received or done. More... | |
void | Barrier (unsigned int num_participants) |
This function is responsible for blocking until the passed number of participants has been matched. More... | |
std::vector< std::string > | GetMatchedParticipantNames () |
Get the matched participant's names A matched participant is defined as a DDSParticipant Entity that has matched with the participant created on this node. More... | |
std::shared_ptr< SynDDSTopic > | CreateTopic (const std::string &topic_name, eprosima::fastdds::dds::TopicDataType *data_type, const std::string &topic_prefix) |
Create a topic The topic will be registered with the participant, if desired. More... | |
std::shared_ptr< SynDDSTopic > | CreateTopic (const std::string &topic_name, eprosima::fastdds::dds::TopicDataType *data_type) |
Create a topic using the communicator's prefix The topic will be registered with the participant, if desired. More... | |
std::shared_ptr< SynDDSSubscriber > | CreateSubscriber (std::shared_ptr< SynDDSTopic > topic, std::function< void(void *)> callback, void *message, bool is_synchronous=true, bool is_managed=false, eprosima::fastdds::dds::DataReaderQos *read_qos=nullptr) |
Create a subscription to the specified topic Will call the callback when a subscription is received Takes a SynDDSTopic object (see CreateTopic) More... | |
std::shared_ptr< SynDDSSubscriber > | CreateSubscriber (const std::string &topic_name, eprosima::fastdds::dds::TopicDataType *data_type, std::function< void(void *)> callback, void *message, bool is_synchronous=true, bool is_managed=false) |
Create a subscription to the specified topic Will call the callback when a subscription is received Takes a string and TopicDataType and creates a topic (see CreateTopic) More... | |
std::shared_ptr< SynDDSPublisher > | CreatePublisher (std::shared_ptr< SynDDSTopic > topic, bool is_managed=false, eprosima::fastdds::dds::DataWriterQos *write_qos=nullptr) |
Create a Publisher Returns a publisher handle to be used to publish information Takes a SynDDSTopic object (see CreateTopic) More... | |
std::shared_ptr< SynDDSPublisher > | CreatePublisher (const std::string &topic_name, eprosima::fastdds::dds::TopicDataType *data_type, bool is_managed=false) |
Create a Publisher Returns a publisher handle to be used to publish information Takes a string and TopicDataType and creates a topic (see CreateTopic) More... | |
![]() | |
SynCommunicator () | |
Default constructor. More... | |
virtual | ~SynCommunicator () |
Destructor. More... | |
void | Reset () |
Reset the communicator Will clear out message buffers. More... | |
void | AddOutgoingMessages (SynMessageList &messages) |
Add the messages to the outgoing message buffer. More... | |
void | AddQuitMessage () |
Adds a quit message to the queue telling other nodes to end the simulation. | |
void | AddIncomingMessages (SynMessageList &messages) |
Add the messages to the incoming message buffer. More... | |
void | ProcessBuffer (std::vector< uint8_t > &data) |
Process a data buffer by passing it to the underlying FlatBuffersManager. More... | |
virtual SynMessageList & | GetMessages () |
Get the messages received by the communicator. More... | |
Public Attributes | |
std::string | m_prefix |
Additional Inherited Members | |
![]() | |
bool | m_initialized |
whether the communicator has been initialized | |
SynMessageList | m_incoming_messages |
Incoming messages. | |
SynFlatBuffersManager | m_flatbuffers_manager |
flatbuffer manager for this rank | |
Constructor & Destructor Documentation
◆ SynDDSCommunicator() [1/2]
chrono::synchrono::SynDDSCommunicator::SynDDSCommunicator | ( | const std::string & | name, |
const std::string & | prefix = default_prefix |
||
) |
Default constructor.
- Parameters
-
name The name to set to the qos
◆ SynDDSCommunicator() [2/2]
chrono::synchrono::SynDDSCommunicator::SynDDSCommunicator | ( | eprosima::fastdds::dds::DomainParticipantQos & | qos, |
const std::string & | prefix = default_prefix |
||
) |
Set the QoS directly from the constructor.
- Parameters
-
qos the Quality of Service to set for the participant
◆ ~SynDDSCommunicator()
|
virtual |
Destructor.
Member Function Documentation
◆ Barrier() [1/2]
|
overridevirtual |
This function is responsible for blocking until an action is received or done.
For example, a process may call Barrier to wait until another process has established certain classes and initialized certain quantities. This functionality should be implemented in this function.
Implements chrono::synchrono::SynCommunicator.
◆ Barrier() [2/2]
void chrono::synchrono::SynDDSCommunicator::Barrier | ( | unsigned int | num_participants | ) |
This function is responsible for blocking until the passed number of participants has been matched.
