Tutorial that teaches how to use the POSTPROCESS module to create animations with POVray.
When the simulation is run, a set of .pov and .ini files are saved on disk, so that one can use POVray later to do high-quality rendering of simulations.
Note: the same assets can be used to render animations in real-time in the interactive 3D view of Irrlicht, as explained in demo_IRR_assets.cpp
Example 1
Create a chrono::ChBody, and attach some 'assets' that define 3D shapes. These shapes can be shown by Irrlicht, OpenGL, or POV postprocessing. Note: these assets are independent from collision shapes.
auto floor = chrono_types::make_shared<ChBody>();
floor->SetBodyFixed(true);
auto floor_mat = chrono_types::make_shared<ChMaterialSurfaceNSC>();
floor->GetCollisionModel()->ClearModel();
floor->GetCollisionModel()->AddBox(floor_mat, 10, 0.5, 10, ChVector<>(0, -1, 0));
floor->GetCollisionModel()->BuildModel();
floor->SetCollide(true);
auto boxfloor = chrono_types::make_shared<ChBoxShape>();
boxfloor->GetBoxGeometry().Size = ChVector<>(10, 0.5, 10);
boxfloor->SetColor(ChColor(0.3f, 0.3f, 0.6f));
floor->AddVisualShape(boxfloor, ChFrame<>(ChVector<>(0, -1, 0)));
Example 2
Textures, colors, asset levels with transformations. This section shows how to add more advanced types of assets.
auto body = chrono_types::make_shared<ChBody>();
body->SetBodyFixed(true);
auto sphere = chrono_types::make_shared<ChSphereShape>();
sphere->GetSphereGeometry().rad = 0.5;
body->AddVisualShape(sphere, ChFrame<>(ChVector<>(-1, 0, 0)));
auto mbox = chrono_types::make_shared<ChBoxShape>();
mbox->GetBoxGeometry().Size = ChVector<>(0.2, 0.5, 0.1);
body->AddVisualShape(mbox, ChFrame<>(ChVector<>(1, 0, 0)));
auto cyl = chrono_types::make_shared<ChCylinderShape>();
cyl->GetCylinderGeometry().p1 = ChVector<>(2, -0.2, 0);
cyl->GetCylinderGeometry().p2 = ChVector<>(2.2, 0.5, 0);
cyl->GetCylinderGeometry().rad = 0.3;
body->AddVisualShape(cyl);
auto objmesh = chrono_types::make_shared<ChObjFileShape>();
body->AddVisualShape(objmesh, ChFrame<>(ChVector<>(0, 0, 2)));
for (int j = 0; j < 20; j++) {
auto smallbox = chrono_types::make_shared<ChBoxShape>();
smallbox->GetBoxGeometry().Size = ChVector<>(0.1, 0.1, 0.01);
smallbox->SetColor(ChColor(j * 0.05f, 1 - j * 0.05f, 0.0f));
ChMatrix33<> rot(Q_from_AngY(j * 21 * CH_C_DEG_TO_RAD));
ChVector<> pos = rot * ChVector<>(0.4, 0, 0) + ChVector<>(0, j * 0.02, 0);
body->AddVisualShape(smallbox, ChFrame<>(pos, rot));
}
auto camera = chrono_types::make_shared<ChCamera>();
camera->SetAngle(50);
camera->SetPosition(ChVector<>(-3, 4, -5));
camera->SetAimPoint(ChVector<>(0, 1, 0));
body->AddCamera(camera);
Example 3
Create a chrono::ChParticleCloud cluster, and attach 'assets' that define a single "sample" 3D shape. This will be shown N times in POV or Irrlicht.
