Description
Specialized class for representing a 6-DOF 3D rigid body, with mass matrix and associated variables.
Differently from the generic ChVariablesGeneric, here a full 6x6 mass matrix is not built; rather this object encapsulates a scalar mass and a 3x3 inertia matrix.
#include <ChVariablesBodyOwnMass.h>
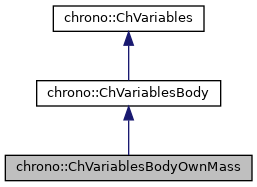
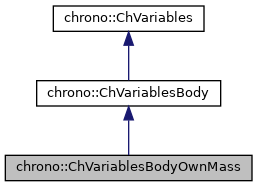
Public Member Functions | |
ChVariablesBodyOwnMass & | operator= (const ChVariablesBodyOwnMass &other) |
Assignment operator: copy from other object. | |
virtual double | GetBodyMass () const override |
Get the mass associated with translation of body. | |
virtual ChMatrix33 & | GetBodyInertia () override |
Access the 3x3 inertia matrix. | |
virtual const ChMatrix33 & | GetBodyInertia () const override |
virtual ChMatrix33 & | GetBodyInvInertia () override |
Access the 3x3 inertia matrix inverted. | |
virtual const ChMatrix33 & | GetBodyInvInertia () const override |
void | SetBodyInertia (const ChMatrix33<> &minertia) |
Set the inertia matrix. | |
void | SetBodyMass (const double mmass) |
Set the mass associated with translation of body. | |
virtual void | ComputeMassInverseTimesVector (ChVectorRef result, ChVectorConstRef vect) const override |
Compute the product of the inverse mass matrix by a given vector and store in result. More... | |
virtual void | AddMassTimesVector (ChVectorRef result, ChVectorConstRef vect) const override |
Compute the product of the mass matrix by a given vector and increment result. More... | |
virtual void | AddMassTimesVectorInto (ChVectorRef result, ChVectorConstRef vect, const double ca) const override |
Add the product of the mass submatrix by a given vector, scaled by ca, to result. More... | |
virtual void | AddMassDiagonalInto (ChVectorRef result, const double ca) const override |
Add the diagonal of the mass matrix, as a vector scaled by ca, to result. More... | |
virtual void | PasteMassInto (ChSparseMatrix &mat, unsigned int start_row, unsigned int start_col, const double ca) const override |
Write the mass submatrix for these variables into the specified global matrix at the offsets of each variable. More... | |
virtual void | ArchiveOut (ChArchiveOut &archive_out) override |
Method to allow serialization of transient data to archives. | |
virtual void | ArchiveIn (ChArchiveIn &archive_in) override |
Method to allow de-serialization of transient data from archives. | |
![]() | |
ChVariablesBody & | operator= (const ChVariablesBody &other) |
Assignment operator: copy from other object. | |
void * | GetUserData () |
void | SetUserData (void *mdata) |
![]() | |
ChVariables (unsigned int dof) | |
ChVariables & | operator= (const ChVariables &other) |
Assignment operator: copy from other object. | |
void | SetDisabled (bool mdis) |
Deactivates/freezes the variable (these variables won't be modified by the system solver). | |
bool | IsDisabled () const |
Check if the variables have been deactivated (these variables won't be modified by the system solver). | |
bool | IsActive () const |
Check if these variables are currently active. More... | |
unsigned int | GetDOF () const |
The number of scalar variables in the vector qb (dof=degrees of freedom). | |
ChVectorRef | State () |
Get a reference to the differential states encapsulated by these variables. More... | |
ChVectorRef | Force () |
Get a reference to the generalized force corresponding to these variables. More... | |
void | SetOffset (unsigned int moff) |
Set offset in the global state vector. More... | |
unsigned int | GetOffset () const |
Get offset in the global state vector. | |
Additional Inherited Members | |
![]() | |
unsigned int | offset |
offset in global q state vector (needed by some solvers) | |
unsigned int | ndof |
number of degrees of freedom (number of contained scalar variables) | |
Member Function Documentation
◆ AddMassDiagonalInto()
|
overridevirtual |
Add the diagonal of the mass matrix, as a vector scaled by ca, to result.
Note: 'result' is a system-level vector of appropriate size. This function must index into this vector using the offsets of each variable.
Implements chrono::ChVariables.
◆ AddMassTimesVector()
|
overridevirtual |
Compute the product of the mass matrix by a given vector and increment result.
This function must perform the operation result += M * vect
for a vector of same size as the variables state.
Implements chrono::ChVariables.
◆ AddMassTimesVectorInto()
|
overridevirtual |
Add the product of the mass submatrix by a given vector, scaled by ca, to result.
Note: 'result' and 'vect' are system-level vectors of appropriate size. This function must index into these vectors using the offsets of each variable.
Implements chrono::ChVariables.
◆ ComputeMassInverseTimesVector()
|
overridevirtual |
Compute the product of the inverse mass matrix by a given vector and store in result.
This function must calculate result = M^(-1) * vect
for a vector of same size as the variables state.
Implements chrono::ChVariables.
◆ PasteMassInto()
|
overridevirtual |
Write the mass submatrix for these variables into the specified global matrix at the offsets of each variable.
The masses will be scaled by the given factor 'ca'. The (start_row, start_col) pair specifies the top-left corner of the system-level mass matrix in the provided matrix. Assembling the system-level sparse matrix is required only if using a direct sparse solver or for debugging/reporting purposes.
Implements chrono::ChVariables.
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono/solver/ChVariablesBodyOwnMass.h
- /builds/uwsbel/chrono/src/chrono/solver/ChVariablesBodyOwnMass.cpp