- Parameters
-
num_participants number of participants to block until matched
◆ CreatePublisher() [1/2]
std::shared_ptr< SynDDSPublisher > chrono::synchrono::SynDDSCommunicator::CreatePublisher | ( | const std::string & | topic_name, |
eprosima::fastdds::dds::TopicDataType * | data_type, | ||
bool | is_managed = false |
||
) |
Create a Publisher Returns a publisher handle to be used to publish information Takes a string and TopicDataType and creates a topic (see CreateTopic)
- Parameters
-
topic_name The name associated with the topic data_type The topic data type is_managed Whether the SynDDSCommunicator is responsible for using the sending/receiving function calls
◆ CreatePublisher() [2/2]
std::shared_ptr< SynDDSPublisher > chrono::synchrono::SynDDSCommunicator::CreatePublisher | ( | std::shared_ptr< SynDDSTopic > | topic, |
bool | is_managed = false , |
||
eprosima::fastdds::dds::DataWriterQos * | write_qos = nullptr |
||
) |
Create a Publisher Returns a publisher handle to be used to publish information Takes a SynDDSTopic object (see CreateTopic)
- Parameters
-
topic Topic object describing the DDS topic is_managed Whether the SynDDSCommunicator is responsible for using the sending/receiving function calls read_qos Data Writer Quality of Service. Falls back to default if nullptr
◆ CreateSubscriber() [1/2]
std::shared_ptr< SynDDSSubscriber > chrono::synchrono::SynDDSCommunicator::CreateSubscriber | ( | const std::string & | topic_name, |
eprosima::fastdds::dds::TopicDataType * | data_type, | ||
std::function< void(void *)> | callback, | ||
void * | message, | ||
bool | is_synchronous = true , |
||
bool | is_managed = false |
||
) |
Create a subscription to the specified topic Will call the callback when a subscription is received Takes a string and TopicDataType and creates a topic (see CreateTopic)
- Parameters
-
topic_name The name associated with the topic data_type The topic data type callback The callback called when data is received message The message that is used to parse the returned message is_synchronous Whether the subscriber synchronously receives data (default: true) is_managed Whether the SynDDSCommunicator is responsible for using the sending/receiving function calls
◆ CreateSubscriber() [2/2]
std::shared_ptr<SynDDSSubscriber> chrono::synchrono::SynDDSCommunicator::CreateSubscriber | ( | std::shared_ptr< SynDDSTopic > | topic, |
std::function< void(void *)> | callback, | ||
void * | message, | ||
bool | is_synchronous = true , |
||
bool | is_managed = false , |
||
eprosima::fastdds::dds::DataReaderQos * | read_qos = nullptr |
||
) |
Create a subscription to the specified topic Will call the callback when a subscription is received Takes a SynDDSTopic object (see CreateTopic)
- Parameters
-
topic Topic object describing the DDS topic callback The callback called when data is received message The message that is used to parse the returned message is_synchronous Whether the subscriber synchronously receives data (default: true) is_managed Whether the SynDDSCommunicator is responsible for using the sending/receiving function calls read_qos Data Reader Quality of Service. Falls back to default if nullptr
◆ CreateTopic() [1/2]
std::shared_ptr< SynDDSTopic > chrono::synchrono::SynDDSCommunicator::CreateTopic | ( | const std::string & | topic_name, |
eprosima::fastdds::dds::TopicDataType * | data_type | ||
) |
Create a topic using the communicator's prefix The topic will be registered with the participant, if desired.
- Parameters
-
topic_name The name associated with the topic data_type The topic data type
◆ CreateTopic() [2/2]
std::shared_ptr< SynDDSTopic > chrono::synchrono::SynDDSCommunicator::CreateTopic | ( | const std::string & | topic_name, |
eprosima::fastdds::dds::TopicDataType * | data_type, | ||
const std::string & | topic_prefix | ||
) |
Create a topic The topic will be registered with the participant, if desired.
The SynDDSCommunicator maintains a list of topics instantiated in the simulation. A topic can only by constructed through this function or indirectly through the CreateSubscriber/CreatePublisher functions
- Parameters
-
topic_name The name associated with the topic data_type The topic data type topic_prefix The prefix to use
◆ GetMatchedParticipantNames()
std::vector< std::string > chrono::synchrono::SynDDSCommunicator::GetMatchedParticipantNames | ( | ) |
Get the matched participant's names A matched participant is defined as a DDSParticipant Entity that has matched with the participant created on this node.
◆ Initialize()
|
overridevirtual |
Initialization function typically responsible for establishing a connection.
Although not mandatory, this function should handle initial peer-to-peer communication. This could mean a simple handshake or an actual exchange of information used during the simulation.
Reimplemented from chrono::synchrono::SynCommunicator.
◆ Synchronize()
|
overridevirtual |
This function is responsible for continuous synchronization steps This function, depending on it's implementation, could be blocking or non-blocking.
As in, depending on the derived class implementation, this function could use synchronous or asynchronous function calls to implement a communication interface.
Synchronize will serialize the underlying message buffer if it hasn't yet
Implements chrono::synchrono::SynCommunicator.
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono_synchrono/communication/dds/SynDDSCommunicator.h
- /builds/uwsbel/chrono/src/chrono_synchrono/communication/dds/SynDDSCommunicator.cpp