auto particles = chrono_types::make_shared<ChParticleCloud>();
auto particle_mat = chrono_types::make_shared<ChMaterialSurfaceNSC>();
particles->GetCollisionModel()->ClearModel();
particles->GetCollisionModel()->AddSphere(particle_mat, 0.05);
particles->GetCollisionModel()->BuildModel();
particles->SetCollide(true);
for (int np = 0; np < 100; ++np)
particles->AddParticle(ChCoordsys<>(ChVector<>(
ChRandom() - 2, 1,
ChRandom() - 0.5)));
auto sphereparticle = chrono_types::make_shared<ChSphereShape>();
sphereparticle->GetSphereGeometry().rad = 0.05;
particles->AddVisualShape(sphereparticle);
The POV exporter
The following part is very important because this is what makes this demo different from the demo_IRR_assets, that used Irrlicht. We need to create a postprocessor of type chrono::postprocess::ChPovRay and tell him that we are going to export our visualization assets:
ChPovRay pov_exporter = ChPovRay(&sys);
pov_exporter.SetLight(ChVector<>(-3, 4, 2), ChColor(0.15f, 0.15f, 0.12f), false);
pov_exporter.SetCustomPOVcommandsScript(
" \
light_source { \
<2, 10, -3> \
color rgb<1.2,1.2,1.2> \
area_light <4, 0, 0>, <0, 0, 4>, 8, 8 \
adaptive 1 \
jitter\
} \
object{ Grid(1,0.02, rgb<0.7,0.8,0.8>, rgbt<1,1,1,1>) rotate <0, 0, 90> } \
");
The simulation loop
Now you have to write the usual while() loop to perform the simulation. Note that before running the loop you need to use pov_exporter.ExportScript(); , and for each timestep you must use pov_exporter.ExportData(); actually this is the instruction that creates the many .dat and .pov files in the output directory.
pov_exporter.ExportScript();
pov_exporter.ExportData();
}
Executing and rendering with POVray
Once you created your program, compile it, then:
- execute the
demo_POST_povray1.exe
- on the console you will see a time counter showing that the system is load and it is being simulated
- when the program ends, you must open POVray and open the
rendering_frames.pov.ini
file, using the Open menu or button, or drag&drop (you can find this .ini file and other POVray as they are saved in the same directory of the executable)
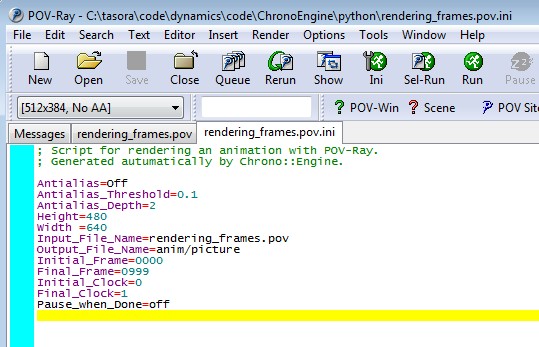
- press the Run button in POVray to execute the .ini file , and you should see that POVray generates lot of frames, being saved in the directory
anim
.
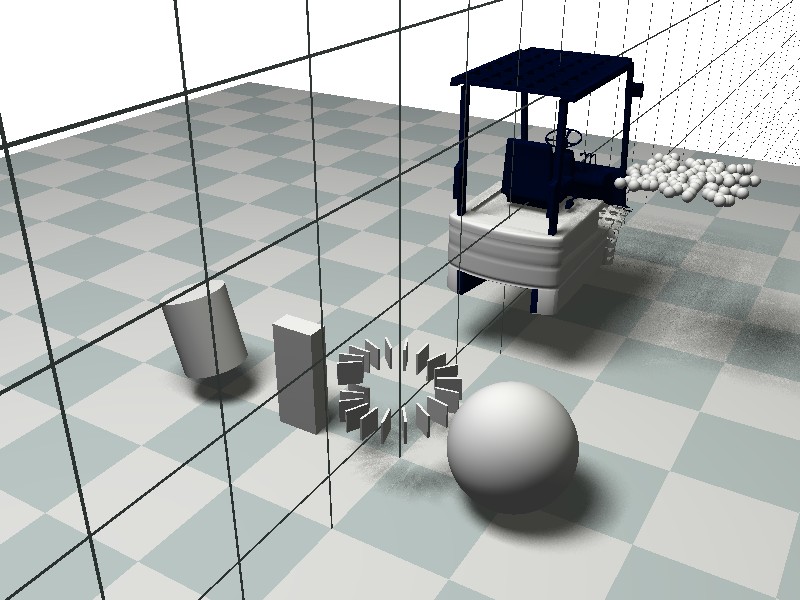
Optional encoding into an AVI or MPEG animation
If you want to generate a .mpeg or .avi animation from the rendered .bmp images, we suggest to use the VirtualDub tool:
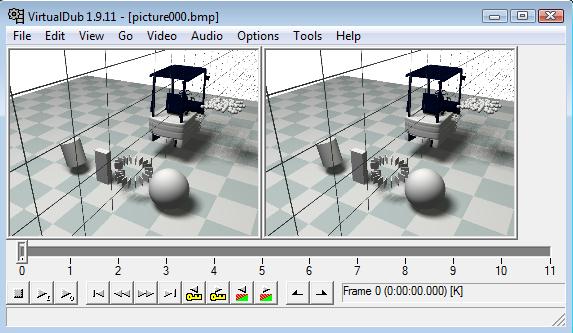
- drag&drop the first .jpg frame in its interface; it will automatically load all other frames in the timeline
- use menu Video/Compression... to setup the proper video codec (suggested: Xvid, DivX, mpeg4, etc.)
- use menu File/Save As Avi... to encode and save the animation on disk.
Listing
In the following we report the entire source code for reference.
#include "chrono/assets/ChBoxShape.h"
#include "chrono/assets/ChCamera.h"
#include "chrono/assets/ChCylinderShape.h"
#include "chrono/assets/ChObjFileShape.h"
#include "chrono/assets/ChSphereShape.h"
#include "chrono/assets/ChTexture.h"
#include "chrono/physics/ChParticleCloud.h"
#include "chrono/physics/ChSystemNSC.h"
#include "chrono_postprocess/ChPovRay.h"
#include "chrono_thirdparty/filesystem/path.h"
using namespace postprocess;
int main(int argc, char* argv[]) {
GetLog() <<
"Copyright (c) 2017 projectchrono.org\nChrono version: " << CHRONO_VERSION <<
"\n\n";
ChPovRay pov_exporter = ChPovRay(&sys);
pov_exporter.SetCustomPOVcommandsScript(
" \
light_source { \
<2, 10, -3> \
color rgb<1.2,1.2,1.2> \
area_light <4, 0, 0>, <0, 0, 4>, 8, 8 \
adaptive 1 \
jitter\
} \
object{ Grid(1,0.02, rgb<0.7,0.8,0.8>, rgbt<1,1,1,1>) rotate <0, 0, 90> } \
");
auto floor = chrono_types::make_shared<ChBody>();
floor->SetBodyFixed(true);
auto floor_mat = chrono_types::make_shared<ChMaterialSurfaceNSC>();
floor->GetCollisionModel()->ClearModel();
floor->GetCollisionModel()->AddBox(floor_mat, 10, 0.5, 10,
ChVector<>(0, -1, 0));
floor->GetCollisionModel()->BuildModel();
floor->SetCollide(true);
auto boxfloor = chrono_types::make_shared<ChBoxShape>();
boxfloor->GetBoxGeometry().Size =
ChVector<>(10, 0.5, 10);
boxfloor->SetColor(
ChColor(0.3f, 0.3f, 0.6f));
auto body = chrono_types::make_shared<ChBody>();
body->SetBodyFixed(true);
auto sphere = chrono_types::make_shared<ChSphereShape>();
sphere->GetSphereGeometry().rad = 0.5;
auto mbox = chrono_types::make_shared<ChBoxShape>();
mbox->GetBoxGeometry().Size =
ChVector<>(0.2, 0.5, 0.1);
auto cyl = chrono_types::make_shared<ChCylinderShape>();
cyl->GetCylinderGeometry().p1 =
ChVector<>(2, -0.2, 0);
cyl->GetCylinderGeometry().p2 =
ChVector<>(2.2, 0.5, 0);
cyl->GetCylinderGeometry().rad = 0.3;
body->AddVisualShape(cyl);
auto objmesh = chrono_types::make_shared<ChObjFileShape>();
for (int j = 0; j < 20; j++) {
auto smallbox = chrono_types::make_shared<ChBoxShape>();
smallbox->GetBoxGeometry().Size =
ChVector<>(0.1, 0.1, 0.01);
smallbox->SetColor(
ChColor(j * 0.05f, 1 - j * 0.05f, 0.0f));
body->AddVisualShape(smallbox,
ChFrame<>(pos, rot));
}
auto camera = chrono_types::make_shared<ChCamera>();
camera->SetAngle(50);
body->AddCamera(camera);
auto particles = chrono_types::make_shared<ChParticleCloud>();
auto particle_mat = chrono_types::make_shared<ChMaterialSurfaceNSC>();
particles->GetCollisionModel()->ClearModel();
particles->GetCollisionModel()->AddSphere(particle_mat, 0.05);
particles->GetCollisionModel()->BuildModel();
particles->SetCollide(true);
for (int np = 0; np < 100; ++np)
auto sphereparticle = chrono_types::make_shared<ChSphereShape>();
sphereparticle->GetSphereGeometry().rad = 0.05;
particles->AddVisualShape(sphereparticle);
pov_exporter.AddAll();
pov_exporter.ExportScript();
pov_exporter.ExportData();
}
return 0;
}